FILE: Bài tập quản lý sinh viên bằng Java Swing + File
Tạo 1 file students.txt chứa thông tin sinh viên. Mỗi sinh viên được lưu trên 1 dòng gồm các thuộc tính : Họ tên, giới tính, rollNo, email, địa chỉ
Thiết kế phần mềm có giao diện như sau
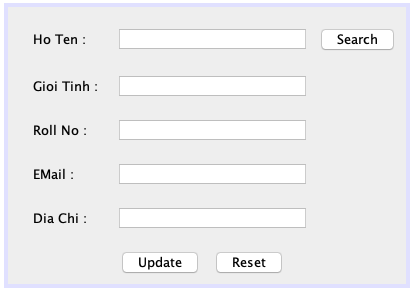
Khi người dùng nhấn vào button search thực hiện task vụ sau
- Nếu RollNo : để trống thì hiển thị message yêu cầu nhập rollno cần tìm kiếm
- Nếu RollNo : khác rỗng -> thực hiện tìm kiếm sinh viên trong file students.txt và hiển thị ra các trường trong form
Khi người dùng click reset thì xoá toàn bộ dữ liệu sv trong form
Khi người dùng click vào update thì cập nhật dữ liệu trong students.txt và xoá tất cả các dữ liệu trong form.
Tags:
Phản hồi từ học viên
5
(Dựa trên đánh giá ngày hôm nay)
![vuong huu phu [T2008A]](https://www.gravatar.com/avatar/307a5cf29780afab49706dc8b15b86c6.jpg?s=80&d=mm&r=g)
vuong huu phu
2021-03-26 06:45:21
package javaapplication49;
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.JOptionPane;
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
/**
*
* @author Admin
*/
public class NewJFrame extends javax.swing.JFrame {
int currentIndex = -1;
ArrayList<Student> stdlist = new ArrayList<>();
/**
* Creates new form NewJFrame
*/
public NewJFrame() {
initComponents();
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
jPanel1 = new javax.swing.JPanel();
Search = new javax.swing.JButton();
jLabel2 = new javax.swing.JLabel();
gender = new javax.swing.JTextField();
jLabel3 = new javax.swing.JLabel();
rollno = new javax.swing.JTextField();
jLabel4 = new javax.swing.JLabel();
email = new javax.swing.JTextField();
jLabel5 = new javax.swing.JLabel();
address = new javax.swing.JTextField();
jLabel6 = new javax.swing.JLabel();
name = new javax.swing.JTextField();
Update = new javax.swing.JButton();
Delete = new javax.swing.JButton();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
Search.setFont(new java.awt.Font("Tahoma", 1, 14)); // NOI18N
Search.setText("Search");
Search.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
SearchActionPerformed(evt);
}
});
jLabel2.setFont(new java.awt.Font("Tahoma", 1, 14)); // NOI18N
jLabel2.setText("Gioi tinh");
gender.setFont(new java.awt.Font("Tahoma", 0, 14)); // NOI18N
jLabel3.setFont(new java.awt.Font("Tahoma", 1, 14)); // NOI18N
jLabel3.setText("Roll No");
rollno.setFont(new java.awt.Font("Tahoma", 0, 14)); // NOI18N
jLabel4.setFont(new java.awt.Font("Tahoma", 1, 14)); // NOI18N
jLabel4.setText("Email");
email.setFont(new java.awt.Font("Tahoma", 0, 14)); // NOI18N
jLabel5.setFont(new java.awt.Font("Tahoma", 1, 14)); // NOI18N
jLabel5.setText("Dia chi");
address.setFont(new java.awt.Font("Tahoma", 0, 14)); // NOI18N
jLabel6.setFont(new java.awt.Font("Tahoma", 1, 14)); // NOI18N
jLabel6.setText("Ho va ten");
name.setFont(new java.awt.Font("Tahoma", 0, 14)); // NOI18N
Update.setFont(new java.awt.Font("Tahoma", 1, 14)); // NOI18N
Update.setText("Update");
Update.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
UpdateActionPerformed(evt);
}
});
Delete.setFont(new java.awt.Font("Tahoma", 1, 14)); // NOI18N
Delete.setText("Delete");
Delete.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
DeleteActionPerformed(evt);
}
});
javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1);
jPanel1.setLayout(jPanel1Layout);
jPanel1Layout.setHorizontalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addGap(23, 23, 23)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(jLabel6, javax.swing.GroupLayout.PREFERRED_SIZE, 100, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(name, javax.swing.GroupLayout.PREFERRED_SIZE, 440, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(jLabel4, javax.swing.GroupLayout.PREFERRED_SIZE, 100, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(email, javax.swing.GroupLayout.PREFERRED_SIZE, 440, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(jLabel3, javax.swing.GroupLayout.PREFERRED_SIZE, 100, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(rollno, javax.swing.GroupLayout.PREFERRED_SIZE, 440, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(jLabel2, javax.swing.GroupLayout.PREFERRED_SIZE, 100, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(gender, javax.swing.GroupLayout.PREFERRED_SIZE, 440, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(jLabel5, javax.swing.GroupLayout.PREFERRED_SIZE, 100, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(address, javax.swing.GroupLayout.PREFERRED_SIZE, 440, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(Update, javax.swing.GroupLayout.PREFERRED_SIZE, 96, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(Delete, javax.swing.GroupLayout.PREFERRED_SIZE, 96, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(216, 216, 216)))))
.addGap(18, 18, 18)
.addComponent(Search, javax.swing.GroupLayout.DEFAULT_SIZE, 96, Short.MAX_VALUE)
.addContainerGap())
);
jPanel1Layout.setVerticalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addGap(62, 62, 62)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(Search, javax.swing.GroupLayout.PREFERRED_SIZE, 33, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(name, javax.swing.GroupLayout.PREFERRED_SIZE, 32, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel6, javax.swing.GroupLayout.PREFERRED_SIZE, 32, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel2, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(gender, javax.swing.GroupLayout.DEFAULT_SIZE, 33, Short.MAX_VALUE))
.addGap(18, 18, 18)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel3, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(rollno, javax.swing.GroupLayout.DEFAULT_SIZE, 30, Short.MAX_VALUE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel4, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(email, javax.swing.GroupLayout.DEFAULT_SIZE, 30, Short.MAX_VALUE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel5, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(address, javax.swing.GroupLayout.DEFAULT_SIZE, 30, Short.MAX_VALUE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(Update, javax.swing.GroupLayout.PREFERRED_SIZE, 33, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(Delete, javax.swing.GroupLayout.PREFERRED_SIZE, 33, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(61, 61, 61))
);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jPanel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(jPanel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(0, 3, Short.MAX_VALUE))
);
pack();
}// </editor-fold>
private void SearchActionPerformed(java.awt.event.ActionEvent evt) {
if (rollno.getText().equals("")) {
JOptionPane.showMessageDialog(this, "Nhap Rollno !");
} else {
FileInputStream fis = null;
InputStreamReader rd = null;
BufferedReader bfr = null;
try {
fis = new FileInputStream("student.txt");
rd = new InputStreamReader(fis, StandardCharsets.UTF_8);
bfr = new BufferedReader(rd);
String line;
try {
while ((line = bfr.readLine()) != null) {
Student std = new Student();
std.cu_phap(line);
stdlist.add(std);
}
} catch (IOException ex) {
Logger.getLogger(NewJFrame.class.getName()).log(Level.SEVERE, null, ex);
}
} catch (FileNotFoundException ex) {
Logger.getLogger(NewJFrame.class.getName()).log(Level.SEVERE, null, ex);
} finally {
if (fis != null) {
try {
fis.close();
} catch (IOException ex) {
Logger.getLogger(NewJFrame.class.getName()).log(Level.SEVERE, null, ex);
}
}
if (rd != null) {
try {
rd.close();
} catch (IOException ex) {
Logger.getLogger(NewJFrame.class.getName()).log(Level.SEVERE, null, ex);
}
}
if (bfr != null) {
try {
bfr.close();
} catch (IOException ex) {
Logger.getLogger(NewJFrame.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
for (Student student : stdlist) {
System.out.println(student.getRollno() + " - " + rollno.getText().trim());
if (student.getRollno().trim().equalsIgnoreCase(rollno.getText().trim())) {
System.out.println("Thanh cong");
name.setText(student.getHo_va_ten());
gender.setText(student.getGioi_tinh());
rollno.setText(student.getRollno());
email.setText(student.getEmail());
address.setText(student.getDia_chi());
break;
}
}
}
// for (Student s : stdlist) {
// if (rollno.getText().equalsIgnoreCase(s.getRollno())) {
// i++;
// name.setText(s.getHo_va_ten());
// gender.setText(s.getGioi_tinh());
// rollno.setText(s.getRollno());
// email.setText(s.getEmail());
// address.setText(s.getDia_chi());
// currentIndex = i;
// }
// }
}
private void UpdateActionPerformed(java.awt.event.ActionEvent evt) {
int t = JOptionPane.showConfirmDialog(rootPane, "Ban co muon luu sinh vien nay khong");
Student std = new Student();
if (t == 0) {
std.setHo_va_ten(name.getText());
std.setGioi_tinh(gender.getText());
std.setRollno(rollno.getText());
std.setEmail(email.getText());
std.setDia_chi(address.getText());
stdlist.add(std);
FileOutputStream fos = null;
try {
fos = new FileOutputStream("student.txt");
for (Student s : stdlist) {
String line = s.getline();
byte[] b = line.getBytes("utf8");
fos.write(b);
}
} catch (IOException ex) {
Logger.getLogger(NewJFrame.class.getName()).log(Level.SEVERE, null, ex);
} finally {
if (fos != null) {
try {
fos.close();
} catch (IOException ex) {
Logger.getLogger(NewJFrame.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
JOptionPane.showMessageDialog(rootPane, "Da luu xong !");
name.setText("");
gender.setText("");
rollno.setText("");
email.setText("");
address.setText("");
} else {
name.setText("");
gender.setText("");
rollno.setText("");
email.setText("");
address.setText("");
}
}
private void DeleteActionPerformed(java.awt.event.ActionEvent evt) {
name.setText("");
gender.setText("");
rollno.setText("");
email.setText("");
address.setText("");
}
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(NewJFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(NewJFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(NewJFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(NewJFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new NewJFrame().setVisible(true);
}
});
}
// Variables declaration - do not modify
private javax.swing.JButton Delete;
private javax.swing.JButton Search;
private javax.swing.JButton Update;
private javax.swing.JTextField address;
private javax.swing.JTextField email;
private javax.swing.JTextField gender;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel4;
private javax.swing.JLabel jLabel5;
private javax.swing.JLabel jLabel6;
private javax.swing.JPanel jPanel1;
private javax.swing.JTextField name;
private javax.swing.JTextField rollno;
// End of variables declaration
}
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package javaapplication49;
/**
*
* @author Admin
*/
public class Student {
String Ho_va_ten, Gioi_tinh, Rollno, Email, Dia_chi;
public Student() {
}
public Student(String Ho_va_ten, String Gioi_tinh, String Rollno, String Email, String Dia_chi) {
this.Ho_va_ten = Ho_va_ten;
this.Gioi_tinh = Gioi_tinh;
this.Rollno = Rollno;
this.Email = Email;
this.Dia_chi = Dia_chi;
}
public String getHo_va_ten() {
return Ho_va_ten;
}
public void setHo_va_ten(String Ho_va_ten) {
this.Ho_va_ten = Ho_va_ten;
}
public String getGioi_tinh() {
return Gioi_tinh;
}
public void setGioi_tinh(String Gioi_tinh) {
this.Gioi_tinh = Gioi_tinh;
}
public String getRollno() {
return Rollno;
}
public void setRollno(String Rollno) {
this.Rollno = Rollno;
}
public String getEmail() {
return Email;
}
public void setEmail(String Email) {
this.Email = Email;
}
public String getDia_chi() {
return Dia_chi;
}
public void setDia_chi(String Dia_chi) {
this.Dia_chi = Dia_chi;
}
@Override
public String toString() {
return "Ho_va_ten=" + Ho_va_ten + ", Gioi_tinh=" + Gioi_tinh + ", Rollno=" + Rollno + ", Email=" + Email + ", Dia_chi=" + Dia_chi;
}
public String getline() {
return Ho_va_ten + "," + Gioi_tinh + "," + Rollno + "," + Email + "," + Dia_chi + "\n";
}
public void cu_phap(String line) {
String[] str = line.split(",");
Ho_va_ten = str[0];
Gioi_tinh = str[1];
Rollno = str[2];
Email = str[3];
Dia_chi = str[4];
}
}
![Nguyễn Tiến Đạt [T2008A]](https://www.gravatar.com/avatar/b5819cd0adc95c727c7ad0c2bcf6098b.jpg?s=80&d=mm&r=g)
Nguyễn Tiến Đạt
2021-03-25 04:47:20
#Student.java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package Java2.lesson6.QuanLySinhVien;
/**
*
* @author MyPC
*/
public class Student {
String name, gender, rollNo, email, address;
public Student() {
}
public Student(String name, String gender, String rollNo, String email, String address) {
this.name = name;
this.gender = gender;
this.rollNo = rollNo;
this.email = email;
this.address = address;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
public String getRollNo() {
return rollNo;
}
public void setRollNo(String rollNo) {
this.rollNo = rollNo;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getLine(){
return name + "," + gender + "," + rollNo + "," + email + "," + address + "\n";
}
}
#StudentFrame.form
<?xml version="1.0" encoding="UTF-8" ?>
<Form version="1.3" maxVersion="1.9" type="org.netbeans.modules.form.forminfo.JFrameFormInfo">
<Properties>
<Property name="defaultCloseOperation" type="int" value="3"/>
</Properties>
<SyntheticProperties>
<SyntheticProperty name="formSizePolicy" type="int" value="1"/>
<SyntheticProperty name="generateCenter" type="boolean" value="false"/>
</SyntheticProperties>
<AuxValues>
<AuxValue name="FormSettings_autoResourcing" type="java.lang.Integer" value="0"/>
<AuxValue name="FormSettings_autoSetComponentName" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_generateFQN" type="java.lang.Boolean" value="true"/>
<AuxValue name="FormSettings_generateMnemonicsCode" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_i18nAutoMode" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_layoutCodeTarget" type="java.lang.Integer" value="1"/>
<AuxValue name="FormSettings_listenerGenerationStyle" type="java.lang.Integer" value="0"/>
<AuxValue name="FormSettings_variablesLocal" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_variablesModifier" type="java.lang.Integer" value="2"/>
</AuxValues>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<EmptySpace min="36" pref="36" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel3" min="-2" pref="73" max="-2" attributes="0"/>
<Component id="jLabel4" min="-2" pref="73" max="-2" attributes="0"/>
<Component id="jLabel5" min="-2" pref="73" max="-2" attributes="0"/>
<Component id="jLabel6" min="-2" pref="73" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="29" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="txtRollNo" min="-2" pref="308" max="-2" attributes="0"/>
<Component id="txtGender" min="-2" pref="308" max="-2" attributes="0"/>
<Component id="txtEmail" alignment="0" min="-2" pref="308" max="-2" attributes="0"/>
<Component id="txtAddress" alignment="0" min="-2" pref="308" max="-2" attributes="0"/>
</Group>
<EmptySpace max="32767" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Component id="jLabel2" min="-2" pref="73" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="29" max="-2" attributes="0"/>
<Component id="txtName" min="-2" pref="308" max="-2" attributes="0"/>
<EmptySpace pref="43" max="32767" attributes="0"/>
<Component id="btnSearch" min="-2" pref="73" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="33" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
<Group type="102" alignment="1" attributes="0">
<EmptySpace max="32767" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="1" attributes="0">
<Component id="jLabel1" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="187" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="1" attributes="0">
<Component id="btnUpdate" min="-2" pref="66" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="44" max="-2" attributes="0"/>
<Component id="btnReset" min="-2" pref="68" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="222" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<EmptySpace min="-2" pref="48" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel2" alignment="3" min="-2" pref="33" max="-2" attributes="0"/>
<Component id="txtName" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<Group type="102" attributes="0">
<EmptySpace min="-2" pref="57" max="-2" attributes="0"/>
<Component id="btnSearch" min="-2" pref="38" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="txtGender" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="jLabel3" alignment="3" min="-2" pref="33" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="txtRollNo" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="jLabel4" alignment="3" min="-2" pref="33" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="30" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="txtEmail" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="jLabel5" alignment="3" min="-2" pref="33" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="28" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="txtAddress" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="jLabel6" alignment="3" min="-2" pref="33" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="35" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="btnUpdate" pref="34" max="32767" attributes="0"/>
<Component id="btnReset" max="32767" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="44" max="-2" attributes="0"/>
<Component id="jLabel1" min="-2" max="-2" attributes="0"/>
<EmptySpace pref="60" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JLabel" name="jLabel1">
</Component>
<Component class="javax.swing.JLabel" name="jLabel2">
<Properties>
<Property name="text" type="java.lang.String" value="Ho Ten:"/>
</Properties>
</Component>
<Component class="javax.swing.JTextField" name="txtName">
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="txtNameActionPerformed"/>
</Events>
</Component>
<Component class="javax.swing.JLabel" name="jLabel3">
<Properties>
<Property name="text" type="java.lang.String" value="Gioi tinh:"/>
</Properties>
</Component>
<Component class="javax.swing.JTextField" name="txtGender">
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="txtGenderActionPerformed"/>
</Events>
</Component>
<Component class="javax.swing.JLabel" name="jLabel4">
<Properties>
<Property name="text" type="java.lang.String" value="Roll No:"/>
</Properties>
</Component>
<Component class="javax.swing.JTextField" name="txtRollNo">
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="txtRollNoActionPerformed"/>
</Events>
</Component>
<Component class="javax.swing.JTextField" name="txtEmail">
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="txtEmailActionPerformed"/>
</Events>
</Component>
<Component class="javax.swing.JLabel" name="jLabel5">
<Properties>
<Property name="text" type="java.lang.String" value="Email:"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel6">
<Properties>
<Property name="text" type="java.lang.String" value="Dia chi:"/>
</Properties>
</Component>
<Component class="javax.swing.JTextField" name="txtAddress">
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="txtAddressActionPerformed"/>
</Events>
</Component>
<Component class="java.awt.Button" name="btnSearch">
<Properties>
<Property name="label" type="java.lang.String" value="Search"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="btnSearchActionPerformed"/>
</Events>
</Component>
<Component class="java.awt.Button" name="btnReset">
<Properties>
<Property name="label" type="java.lang.String" value="Reset"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="btnResetActionPerformed"/>
</Events>
</Component>
<Component class="java.awt.Button" name="btnUpdate">
<Properties>
<Property name="label" type="java.lang.String" value="Update"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="btnUpdateActionPerformed"/>
</Events>
</Component>
</SubComponents>
</Form>
#StudentFrame.java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package Java2.lesson6.QuanLySinhVien;
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.List;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.JOptionPane;
/**
*
* @author MyPC
*/
public class StudentFrame extends javax.swing.JFrame {
List<Student> studentList = new ArrayList<>();
int currentIndex = -1;
/**
* Creates new form StudentFrame
*/
public StudentFrame() {
initComponents();
FileInputStream fis = null;
InputStreamReader reader = null;
BufferedReader bReader = null;
try {
fis = new FileInputStream("students.txt");
reader = new InputStreamReader(fis);
bReader = new BufferedReader(reader);
String line;
while((line = bReader.readLine()) != null){
String[] array = line.split(",");
Student std = new Student();
std.name = array[0];
std.gender = array[1];
std.rollNo = array[2];
std.email = array[3];
std.address = array[4];
studentList.add(std);
}
} catch (FileNotFoundException ex) {
Logger.getLogger(StudentFrame.class.getName()).log(Level.SEVERE, null, ex);
} catch (IOException ex) {
Logger.getLogger(StudentFrame.class.getName()).log(Level.SEVERE, null, ex);
}finally{
if(fis != null){
try {
fis.close();
} catch (IOException ex) {
Logger.getLogger(StudentFrame.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
jLabel1 = new javax.swing.JLabel();
jLabel2 = new javax.swing.JLabel();
txtName = new javax.swing.JTextField();
jLabel3 = new javax.swing.JLabel();
txtGender = new javax.swing.JTextField();
jLabel4 = new javax.swing.JLabel();
txtRollNo = new javax.swing.JTextField();
txtEmail = new javax.swing.JTextField();
jLabel5 = new javax.swing.JLabel();
jLabel6 = new javax.swing.JLabel();
txtAddress = new javax.swing.JTextField();
btnSearch = new java.awt.Button();
btnReset = new java.awt.Button();
btnUpdate = new java.awt.Button();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jLabel2.setText("Ho Ten:");
txtName.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
txtNameActionPerformed(evt);
}
});
jLabel3.setText("Gioi tinh:");
txtGender.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
txtGenderActionPerformed(evt);
}
});
jLabel4.setText("Roll No:");
txtRollNo.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
txtRollNoActionPerformed(evt);
}
});
txtEmail.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
txtEmailActionPerformed(evt);
}
});
jLabel5.setText("Email:");
jLabel6.setText("Dia chi:");
txtAddress.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
txtAddressActionPerformed(evt);
}
});
btnSearch.setLabel("Search");
btnSearch.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnSearchActionPerformed(evt);
}
});
btnReset.setLabel("Reset");
btnReset.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnResetActionPerformed(evt);
}
});
btnUpdate.setLabel("Update");
btnUpdate.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnUpdateActionPerformed(evt);
}
});
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(36, 36, 36)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel3, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel4, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel5, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel6, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(29, 29, 29)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(txtRollNo, javax.swing.GroupLayout.PREFERRED_SIZE, 308, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(txtGender, javax.swing.GroupLayout.PREFERRED_SIZE, 308, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(txtEmail, javax.swing.GroupLayout.PREFERRED_SIZE, 308, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(txtAddress, javax.swing.GroupLayout.PREFERRED_SIZE, 308, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel2, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(29, 29, 29)
.addComponent(txtName, javax.swing.GroupLayout.PREFERRED_SIZE, 308, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 43, Short.MAX_VALUE)
.addComponent(btnSearch, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(33, 33, 33))))
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addComponent(jLabel1)
.addGap(187, 187, 187))
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addComponent(btnUpdate, javax.swing.GroupLayout.PREFERRED_SIZE, 66, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(44, 44, 44)
.addComponent(btnReset, javax.swing.GroupLayout.PREFERRED_SIZE, 68, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(222, 222, 222))))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(48, 48, 48)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel2, javax.swing.GroupLayout.PREFERRED_SIZE, 33, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(txtName, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addGroup(layout.createSequentialGroup()
.addGap(57, 57, 57)
.addComponent(btnSearch, javax.swing.GroupLayout.PREFERRED_SIZE, 38, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(txtGender, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel3, javax.swing.GroupLayout.PREFERRED_SIZE, 33, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(25, 25, 25)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(txtRollNo, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel4, javax.swing.GroupLayout.PREFERRED_SIZE, 33, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(30, 30, 30)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(txtEmail, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel5, javax.swing.GroupLayout.PREFERRED_SIZE, 33, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(28, 28, 28)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(txtAddress, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel6, javax.swing.GroupLayout.PREFERRED_SIZE, 33, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(35, 35, 35)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(btnUpdate, javax.swing.GroupLayout.DEFAULT_SIZE, 34, Short.MAX_VALUE)
.addComponent(btnReset, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addGap(44, 44, 44)
.addComponent(jLabel1)
.addContainerGap(60, Short.MAX_VALUE))
);
pack();
}// </editor-fold>//GEN-END:initComponents
private void txtNameActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_txtNameActionPerformed
// TODO add your handling code here:
}//GEN-LAST:event_txtNameActionPerformed
private void txtGenderActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_txtGenderActionPerformed
// TODO add your handling code here:
}//GEN-LAST:event_txtGenderActionPerformed
private void txtRollNoActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_txtRollNoActionPerformed
// TODO add your handling code here:
}//GEN-LAST:event_txtRollNoActionPerformed
private void txtEmailActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_txtEmailActionPerformed
// TODO add your handling code here:
}//GEN-LAST:event_txtEmailActionPerformed
private void txtAddressActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_txtAddressActionPerformed
// TODO add your handling code here:
}//GEN-LAST:event_txtAddressActionPerformed
private void btnSearchActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnSearchActionPerformed
// TODO add your handling code here:
if("".equals(txtRollNo.getText())){
JOptionPane.showMessageDialog(rootPane, "Nhap rollNo de tim kiem");
return;
}
int check = 0;
int index = -1;
for (Student student : studentList) {
index++;
if(txtRollNo.getText().equalsIgnoreCase(student.getRollNo())){
txtName.setText(student.getName());
txtGender.setText(student.getGender());
txtRollNo.setText(student.getRollNo());
txtEmail.setText(student.getEmail());
txtAddress.setText(student.getAddress());
currentIndex = index;
check++;
break;
}
}
if(check == 0){
JOptionPane.showMessageDialog(rootPane, "Khong tim thay sinh vien nao");
btnResetActionPerformed(evt);
}
}//GEN-LAST:event_btnSearchActionPerformed
private void btnResetActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnResetActionPerformed
// TODO add your handling code here:
txtName.setText("");
txtGender.setText("");
txtRollNo.setText("");
txtEmail.setText("");
txtAddress.setText("");
}//GEN-LAST:event_btnResetActionPerformed
private void btnUpdateActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnUpdateActionPerformed
// TODO add your handling code here:
if(currentIndex == - 1){
JOptionPane.showMessageDialog(rootPane, "Lua chon sinh vien can update");
}else{
studentList.get(currentIndex).name = txtName.getText();
studentList.get(currentIndex).gender = txtGender.getText();
studentList.get(currentIndex).rollNo = txtRollNo.getText();
studentList.get(currentIndex).email = txtEmail.getText();
studentList.get(currentIndex).address = txtAddress.getText();
FileOutputStream fos = null;
try {
fos = new FileOutputStream("students.txt");
for (Student student : studentList) {
byte[] b = student.getLine().getBytes();
fos.write(b);
}
} catch (FileNotFoundException ex) {
Logger.getLogger(StudentFrame.class.getName()).log(Level.SEVERE, null, ex);
} catch (IOException ex) {
Logger.getLogger(StudentFrame.class.getName()).log(Level.SEVERE, null, ex);
}finally{
if(fos != null){
try {
fos.close();
} catch (IOException ex) {
Logger.getLogger(StudentFrame.class.getName()).log(Level.SEVERE, null, ex);
}
}
JOptionPane.showMessageDialog(rootPane, "Update thanh cong!!");
currentIndex = -1;
btnResetActionPerformed(evt);
}
}
}//GEN-LAST:event_btnUpdateActionPerformed
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(StudentFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(StudentFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(StudentFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(StudentFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new StudentFrame().setVisible(true);
}
});
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private java.awt.Button btnReset;
private java.awt.Button btnSearch;
private java.awt.Button btnUpdate;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel4;
private javax.swing.JLabel jLabel5;
private javax.swing.JLabel jLabel6;
private javax.swing.JTextField txtAddress;
private javax.swing.JTextField txtEmail;
private javax.swing.JTextField txtGender;
private javax.swing.JTextField txtName;
private javax.swing.JTextField txtRollNo;
// End of variables declaration//GEN-END:variables
}
![NguyenHuuThanh [T1907A]](https://www.gravatar.com/avatar/035e4f4fed661b8e1c3e066e43cd5e41.jpg?s=80&d=mm&r=g)
NguyenHuuThanh
2020-04-06 00:21:58
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package javaswingfile;
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.util.ArrayList;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.JOptionPane;
/**
*
* @author abc
*/
public class jframe extends javax.swing.JFrame {
ArrayList<Student> studentlist = new ArrayList<>();
/**
* Creates new form jframe
*/
public jframe() {
initComponents();
setLocationRelativeTo(this);
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
jLabel1 = new javax.swing.JLabel();
jLabel2 = new javax.swing.JLabel();
jLabel3 = new javax.swing.JLabel();
jLabel4 = new javax.swing.JLabel();
jLabel5 = new javax.swing.JLabel();
txtFullname = new javax.swing.JTextField();
txtGender = new javax.swing.JTextField();
txtRollno = new javax.swing.JTextField();
txtEmail = new javax.swing.JTextField();
txtAddress = new javax.swing.JTextField();
Search = new javax.swing.JButton();
Update = new javax.swing.JButton();
Reset = new javax.swing.JButton();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jLabel1.setText("Ho Ten :");
jLabel2.setText("Gioi tinh :");
jLabel3.setText("Roll No :");
jLabel4.setText("Email :");
jLabel5.setText("Dia chi :");
txtFullname.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
txtFullnameActionPerformed(evt);
}
});
Search.setText("Search");
Search.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
SearchActionPerformed(evt);
}
});
Update.setText("Update");
Update.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
UpdateActionPerformed(evt);
}
});
Reset.setText("Reset");
Reset.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
ResetActionPerformed(evt);
}
});
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(23, 23, 23)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel1)
.addComponent(jLabel2)
.addComponent(jLabel3)
.addComponent(jLabel4)
.addComponent(jLabel5))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(txtAddress, javax.swing.GroupLayout.DEFAULT_SIZE, 153, Short.MAX_VALUE)
.addComponent(txtEmail)
.addComponent(txtRollno)
.addComponent(txtGender)
.addComponent(txtFullname))
.addGap(36, 36, 36)
.addComponent(Search)
.addGap(28, 28, 28))
.addGroup(layout.createSequentialGroup()
.addGap(63, 63, 63)
.addComponent(Update)
.addGap(77, 77, 77)
.addComponent(Reset)
.addContainerGap(132, Short.MAX_VALUE))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel1)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(txtFullname, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(Search)))
.addGap(30, 30, 30)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel2)
.addComponent(txtGender, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(37, 37, 37)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel3)
.addComponent(txtRollno, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(40, 40, 40)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel4)
.addComponent(txtEmail, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(23, 23, 23)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel5)
.addComponent(txtAddress, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(Update)
.addComponent(Reset))
.addContainerGap(15, Short.MAX_VALUE))
);
pack();
}// </editor-fold>
private void txtFullnameActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
}
private void ResetActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
// reset
System.out.println("Reset");
txtFullname.setText("");
txtGender.setText("");
txtRollno.setText("");
txtEmail.setText("");
txtAddress.setText("");
}
private void UpdateActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
// Update
Student student = new Student();
student.setName(txtFullname.getText());
student.setGender(txtGender.getText());
student.setAddress(txtAddress.getText());
student.setRollno(txtRollno.getText());
student.setEmail(txtEmail.getText());
studentlist.add(student);
FileOutputStream fos = null;
BufferedOutputStream bos = null;
try {
fos = new FileOutputStream("student.txt");
bos = new BufferedOutputStream(fos);
for (Student student1 : studentlist)
{
String line = student1.getLine();
System.out.println("Line: " + line);
byte[] b = line.getBytes("utf8");
bos.write(b);
}
} catch (FileNotFoundException ex) {
Logger.getLogger(jframe.class.getName()).log(Level.SEVERE, null, ex);
} catch (UnsupportedEncodingException ex) {
Logger.getLogger(jframe.class.getName()).log(Level.SEVERE, null, ex);
} catch (IOException ex) {
Logger.getLogger(jframe.class.getName()).log(Level.SEVERE, null, ex);
}finally {
if(fos != null)
{
try {
fos.close();
} catch (IOException ex) {
Logger.getLogger(jframe.class.getName()).log(Level.SEVERE, null, ex);
}
}
if(bos != null)
{
try {
bos.close();
} catch (IOException ex) {
Logger.getLogger(jframe.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
System.out.println("Thanh cong");
System.out.println("Reset");
txtFullname.setText("");
txtGender.setText("");
txtRollno.setText("");
txtEmail.setText("");
txtAddress.setText("");
}
private void SearchActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
// Search
studentlist.clear();
boolean check = true;
int x = -1;
if (txtRollno.getText().equals(""))
{
JOptionPane.showMessageDialog(rootPane, "Nhap roll no"); // pop up
check = false;
}
else
{
try {
readFile();
} catch (IOException ex) {
Logger.getLogger(jframe.class.getName()).log(Level.SEVERE, null, ex);
}
for ( int i = 0 ; i< studentlist.size() ; i++)
{
if(studentlist.get(i).getRollno().equalsIgnoreCase(txtRollno.getText()))
{
check = true;
x = i;
break;
}
}
}
if ( check = true )
{
txtFullname.setText(studentlist.get(x).getName());
txtGender.setText(studentlist.get(x).getGender());
txtRollno.setText(studentlist.get(x).getRollno());
txtEmail.setText(studentlist.get(x).getEmail());
txtAddress.setText(studentlist.get(x).getAddress());
}
else
{
JOptionPane.showMessageDialog(rootPane,txtRollno.getText() + "Nhap roll no");
}
}
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(jframe.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(jframe.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(jframe.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(jframe.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new jframe().setVisible(true);
}
});
}
private void readFile() throws IOException
{
FileReader fr = null;
BufferedReader br = null;
try {
fr = new FileReader("student.txt");
br = new BufferedReader(fr);
// doc file
String line = null;
while((line = br.readLine())!= null)
{
Student student = new Student();
student.parseLine(line);
studentlist.add(student);
}
} catch (FileNotFoundException ex) {
Logger.getLogger(jframe.class.getName()).log(Level.SEVERE, null, ex);
}
}
// Variables declaration - do not modify
private javax.swing.JButton Reset;
private javax.swing.JButton Search;
private javax.swing.JButton Update;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel4;
private javax.swing.JLabel jLabel5;
private javax.swing.JTextField txtAddress;
private javax.swing.JTextField txtEmail;
private javax.swing.JTextField txtFullname;
private javax.swing.JTextField txtGender;
private javax.swing.JTextField txtRollno;
// End of variables declaration
}
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package javaswingfile;
/**
*
* @author abc
*/
public class Student {
String name , gender , rollno, email, address;
public Student()
{
}
public Student(String name, String gender, String rollno, String email, String address) {
this.name = name;
this.gender = gender;
this.rollno = rollno;
this.email = email;
this.address = address;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
public String getRollno() {
return rollno;
}
public void setRollno(String rollno) {
this.rollno = rollno;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getLine()
{
return name + "," + gender + "," + rollno + "," + email + "," + address;
}
public void parseLine(String line)
{
line = line.trim();
String[] param = line.split(",");
if (param.length < 5)
{
System.out.println("Error");
}
this.name = param[0];
this.gender = param[1];
this.rollno = param[2];
this.email = param[3];
this.address = param[4];
}
}
![nguyễn văn huy [T1907A]](https://www.gravatar.com/avatar/b107d14d7d43c142b68c12c377262371.jpg?s=80&d=mm&r=g)
nguyễn văn huy
2020-04-05 07:38:11
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package StudentSwing;
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.util.ArrayList;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.JOptionPane;
import javax.swing.table.DefaultTableModel;
/**
*
* @author ASUS
*/
public class Swing extends javax.swing.JFrame {
ArrayList<Student> thaolist;
DefaultTableModel table;
private Object address;
private Object email;
private Object rollno;
private Object sex;
/**
* Creates new form Swing
*/
public Swing() {
initComponents();
thaolist=new ArrayList<>();
setLocationRelativeTo(this);
table=(DefaultTableModel) bltStudent.getModel();
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
jPanel1 = new javax.swing.JPanel();
jLabel1 = new javax.swing.JLabel();
jLabel2 = new javax.swing.JLabel();
jLabel3 = new javax.swing.JLabel();
jLabel4 = new javax.swing.JLabel();
jLabel5 = new javax.swing.JLabel();
txtName = new javax.swing.JTextField();
txtSex = new javax.swing.JTextField();
txtRollno = new javax.swing.JTextField();
txtEmail = new javax.swing.JTextField();
txtAddress = new javax.swing.JTextField();
btnSearch = new javax.swing.JButton();
btnUpdate = new javax.swing.JButton();
btnReset = new javax.swing.JButton();
jScrollPane1 = new javax.swing.JScrollPane();
bltStudent = new javax.swing.JTable();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jPanel1.setBackground(new java.awt.Color(153, 255, 255));
jPanel1.setBorder(javax.swing.BorderFactory.createTitledBorder("Students"));
jLabel1.setText("Name");
jLabel2.setText("Sex");
jLabel3.setText("Rollno");
jLabel4.setText("Email");
jLabel5.setText("Address");
btnSearch.setText("Search");
btnSearch.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnSearchActionPerformed(evt);
}
});
btnUpdate.setText("Update");
btnUpdate.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnUpdateActionPerformed(evt);
}
});
btnReset.setText("Reset");
btnReset.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnResetActionPerformed(evt);
}
});
bltStudent.setModel(new javax.swing.table.DefaultTableModel(
new Object [][] {
},
new String [] {
"Name", "Sex", "RolloNo", "Email", "Address"
}
));
jScrollPane1.setViewportView(bltStudent);
javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1);
jPanel1.setLayout(jPanel1Layout);
jPanel1Layout.setHorizontalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 61, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(168, 168, 168)
.addComponent(txtName, javax.swing.GroupLayout.PREFERRED_SIZE, 426, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(jPanel1Layout.createSequentialGroup()
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jLabel4, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jLabel5, javax.swing.GroupLayout.DEFAULT_SIZE, 61, Short.MAX_VALUE))
.addGap(168, 168, 168)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(btnUpdate, javax.swing.GroupLayout.PREFERRED_SIZE, 119, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 176, Short.MAX_VALUE)
.addComponent(btnReset, javax.swing.GroupLayout.PREFERRED_SIZE, 131, javax.swing.GroupLayout.PREFERRED_SIZE))
.addComponent(txtEmail)
.addComponent(txtAddress)))
.addGroup(jPanel1Layout.createSequentialGroup()
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING, false)
.addComponent(jLabel3, javax.swing.GroupLayout.DEFAULT_SIZE, 61, Short.MAX_VALUE)
.addComponent(jLabel2, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(txtSex, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.PREFERRED_SIZE, 426, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(txtRollno, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.PREFERRED_SIZE, 426, javax.swing.GroupLayout.PREFERRED_SIZE))))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 193, Short.MAX_VALUE)
.addComponent(btnSearch)
.addGap(25, 25, 25))
.addComponent(jScrollPane1, javax.swing.GroupLayout.Alignment.TRAILING)
);
jPanel1Layout.setVerticalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addGap(24, 24, 24)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel1)
.addComponent(txtName, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(btnSearch))
.addGap(27, 27, 27)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel2)
.addComponent(txtSex, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(37, 37, 37)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel3)
.addComponent(txtRollno, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addGap(40, 40, 40)
.addComponent(jLabel4))
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, jPanel1Layout.createSequentialGroup()
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(txtEmail, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addGap(33, 33, 33)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(jLabel5)
.addComponent(txtAddress, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(64, 64, 64)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(btnUpdate)
.addComponent(btnReset))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 50, Short.MAX_VALUE)
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jPanel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(jPanel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(0, 263, Short.MAX_VALUE))
);
pack();
}// </editor-fold>
private void btnResetActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
txtAddress.setText("");
txtEmail.setText("");
txtName.setText("");
txtRollno.setText("");
txtSex.setText("");
}
private void btnUpdateActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
Student thaoh=new Student();
thaoh.address(txtAddress.getText());
thaoh.email(txtEmail.getText());
thaoh.name(txtName.getText());
thaoh.rollno(txtRollno.getText());
thaoh.sex(txtSex.getText());
thaolist.add(thaoh);
FileOutputStream fis=null;
BufferedOutputStream fos=null;
try {
String filename="E:\\BT1907A\\btjava\\swing\\src\\swing01\\student.txt";
fis=new FileOutputStream(filename);
fos=new BufferedOutputStream(fos);
for (Student student : thaolist) {
String line=student.getLine();
System.out.println(">>>>>"+line);
byte[] b=line.getBytes("utf8");
try {
fos.write(b);
} catch (IOException ex) {
Logger.getLogger(Swing.class.getName()).log(Level.SEVERE, null, ex);
}
}
} catch (FileNotFoundException ex) {
Logger.getLogger(Swing.class.getName()).log(Level.SEVERE, null, ex);
} catch (UnsupportedEncodingException ex) {
Logger.getLogger(Swing.class.getName()).log(Level.SEVERE, null, ex);
}finally{
if(fis!=null){
try {
fis.close();
} catch (IOException ex) {
Logger.getLogger(Swing.class.getName()).log(Level.SEVERE, null, ex);
}
}
if(fos!=null){
try {
fos.close();
} catch (IOException ex) {
Logger.getLogger(Swing.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
btnReset();
}
private void btnSearchActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
thaolist.clear();
int ht=-1;
if(txtRollno.getText().equals("")){
JOptionPane.showMessageDialog(rootPane, "Nhap Roll No");
}else{
try {
Filel();
for (int i = 0; i < thaolist.size(); i++) {
if(thaolist.get(i).getRollno().equalsIgnoreCase(txtRollno.getText())){
ht=i;
}
if(ht!=-1){
txtName.setText(thaolist.get(i).getName());
txtAddress.setText(thaolist.get(i).getAddress());
txtEmail.setText(thaolist.get(i).getEmail());
txtRollno.setText(thaolist.get(i).getRollno());
txtSex.setText(thaolist.get(i).getSex());
}
}
} catch (IOException ex) {
Logger.getLogger(Swing.class.getName()).log(Level.SEVERE, null, ex);
}
}
table.addRow(new Object[]{table.getRowCount()+1,rollno,email,address,sex});
}
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(Swing.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(Swing.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(Swing.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(Swing.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new Swing().setVisible(true);
}
});
}
// Variables declaration - do not modify
private javax.swing.JTable bltStudent;
private javax.swing.JButton btnReset;
private javax.swing.JButton btnSearch;
private javax.swing.JButton btnUpdate;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel4;
private javax.swing.JLabel jLabel5;
private javax.swing.JPanel jPanel1;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JTextField txtAddress;
private javax.swing.JTextField txtEmail;
private javax.swing.JTextField txtName;
private javax.swing.JTextField txtRollno;
private javax.swing.JTextField txtSex;
// End of variables declaration
private void btnReset() {
txtName.setText("");
txtAddress.setText("");
txtEmail.setText("");
txtRollno.setText("");
txtSex.setText("");
}
private void Filel() throws FileNotFoundException, IOException {
FileReader nis=null;
BufferedReader nos=null;
nis=new FileReader("E:\\BT1907A\\btjava\\swing\\src\\swing01\\student.txt");
nos=new BufferedReader(nis);
String line=null;
while((line=nos.readLine())!=null){
Student thao=new Student();
thao.parseLine(line);
thaolist.add(thao);
}
}
}
/////
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package StudentSwing;
/**
*
* @author ASUS
*/
public class Student {
String name,email,address,sex,rollno;
public Student() {
}
public Student(String name, String email, String address, String sex, String rollno) {
this.name = name;
this.email = email;
this.address = address;
this.sex = sex;
this.rollno = rollno;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getRollno() {
return rollno;
}
public void setRollno(String rollno) {
this.rollno = rollno;
}
public void lineking(String line){
line=line.trim();
String[] thao=line.split(",");
if(thao.length<5){
System.out.println(">>>>>>");
}
name=thao[0];
email=thao[1];
address=thao[2];
sex=thao[3];
rollno=thao[4];
}
}
![Phí Văn Long [T1907A]](https://www.gravatar.com/avatar/5db166b7b74443c5c221e4c0068d6da9.jpg?s=80&d=mm&r=g)
Phí Văn Long
2020-04-01 14:56:15
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package javaSwing;
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.logging.Level;
import java.util.logging.Logger;
import java2.Lesson2.Student;
/**
*
* @author Admin
*/
public class Main extends javax.swing.JFrame {
private Object ex;
/**
* Creates new form Main
*/
public Main() {
initComponents();
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
panel1 = new java.awt.Panel();
HoTen = new javax.swing.JLabel();
GioiTinh = new javax.swing.JLabel();
RollNo = new javax.swing.JLabel();
DiaChi = new javax.swing.JLabel();
Email = new javax.swing.JLabel();
Update = new javax.swing.JButton();
Reset = new javax.swing.JButton();
search = new javax.swing.JButton();
hoten = new javax.swing.JTextField();
gioitinh = new javax.swing.JTextField();
stt = new javax.swing.JTextField();
diachi = new javax.swing.JTextField();
email = new javax.swing.JTextField();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
panel1.setName("JAVASWINGB1"); // NOI18N
HoTen.setText("Ho Ten :");
GioiTinh.setText("Gioi Tinh :");
RollNo.setText("Roll No :");
DiaChi.setText("Dia Chi :");
Email.setText("Email :");
Update.setText("Update");
Update.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
UpdateActionPerformed(evt);
}
});
Reset.setText("Reset");
Reset.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
ResetActionPerformed(evt);
}
});
search.setText("Search");
search.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
searchActionPerformed(evt);
}
});
javax.swing.GroupLayout panel1Layout = new javax.swing.GroupLayout(panel1);
panel1.setLayout(panel1Layout);
panel1Layout.setHorizontalGroup(
panel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(panel1Layout.createSequentialGroup()
.addGap(60, 60, 60)
.addGroup(panel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(panel1Layout.createSequentialGroup()
.addGroup(panel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(Email)
.addComponent(DiaChi)
.addComponent(RollNo)
.addComponent(GioiTinh))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addGroup(panel1Layout.createSequentialGroup()
.addComponent(HoTen)
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))))
.addGroup(panel1Layout.createSequentialGroup()
.addGap(135, 135, 135)
.addGroup(panel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(panel1Layout.createSequentialGroup()
.addGap(67, 67, 67)
.addComponent(Update)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(Reset)
.addGap(179, 179, 179))
.addGroup(panel1Layout.createSequentialGroup()
.addGroup(panel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(panel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING, false)
.addComponent(email, javax.swing.GroupLayout.DEFAULT_SIZE, 461, Short.MAX_VALUE)
.addComponent(diachi)
.addComponent(stt)
.addComponent(gioitinh))
.addComponent(hoten, javax.swing.GroupLayout.PREFERRED_SIZE, 461, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addComponent(search)
.addGap(0, 39, Short.MAX_VALUE))))
);
panel1Layout.setVerticalGroup(
panel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(panel1Layout.createSequentialGroup()
.addGap(52, 52, 52)
.addGroup(panel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(HoTen)
.addComponent(hoten, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(search))
.addGap(37, 37, 37)
.addGroup(panel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(GioiTinh)
.addComponent(gioitinh, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(37, 37, 37)
.addGroup(panel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(RollNo)
.addComponent(stt, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(32, 32, 32)
.addGroup(panel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(DiaChi)
.addComponent(diachi, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(32, 32, 32)
.addGroup(panel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(Email)
.addComponent(email, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(76, 76, 76)
.addGroup(panel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(Update)
.addComponent(Reset))
.addContainerGap(91, Short.MAX_VALUE))
);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(panel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(panel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(0, 0, Short.MAX_VALUE))
);
pack();
}// </editor-fold>
private void searchActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
ArrayList<Student> list = new ArrayList<>();
if (stt.getText() == null) {
System.err.println("RollNo khong ton tai");
}else{
FileReader reader = null;
BufferedReader bufferedReader = null;
try {
String filename = "D://Java/Student.txt";
reader = new FileReader(filename);
bufferedReader = new BufferedReader(reader);
String line ;
while((line = bufferedReader.readLine()) != null) {
Student std = new Student();
std.parseLine(line);
list.add(std);
}
String str = stt.getText();
for (int i = 0; i < list.size(); i++) {
if (str.equalsIgnoreCase(list.get(i).getId())) {
hoten.setText(list.get(i).getName());
diachi.setText(list.get(i).getAddress());
email.setText(list.get(i).getEmail());
gioitinh.setText(list.get(i).getGender());
}else{
System.err.println("Not Find !!");
}
}
} catch (FileNotFoundException exception) {
Logger.getLogger(Student.class.getName()).log(Level.SEVERE, null, exception);
} catch (IOException exception) {
Logger.getLogger(Student.class.getName()).log(Level.SEVERE, null, exception);
} finally {
try {
if(bufferedReader != null) {
bufferedReader.close();
}
if(reader != null) {
reader.close();
}
} catch (IOException exception) {
Logger.getLogger(Student.class.getName()).log(Level.SEVERE, null, exception);
}
}
}
}
private void ResetActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
System.out.println("Resett...");
hoten.setText("");
gioitinh.setText("");
stt.setText("");
diachi.setText("");
email.setText("");
}
private void UpdateActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
FileOutputStream fos = null;
BufferedOutputStream bos = null;
try {
fos = new FileOutputStream("D://Java/Student.txt");
bos = new BufferedOutputStream(fos);
String update = hoten.getText() + "\n" + gioitinh.getText() + "\n" + stt.getText() + "\n" + diachi.getText() + "\n" + email.getText() + "\n";
byte[] b;
b = update.getBytes("utf8");
bos.write(b);
} catch (IOException e) {
System.out.println("Error" + e);
}finally{
if (bos != null){
try {
bos.close();
} catch (IOException e) {
Object exception = null;
Logger.getLogger(Student.class.getName()).log(Level.SEVERE,null,exception);
}
}if(fos != null){
try {
fos.close();
} catch (IOException exception) {
Logger.getLogger(Student.class.getName()).log(Level.SEVERE,null,exception);
}
}
System.out.println("Resett...");
hoten.setText("");
gioitinh.setText("");
stt.setText("");
diachi.setText("");
email.setText("");
System.out.println("XONG");
}
}
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(Main.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(Main.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(Main.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(Main.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new Main().setVisible(true);
}
});
}
// Variables declaration - do not modify
private javax.swing.JLabel DiaChi;
private javax.swing.JLabel Email;
private javax.swing.JLabel GioiTinh;
private javax.swing.JLabel HoTen;
private javax.swing.JButton Reset;
private javax.swing.JLabel RollNo;
private javax.swing.JButton Update;
private javax.swing.JTextField diachi;
private javax.swing.JTextField email;
private javax.swing.JTextField gioitinh;
private javax.swing.JTextField hoten;
private java.awt.Panel panel1;
private javax.swing.JButton search;
private javax.swing.JTextField stt;
// End of variables declaration
}
Student:/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package java2.Lesson2;
/**
*
* @author Admin
*/
public class Student {
String Id,name,gender,address,email;
public Student() {
}
public Student(String Id, String name, String gender, String address, String email) {
this.Id = Id;
this.name = name;
this.gender = gender;
this.address = address;
this.email = email;
}
public String getId() {
return Id;
}
public void setId(String Id) {
this.Id = Id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public void parseLine(String line){
line = line.trim();
String[] param = line.split(",");
if (param.length < 5) {
System.out.println("Data ERROR");
}
Id = param[0];
name = param[1];
gender = param[2];
address = param[3];
email = param[4];
}
}
![thienphu [T1907A]](https://www.gravatar.com/avatar/c4573ea65e411176c1852fd8584f1ab1.jpg?s=80&d=mm&r=g)
thienphu
2020-04-01 13:36:53
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package JavaSwing03;
/**
*
* @author Thien Phu
*/
public class Student {
String hoten, gioiTinh, rollNo, eMail, diaChi;
public Student() {
}
public Student(String hoten, String gioiTinh, String rollNo, String eMail, String diaChi) {
this.hoten = hoten;
this.gioiTinh = gioiTinh;
this.rollNo = rollNo;
this.eMail = eMail;
this.diaChi = diaChi;
}
public String getHoten() {
return hoten;
}
public void setHoten(String hoten) {
this.hoten = hoten;
}
public String getGioiTinh() {
return gioiTinh;
}
public void setGioiTinh(String gioiTinh) {
this.gioiTinh = gioiTinh;
}
public String getRollNo() {
return rollNo;
}
public void setRollNo(String rollNo) {
this.rollNo = rollNo;
}
public String geteMail() {
return eMail;
}
public void seteMail(String eMail) {
this.eMail = eMail;
}
public String getDiaChi() {
return diaChi;
}
public void setDiaChi(String diaChi) {
this.diaChi = diaChi;
}
public String getLine() {
return hoten + "," + gioiTinh + "," + rollNo + "," + eMail + "," + diaChi + "\n";
}
public void parseLine(String line) {
line = line.trim();
String[] chuoi = line.split(",");
if (chuoi.length < 5) {
System.out.println("Data error");
return;
}
this.hoten = chuoi[0];
this.gioiTinh = chuoi[1];
this.rollNo = chuoi[2];
this.eMail = chuoi[3];
this.diaChi = chuoi[4];
}
}
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package JavaSwing03;
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.util.ArrayList;
import java.util.List;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.JOptionPane;
import javax.swing.table.DefaultTableModel;
/**
*
* @author Thien Phu
*/
public class NewJFrame extends javax.swing.JFrame {
List<Student> stdList;
public NewJFrame() {
initComponents();
stdList = new ArrayList<>();
setLocationRelativeTo(this);
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
jLabel1 = new javax.swing.JLabel();
jLabel2 = new javax.swing.JLabel();
tfHoten = new javax.swing.JTextField();
tfRollNo = new javax.swing.JTextField();
tfGioiTinh = new javax.swing.JTextField();
jLabel4 = new javax.swing.JLabel();
jLabel3 = new javax.swing.JLabel();
tfEmail = new javax.swing.JTextField();
jLabel5 = new javax.swing.JLabel();
tfDiaChi = new javax.swing.JTextField();
btUpdate = new javax.swing.JButton();
btReset = new javax.swing.JButton();
btSearch = new javax.swing.JButton();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jLabel1.setText("Ho Ten");
jLabel1.setPreferredSize(new java.awt.Dimension(71, 23));
jLabel2.setText("Gioi Tinh");
jLabel2.setPreferredSize(new java.awt.Dimension(71, 23));
jLabel4.setText("Roll No");
jLabel4.setPreferredSize(new java.awt.Dimension(71, 23));
jLabel3.setText("EMail");
tfEmail.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
tfEmailActionPerformed(evt);
}
});
jLabel5.setText("Dia Chi");
btUpdate.setText("Update");
btUpdate.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btUpdateActionPerformed(evt);
}
});
btReset.setText("Reset");
btReset.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btResetActionPerformed(evt);
}
});
btSearch.setText("Search");
btSearch.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btSearchActionPerformed(evt);
}
});
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(52, 52, 52)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(tfHoten, javax.swing.GroupLayout.PREFERRED_SIZE, 158, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 34, Short.MAX_VALUE)
.addComponent(btSearch))
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(jLabel2, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel4, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addComponent(jLabel3, javax.swing.GroupLayout.PREFERRED_SIZE, 39, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel5))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(tfGioiTinh, javax.swing.GroupLayout.DEFAULT_SIZE, 158, Short.MAX_VALUE)
.addComponent(tfRollNo)
.addComponent(tfEmail)
.addComponent(tfDiaChi)))))
.addGroup(layout.createSequentialGroup()
.addGap(96, 96, 96)
.addComponent(btUpdate)
.addGap(39, 39, 39)
.addComponent(btReset)))
.addContainerGap())
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(33, 33, 33)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(tfHoten, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(btSearch))
.addGap(18, 18, 18)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel2, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(tfGioiTinh, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(13, 13, 13)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(tfRollNo, javax.swing.GroupLayout.PREFERRED_SIZE, 30, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel4, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel3, javax.swing.GroupLayout.PREFERRED_SIZE, 28, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(tfEmail, javax.swing.GroupLayout.PREFERRED_SIZE, 28, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel5, javax.swing.GroupLayout.PREFERRED_SIZE, 27, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(tfDiaChi, javax.swing.GroupLayout.PREFERRED_SIZE, 27, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(30, 30, 30)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(btUpdate)
.addComponent(btReset))
.addContainerGap(20, Short.MAX_VALUE))
);
pack();
}// </editor-fold>
private void tfEmailActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
}
private void btResetActionPerformed(java.awt.event.ActionEvent evt) {
//reset
reset();
}
private void reset() {
tfHoten.setText("");
tfGioiTinh.setText("");
tfRollNo.setText("");
tfEmail.setText("");
tfDiaChi.setText("");
}
private void btUpdateActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
//save to file
Student st = new Student();
st.setHoten(tfHoten.getText());
st.setGioiTinh(tfGioiTinh.getText());
st.setRollNo(tfRollNo.getText());
st.seteMail(tfEmail.getText());
st.setDiaChi(tfDiaChi.getText());
stdList.add(st);
FileOutputStream fos = null;
BufferedOutputStream bos = null;
try {
fos = new FileOutputStream("D:\\baitapjava\\Bientrongjava\\src\\JavaSwing03\\student.txt");
bos = new BufferedOutputStream(fos);
for (Student stdList1 : stdList) {
String line = stdList1.getLine();
System.out.println("Line: " + line);
byte[] b = line.getBytes("utf8");
bos.write(b);
}
} catch (FileNotFoundException ex) {
Logger.getLogger(NewJFrame.class.getName()).log(Level.SEVERE, null, ex);
} catch (UnsupportedEncodingException ex) {
Logger.getLogger(NewJFrame.class.getName()).log(Level.SEVERE, null, ex);
} catch (IOException ex) {
Logger.getLogger(NewJFrame.class.getName()).log(Level.SEVERE, null, ex);
} finally {
try {
if (bos != null) {
bos.close();
}
if (fos != null) {
fos.close();
}
} catch (IOException e) {
Logger.getLogger(NewJFrame.class.getName()).log(Level.SEVERE, null, e);
}
}
System.out.println("Thanh cong");
reset();
}
private void btSearchActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
stdList.clear();
int check = -1;
if (tfRollNo.getText().equals("")) {
JOptionPane.showMessageDialog(rootPane, "Nhap Roll No");
// System.out.println("Co di vao day");
} else {
readFile();
for (int i = 0; i < stdList.size(); i++) {
if (stdList.get(i).getRollNo().equalsIgnoreCase(tfRollNo.getText())) {
check = i;
break;
}
}
if (check != -1) {
tfHoten.setText(stdList.get(check).getHoten());
tfGioiTinh.setText(stdList.get(check).getGioiTinh());
tfRollNo.setText(stdList.get(check).getRollNo());
tfEmail.setText(stdList.get(check).geteMail());
tfDiaChi.setText(stdList.get(check).getDiaChi());
} else {
JOptionPane.showMessageDialog(rootPane, tfRollNo.getText() + " khong co trong danh sach");
}
}
}
private void readFile() {
FileReader fileReader = null;
BufferedReader bufferedReader = null;
try {
fileReader = new FileReader("D:\\baitapjava\\Bientrongjava\\src\\JavaSwing03\\student.txt");
bufferedReader = new BufferedReader(fileReader);
//doc file
String line = null;
while ((line = bufferedReader.readLine()) != null) {
Student st = new Student();
st.parseLine(line);
stdList.add(st);
}
} catch (FileNotFoundException ex) {
Logger.getLogger(NewJFrame.class.getName()).log(Level.SEVERE, null, ex);
} catch (IOException ex) {
Logger.getLogger(NewJFrame.class.getName()).log(Level.SEVERE, null, ex);
}
}
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(NewJFrame.class
.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(NewJFrame.class
.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(NewJFrame.class
.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(NewJFrame.class
.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new NewJFrame().setVisible(true);
}
});
}
// Variables declaration - do not modify
private javax.swing.JButton btReset;
private javax.swing.JButton btSearch;
private javax.swing.JButton btUpdate;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel4;
private javax.swing.JLabel jLabel5;
private javax.swing.JTextField tfDiaChi;
private javax.swing.JTextField tfEmail;
private javax.swing.JTextField tfGioiTinh;
private javax.swing.JTextField tfHoten;
private javax.swing.JTextField tfRollNo;
// End of variables declaration
}
![Trương Công Vinh [T1907A]](https://www.gravatar.com/avatar/223a7e3a46f4a747f81b921fe023fcc4.jpg?s=80&d=mm&r=g)
Trương Công Vinh
2020-04-01 11:50:34
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package JvStr;
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
*
* @author DELL
*/
public class Student extends javax.swing.JFrame {
/**
* Creates new form Student
*/
public Student() {
initComponents();
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
div1 = new javax.swing.JPanel();
jLabel1 = new javax.swing.JLabel();
txtName = new javax.swing.JTextField();
jLabel2 = new javax.swing.JLabel();
txtgender = new javax.swing.JTextField();
jLabel3 = new javax.swing.JLabel();
txtId = new javax.swing.JTextField();
jLabel4 = new javax.swing.JLabel();
txtEmail = new javax.swing.JTextField();
jLabel5 = new javax.swing.JLabel();
TxtAddress = new javax.swing.JTextField();
jButton1 = new javax.swing.JButton();
jButton2 = new javax.swing.JButton();
jButton3 = new javax.swing.JButton();
txterr = new javax.swing.JTextField();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jLabel1.setFont(new java.awt.Font("Tahoma", 0, 24)); // NOI18N
jLabel1.setText(" Ho Ten : ");
txtName.setText(" ");
txtName.setEnabled(false);
txtName.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
txtNameActionPerformed(evt);
}
});
jLabel2.setFont(new java.awt.Font("Tahoma", 0, 24)); // NOI18N
jLabel2.setText(" Giới Tính: ");
txtgender.setText(" ");
txtgender.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
txtgenderActionPerformed(evt);
}
});
jLabel3.setFont(new java.awt.Font("Tahoma", 0, 24)); // NOI18N
jLabel3.setText(" RollNo: ");
txtId.setText(" ");
txtId.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
txtIdActionPerformed(evt);
}
});
jLabel4.setFont(new java.awt.Font("Tahoma", 0, 24)); // NOI18N
jLabel4.setText(" Email : ");
txtEmail.setText(" ");
txtEmail.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
txtEmailActionPerformed(evt);
}
});
jLabel5.setFont(new java.awt.Font("Tahoma", 0, 24)); // NOI18N
jLabel5.setText(" Địa Chỉ: ");
TxtAddress.setText(" ");
TxtAddress.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
TxtAddressActionPerformed(evt);
}
});
jButton1.setBackground(new java.awt.Color(250, 250, 250));
jButton1.setFont(new java.awt.Font("Tahoma", 0, 24)); // NOI18N
jButton1.setText("Update");
jButton1.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton1ActionPerformed(evt);
}
});
jButton2.setBackground(new java.awt.Color(250, 250, 250));
jButton2.setFont(new java.awt.Font("Tahoma", 0, 24)); // NOI18N
jButton2.setText("Reset");
jButton2.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton2ActionPerformed(evt);
}
});
jButton3.setBackground(new java.awt.Color(250, 250, 250));
jButton3.setFont(new java.awt.Font("Tahoma", 0, 24)); // NOI18N
jButton3.setText("Search");
jButton3.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton3ActionPerformed(evt);
}
});
txterr.setBackground(new java.awt.Color(240, 240, 240));
txterr.setForeground(new java.awt.Color(250, 0, 0));
txterr.setBorder(null);
txterr.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
txterrActionPerformed(evt);
}
});
javax.swing.GroupLayout div1Layout = new javax.swing.GroupLayout(div1);
div1.setLayout(div1Layout);
div1Layout.setHorizontalGroup(
div1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(div1Layout.createSequentialGroup()
.addGap(31, 31, 31)
.addGroup(div1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(div1Layout.createSequentialGroup()
.addComponent(jLabel4, javax.swing.GroupLayout.PREFERRED_SIZE, 125, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(txtEmail, javax.swing.GroupLayout.PREFERRED_SIZE, 418, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(div1Layout.createSequentialGroup()
.addComponent(jLabel2, javax.swing.GroupLayout.PREFERRED_SIZE, 125, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(txtgender, javax.swing.GroupLayout.PREFERRED_SIZE, 418, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(div1Layout.createSequentialGroup()
.addComponent(jLabel5, javax.swing.GroupLayout.PREFERRED_SIZE, 125, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(div1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(TxtAddress, javax.swing.GroupLayout.PREFERRED_SIZE, 418, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(div1Layout.createSequentialGroup()
.addComponent(jButton1, javax.swing.GroupLayout.PREFERRED_SIZE, 164, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(81, 81, 81)
.addComponent(jButton2, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))))
.addGroup(div1Layout.createSequentialGroup()
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 125, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(txtName, javax.swing.GroupLayout.PREFERRED_SIZE, 418, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(div1Layout.createSequentialGroup()
.addComponent(jLabel3, javax.swing.GroupLayout.PREFERRED_SIZE, 125, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(txtId, javax.swing.GroupLayout.PREFERRED_SIZE, 418, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addGap(32, 32, 32)
.addGroup(div1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(div1Layout.createSequentialGroup()
.addComponent(txterr, javax.swing.GroupLayout.PREFERRED_SIZE, 153, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(0, 0, Short.MAX_VALUE))
.addComponent(jButton3, javax.swing.GroupLayout.DEFAULT_SIZE, 161, Short.MAX_VALUE))
.addContainerGap())
);
div1Layout.setVerticalGroup(
div1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(div1Layout.createSequentialGroup()
.addGap(60, 60, 60)
.addGroup(div1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jLabel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(txtName)
.addComponent(jButton3, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.DEFAULT_SIZE, 42, Short.MAX_VALUE))
.addGroup(div1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(div1Layout.createSequentialGroup()
.addGap(35, 35, 35)
.addGroup(div1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jLabel2, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(txtgender, javax.swing.GroupLayout.PREFERRED_SIZE, 42, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(38, 38, 38)
.addGroup(div1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jLabel3, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(txtId, javax.swing.GroupLayout.PREFERRED_SIZE, 42, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(33, 33, 33)
.addGroup(div1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jLabel4, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(txtEmail, javax.swing.GroupLayout.PREFERRED_SIZE, 42, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, div1Layout.createSequentialGroup()
.addGap(46, 46, 46)
.addComponent(txterr, javax.swing.GroupLayout.PREFERRED_SIZE, 186, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addGap(39, 39, 39)
.addGroup(div1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jLabel5, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(TxtAddress, javax.swing.GroupLayout.PREFERRED_SIZE, 42, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 41, Short.MAX_VALUE)
.addGroup(div1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jButton1, javax.swing.GroupLayout.PREFERRED_SIZE, 42, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jButton2, javax.swing.GroupLayout.PREFERRED_SIZE, 42, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(30, 30, 30))
);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addComponent(div1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(0, 0, Short.MAX_VALUE))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addComponent(div1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(0, 0, Short.MAX_VALUE))
);
pack();
}// </editor-fold>
private void txtNameActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
}
private void txtgenderActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
}
private void txtIdActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
}
private void txtEmailActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
}
private void TxtAddressActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
}
private void jButton2ActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
txtName.setText("");
TxtAddress.setText("");
txtEmail.setText("");
txtId.setText("");
txtgender.setText("");
}
private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
FileOutputStream fos = null;
BufferedOutputStream bos = null;
try {
fos = new FileOutputStream("d://Java2/Student.txt");
bos = new BufferedOutputStream(fos);
String line = txtName.getText() + "," + txtgender.getText() + "," + txtId.getText() + "," + TxtAddress.getText()+"," + txtEmail.getText() + "\n";
byte[] b;
b = line.getBytes("utf8");
bos.write(b);
} catch (IOException e) {
System.out.println(" Loi ghi file : " + e);
} finally {
if (bos != null) {
try {
bos.close();
} catch (IOException ex) {
Logger.getLogger(Student.class.getName()).log(Level.SEVERE, null, ex);
}
}
if (fos != null) {
try {
fos.close();
} catch (IOException ex) {
Logger.getLogger(Student.class.getName()).log(Level.SEVERE, null, ex);
}
}
txtName.setText("");
TxtAddress.setText("");
txtEmail.setText("");
txtId.setText("");
txtgender.setText("");
System.out.println("Successful !!");
}
}
private void jButton3ActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
ArrayList<objStudent> list = new ArrayList<>();
if (txtId.getText() == null) {
txterr.setText("RollNo --> not Empty !!");
}else{
FileReader reader = null;
BufferedReader bufferedReader = null;
try {
String filename = "d://Java2/Student.txt";
reader = new FileReader(filename);
bufferedReader = new BufferedReader(reader);
//doc du lieu tu FILE
String line = null;
while((line = bufferedReader.readLine()) != null) {
objStudent std = new objStudent();
std.parseLine(line);
list.add(std);
}
String str = txtId.getText();
for (int i = 0; i < list.size(); i++) {
if (str.equalsIgnoreCase(list.get(i).getId())) {
txtName.setText(list.get(i).getName());
TxtAddress.setText(list.get(i).getAddress());
txtEmail.setText(list.get(i).getEmail());
txtgender.setText(list.get(i).getGender());
}else{
System.err.println("Not Find !!");
}
}
} catch (FileNotFoundException ex) {
Logger.getLogger(Student.class.getName()).log(Level.SEVERE, null, ex);
} catch (IOException ex) {
Logger.getLogger(Student.class.getName()).log(Level.SEVERE, null, ex);
} finally {
try {
if(bufferedReader != null) {
bufferedReader.close();
}
if(reader != null) {
reader.close();
}
} catch (IOException ex) {
Logger.getLogger(Student.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
}
private void txterrActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
}
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(Student.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(Student.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(Student.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(Student.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new Student().setVisible(true);
}
});
}
// Variables declaration - do not modify
private javax.swing.JTextField TxtAddress;
private javax.swing.JPanel div1;
private javax.swing.JButton jButton1;
private javax.swing.JButton jButton2;
private javax.swing.JButton jButton3;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel4;
private javax.swing.JLabel jLabel5;
private javax.swing.JTextField txtEmail;
private javax.swing.JTextField txtId;
private javax.swing.JTextField txtName;
private javax.swing.JTextField txterr;
private javax.swing.JTextField txtgender;
// End of variables declaration
}
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package JvStr;
/**
*
* @author DELL
*/
public class objStudent {
String name , id , gender , address , email;
public objStudent() {
}
public objStudent(String name, String id, String gender, String address, String email) {
this.name = name;
this.id = id;
this.gender = gender;
this.address = address;
this.email = email;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public void parseLine(String line){
line = line.trim();
String[] param = line.split(",");
if (param.length<5 ) {
System.err.println("Data err !!");
}
name = param[0];
gender = param[1];
id = param[2];
address = param[3];
email = param[4];
}
}