By GokiSoft.com|
19:40 27/03/2023|
Java Advanced
Bài Tập Quản Lý Sinh Viên - CSDL - Java Swing
Xây dựng chương trình như hình sau
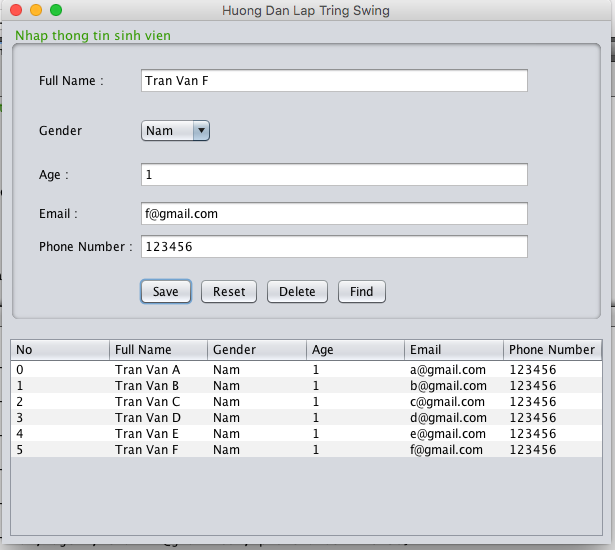
Yêu cầu :
- Khi người dùng click save -> add danh sách sinh viên vào bảng quản lý
- Khi người dùng click vào reset -> Xoá dữ liệu trong form nhập
- Khi người dùng click vào delete -> Xoá sinh viên đang được lựa chọn trong bảng quản lý
- Khi người dùng click vào find -> Hiển thị lên dialog nhập tên sinh viên cần tìm kiếm -> Hiển thị lại dữ liệu sinh viên tìm thấy trong bảng, nếu ko tìm thấy sinh viên nào thì hiển thị dialog thông báo ko tìm thấy bất kỳ sinh viên nào.
Tags:
Phản hồi từ học viên
5
(Dựa trên đánh giá ngày hôm nay)
![thienphu [T1907A]](https://www.gravatar.com/avatar/c4573ea65e411176c1852fd8584f1ab1.jpg?s=80&d=mm&r=g)
thienphu
2020-04-04 10:12:43
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package JavaSwingQuanliSv;
/**
*
* @author Thien Phu
*/
public class Student {
String fullname;
String email, gender;
int age, phone;
public Student() {
}
public Student(String fullname, String email, String gender, int age, int phone) {
this.fullname = fullname;
this.email = email;
this.gender = gender;
this.age = age;
this.phone = phone;
}
public String getFullname() {
return fullname;
}
public void setFullname(String fullname) {
this.fullname = fullname;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public int getPhone() {
return phone;
}
public void setPhone(int phone) {
this.phone = phone;
}
@Override
public String toString() {
return "Student{" + "fullname=" + fullname + ", email=" + email + ", gender=" + gender + ", age=" + age + ", phone=" + phone + '}';
}
public void hienthi(){
System.out.println(toString());
}
}
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package JavaSwingQuanliSv;
import java.awt.event.ActionEvent;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.List;
import java.util.Vector;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.naming.spi.DirStateFactory.Result;
import javax.swing.JOptionPane;
import javax.swing.table.DefaultTableModel;
/**
*
* @author Thien Phu
*/
public class GiaoDien extends javax.swing.JFrame {
DefaultTableModel defaultTableModel;
List<Student> stdList;
FindStudent findStudent;
public GiaoDien() {
initComponents();
setLocationRelativeTo(this);
defaultTableModel = (DefaultTableModel) jtableStudent.getModel();
stdList = new ArrayList<>();
readData();
findStudent = new FindStudent(this, rootPaneCheckingEnabled);
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
jLabel4 = new javax.swing.JLabel();
jScrollPane1 = new javax.swing.JScrollPane();
jTable1 = new javax.swing.JTable();
jScrollPane3 = new javax.swing.JScrollPane();
jTable3 = new javax.swing.JTable();
jScrollPane4 = new javax.swing.JScrollPane();
jTable4 = new javax.swing.JTable();
jDialog1 = new javax.swing.JDialog();
jDialog2 = new javax.swing.JDialog();
jDialog3 = new javax.swing.JDialog();
jDialog4 = new javax.swing.JDialog();
jPanel1 = new javax.swing.JPanel();
jLabel2 = new javax.swing.JLabel();
jCbGender = new javax.swing.JComboBox();
tfFullname = new javax.swing.JTextField();
jLabel3 = new javax.swing.JLabel();
tfAge = new javax.swing.JTextField();
jLabel5 = new javax.swing.JLabel();
tfEmail = new javax.swing.JTextField();
jLabel6 = new javax.swing.JLabel();
jLabel1 = new javax.swing.JLabel();
tfPhone = new javax.swing.JTextField();
btSave = new javax.swing.JButton();
btReset = new javax.swing.JButton();
btDelete = new javax.swing.JButton();
btFind = new javax.swing.JButton();
jScrollPane2 = new javax.swing.JScrollPane();
jtableStudent = new javax.swing.JTable();
jLabel4.setText("jLabel4");
jTable1.setModel(new javax.swing.table.DefaultTableModel(
new Object [][] {
{null, null, null, null},
{null, null, null, null},
{null, null, null, null},
{null, null, null, null}
},
new String [] {
"Title 1", "Title 2", "Title 3", "Title 4"
}
));
jScrollPane1.setViewportView(jTable1);
jTable3.setModel(new javax.swing.table.DefaultTableModel(
new Object [][] {
{null, null, null, null},
{null, null, null, null},
{null, null, null, null},
{null, null, null, null}
},
new String [] {
"Title 1", "Title 2", "Title 3", "Title 4"
}
));
jScrollPane3.setViewportView(jTable3);
jTable4.setModel(new javax.swing.table.DefaultTableModel(
new Object [][] {
{null, null, null, null},
{null, null, null, null},
{null, null, null, null},
{null, null, null, null}
},
new String [] {
"Title 1", "Title 2", "Title 3", "Title 4"
}
));
jScrollPane4.setViewportView(jTable4);
javax.swing.GroupLayout jDialog1Layout = new javax.swing.GroupLayout(jDialog1.getContentPane());
jDialog1.getContentPane().setLayout(jDialog1Layout);
jDialog1Layout.setHorizontalGroup(
jDialog1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGap(0, 400, Short.MAX_VALUE)
);
jDialog1Layout.setVerticalGroup(
jDialog1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGap(0, 300, Short.MAX_VALUE)
);
javax.swing.GroupLayout jDialog2Layout = new javax.swing.GroupLayout(jDialog2.getContentPane());
jDialog2.getContentPane().setLayout(jDialog2Layout);
jDialog2Layout.setHorizontalGroup(
jDialog2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGap(0, 400, Short.MAX_VALUE)
);
jDialog2Layout.setVerticalGroup(
jDialog2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGap(0, 300, Short.MAX_VALUE)
);
javax.swing.GroupLayout jDialog3Layout = new javax.swing.GroupLayout(jDialog3.getContentPane());
jDialog3.getContentPane().setLayout(jDialog3Layout);
jDialog3Layout.setHorizontalGroup(
jDialog3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGap(0, 400, Short.MAX_VALUE)
);
jDialog3Layout.setVerticalGroup(
jDialog3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGap(0, 300, Short.MAX_VALUE)
);
javax.swing.GroupLayout jDialog4Layout = new javax.swing.GroupLayout(jDialog4.getContentPane());
jDialog4.getContentPane().setLayout(jDialog4Layout);
jDialog4Layout.setHorizontalGroup(
jDialog4Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGap(0, 400, Short.MAX_VALUE)
);
jDialog4Layout.setVerticalGroup(
jDialog4Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGap(0, 300, Short.MAX_VALUE)
);
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jPanel1.setBorder(javax.swing.BorderFactory.createTitledBorder("Nhap thong tin sinh vien"));
jPanel1.setToolTipText("Quan li Sinh Vien");
jPanel1.setName("Quanli"); // NOI18N
jLabel2.setText("Gender");
jLabel2.setMaximumSize(new java.awt.Dimension(46, 14));
jLabel2.setMinimumSize(new java.awt.Dimension(46, 14));
jLabel2.setPreferredSize(new java.awt.Dimension(46, 14));
jCbGender.setModel(new javax.swing.DefaultComboBoxModel(new String[] { "Nam", "Nu" }));
jCbGender.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jCbGenderActionPerformed(evt);
}
});
tfFullname.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
tfFullnameActionPerformed(evt);
}
});
jLabel3.setText("Age :");
tfAge.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
tfAgeActionPerformed(evt);
}
});
jLabel5.setText("Email :");
tfEmail.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
tfEmailActionPerformed(evt);
}
});
jLabel6.setText("Full Name");
jLabel1.setText("Phone");
tfPhone.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
tfPhoneActionPerformed(evt);
}
});
btSave.setText("Save");
btSave.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btSaveActionPerformed(evt);
}
});
btReset.setText("Reset");
btReset.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btResetActionPerformed(evt);
}
});
btDelete.setText("Delete");
btDelete.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btDeleteActionPerformed(evt);
}
});
btFind.setText("Find");
btFind.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btFindActionPerformed(evt);
}
});
javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1);
jPanel1.setLayout(jPanel1Layout);
jPanel1Layout.setHorizontalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, jPanel1Layout.createSequentialGroup()
.addGap(0, 103, Short.MAX_VALUE)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel2, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(jLabel6)
.addGap(44, 44, 44)
.addComponent(tfFullname, javax.swing.GroupLayout.PREFERRED_SIZE, 266, javax.swing.GroupLayout.PREFERRED_SIZE))
.addComponent(jLabel3)
.addComponent(jLabel5))
.addGap(123, 123, 123))
.addGroup(jPanel1Layout.createSequentialGroup()
.addGap(99, 99, 99)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(jLabel1)
.addGap(64, 64, 64)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(tfPhone, javax.swing.GroupLayout.PREFERRED_SIZE, 266, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jCbGender, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(tfAge, javax.swing.GroupLayout.PREFERRED_SIZE, 266, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(tfEmail, javax.swing.GroupLayout.PREFERRED_SIZE, 266, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(82, 82, 82))
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(btSave)
.addGap(26, 26, 26)
.addComponent(btReset)
.addGap(37, 37, 37)
.addComponent(btDelete)
.addGap(30, 30, 30)
.addComponent(btFind)
.addGap(50, 50, 50))))
);
jPanel1Layout.setVerticalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addGap(18, 18, 18)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(tfFullname, javax.swing.GroupLayout.PREFERRED_SIZE, 34, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel6, javax.swing.GroupLayout.PREFERRED_SIZE, 34, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(28, 28, 28)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jCbGender, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel2, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel3, javax.swing.GroupLayout.PREFERRED_SIZE, 34, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(tfAge, javax.swing.GroupLayout.PREFERRED_SIZE, 34, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel5, javax.swing.GroupLayout.PREFERRED_SIZE, 34, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(tfEmail, javax.swing.GroupLayout.PREFERRED_SIZE, 34, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 34, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(tfPhone, javax.swing.GroupLayout.PREFERRED_SIZE, 34, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 29, Short.MAX_VALUE)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(btSave)
.addComponent(btReset)
.addComponent(btDelete)
.addComponent(btFind))
.addContainerGap())
);
jtableStudent.setModel(new javax.swing.table.DefaultTableModel(
new Object [][] {
},
new String [] {
"No", "Full Name", "Gender", "Age", "Email", "Phone Number"
}
));
jtableStudent.addMouseListener(new java.awt.event.MouseAdapter() {
public void mouseClicked(java.awt.event.MouseEvent evt) {
jtableStudentMouseClicked(evt);
}
});
jScrollPane2.setViewportView(jtableStudent);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(22, 22, 22)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jPanel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jScrollPane2))
.addContainerGap(20, Short.MAX_VALUE))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(jPanel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(29, 29, 29)
.addComponent(jScrollPane2, javax.swing.GroupLayout.DEFAULT_SIZE, 120, Short.MAX_VALUE)
.addContainerGap())
);
jPanel1.getAccessibleContext().setAccessibleName("Quan li Sinh Vien");
pack();
}// </editor-fold>
private void tfFullnameActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
}
private void jCbGenderActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
}
private void tfAgeActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
}
private void tfEmailActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
}
private void tfPhoneActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
}
private void btSaveActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
//save information list student into file
Student st = new Student();
st.setFullname(tfFullname.getText());
st.setGender((String) jCbGender.getSelectedItem());
st.setAge(Integer.parseInt(tfAge.getText()));
st.setEmail(tfEmail.getText());
st.setPhone(Integer.parseInt(tfPhone.getText()));
// stdList.add(st);
inserIntoMySql(st);
readData();
}
private void btResetActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
tfFullname.setText("");
tfAge.setText("");
tfEmail.setText("");
tfPhone.setText("");
}
private void btDeleteActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
//delete one student in student list
Connection conn = null;
Statement statement = null;
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/t1907a", "root", "");
String sql = "DELETE FROM students WHERE fullname =" + "'" + tfFullname.getText() + "'";
statement = conn.createStatement();
statement.executeUpdate(sql);
} catch (ClassNotFoundException | SQLException ex) {
Logger.getLogger(GiaoDien.class.getName()).log(Level.SEVERE, null, ex);
} finally {
if (conn != null) {
try {
conn.close();
} catch (SQLException ex) {
Logger.getLogger(GiaoDien.class.getName()).log(Level.SEVERE, null, ex);
}
}
if (statement != null) {
try {
statement.close();
} catch (SQLException ex) {
Logger.getLogger(GiaoDien.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
readData();
}
private void jtableStudentMouseClicked(java.awt.event.MouseEvent evt) {
//
int index = jtableStudent.getSelectedRow();
tfFullname.setText(stdList.get(index).getFullname());
if (stdList.get(index).getGender().equalsIgnoreCase("Nu")) {
jCbGender.setSelectedIndex(1);
} else {
jCbGender.setSelectedIndex(0);
}
tfAge.setText(String.valueOf(stdList.get(index).getAge()));
tfEmail.setText(stdList.get(index).getEmail());
tfPhone.setText(String.valueOf(stdList.get(index).getPhone()));
}
private void btFindActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
//fint student in studentlist
findStudent.setVisible(true);
//System.out.println("Chuyen sang Giao dien:" + findStudent.dataShare);
int check = -1;
for (int i = 0; i < stdList.size(); i++) {
if (findStudent.dataShare.equalsIgnoreCase(stdList.get(i).getFullname())) {
check = i;
break;
}
}
if (check != -1) {
defaultTableModel.setRowCount(0);
defaultTableModel.addRow(new Object[]{1,
stdList.get(check).getFullname(),
stdList.get(check).getGender(),
stdList.get(check).getAge(),
stdList.get(check).getEmail(),
stdList.get(check).getPhone()});
} else {
JOptionPane.showMessageDialog(rootPane, "Khong tim thay sinh vien nao");
}
}
private void inserIntoMySql(Student std) {
Connection conn = null;
PreparedStatement preparedStatement = null;
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/t1907a", "root", "");
//query
String sql = "insert into students(fullname,gender,age,email,phone)values (?,?,?,?,?)";
// System.out.println("Chay qua day");
preparedStatement = conn.prepareStatement(sql);
preparedStatement.setString(1, std.getFullname());
preparedStatement.setString(2, std.getGender());
preparedStatement.setInt(3, std.getAge());
preparedStatement.setString(4, std.getEmail());
preparedStatement.setInt(5, std.getPhone());
preparedStatement.execute();
} catch (SQLException ex) {
Logger.getLogger(GiaoDien.class
.getName()).log(Level.SEVERE, null, ex);
} catch (ClassNotFoundException ex) {
Logger.getLogger(GiaoDien.class
.getName()).log(Level.SEVERE, null, ex);
} finally {
if (preparedStatement != null) {
try {
preparedStatement.close();
} catch (SQLException ex) {
Logger.getLogger(GiaoDien.class
.getName()).log(Level.SEVERE, null, ex);
}
}
if (conn != null) {
try {
conn.close();
} catch (SQLException ex) {
Logger.getLogger(GiaoDien.class
.getName()).log(Level.SEVERE, null, ex);
}
}
}
// System.err.println("Chay qua day");
}
//doc du lieu tu my Sql
private void readData() {
stdList.clear();
Connection conn = null;
Statement statement = null;
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/t1907a", "root", "");
String sql = "select * from students";
statement = conn.createStatement();
ResultSet resultSet = statement.executeQuery(sql);
//doc du lieu từng hàng trong mysql
while (resultSet.next()) {
int getphone = Integer.parseInt(resultSet.getString("phone"));
int getAge = Integer.parseInt(resultSet.getString("age"));
Student st = new Student(resultSet.getString("fullname"),
resultSet.getString("email"),
resultSet.getString("gender"),
getAge, getphone);
stdList.add(st);
}
} catch (SQLException ex) {
Logger.getLogger(GiaoDien.class
.getName()).log(Level.SEVERE, null, ex);
} catch (ClassNotFoundException ex) {
Logger.getLogger(GiaoDien.class
.getName()).log(Level.SEVERE, null, ex);
} finally {
if (conn != null) {
try {
conn.close();
} catch (SQLException ex) {
Logger.getLogger(GiaoDien.class
.getName()).log(Level.SEVERE, null, ex);
}
}
if (statement != null) {
try {
statement.close();
} catch (SQLException ex) {
Logger.getLogger(GiaoDien.class
.getName()).log(Level.SEVERE, null, ex);
}
}
}
display();
// System.out.println("Co chay vao day");
}
public void display() {
defaultTableModel.setRowCount(0);
for (Student stdList1 : stdList) {
Vector vtdata = new Vector();
vtdata.add(defaultTableModel.getRowCount() + 1);
vtdata.add(stdList1.getFullname());
vtdata.add(stdList1.getGender());
vtdata.add(stdList1.getAge());
vtdata.add(stdList1.getEmail());
vtdata.add(stdList1.getPhone());
defaultTableModel.addRow(vtdata);
}
}
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(GiaoDien.class
.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(GiaoDien.class
.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(GiaoDien.class
.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(GiaoDien.class
.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new GiaoDien().setVisible(true);
}
});
}
// Variables declaration - do not modify
private javax.swing.JButton btDelete;
private javax.swing.JButton btFind;
private javax.swing.JButton btReset;
private javax.swing.JButton btSave;
private javax.swing.JComboBox jCbGender;
private javax.swing.JDialog jDialog1;
private javax.swing.JDialog jDialog2;
private javax.swing.JDialog jDialog3;
private javax.swing.JDialog jDialog4;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel4;
private javax.swing.JLabel jLabel5;
private javax.swing.JLabel jLabel6;
private javax.swing.JPanel jPanel1;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JScrollPane jScrollPane2;
private javax.swing.JScrollPane jScrollPane3;
private javax.swing.JScrollPane jScrollPane4;
private javax.swing.JTable jTable1;
private javax.swing.JTable jTable3;
private javax.swing.JTable jTable4;
private javax.swing.JTable jtableStudent;
private javax.swing.JTextField tfAge;
private javax.swing.JTextField tfEmail;
private javax.swing.JTextField tfFullname;
private javax.swing.JTextField tfPhone;
// End of variables declaration
}
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package JavaSwingQuanliSv;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
/**
*
* @author Thien Phu
*/
public class FindStudent extends javax.swing.JDialog {
/**
* Creates new form FindStudnet
*/
static String dataShare;;
public FindStudent(java.awt.Frame parent, boolean modal) {
super(parent, modal);
initComponents();
setLocationRelativeTo(this);
dataShare="";
}
private FindStudent(JFrame jFrame, boolean b) {
throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates.
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
jLabel1 = new javax.swing.JLabel();
btFind = new javax.swing.JButton();
tfullname = new javax.swing.JTextField();
setDefaultCloseOperation(javax.swing.WindowConstants.DISPOSE_ON_CLOSE);
jLabel1.setText("Full Name");
btFind.setText("Find");
btFind.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btFindActionPerformed(evt);
}
});
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addContainerGap()
.addComponent(jLabel1)
.addGap(33, 33, 33)
.addComponent(tfullname, javax.swing.GroupLayout.DEFAULT_SIZE, 209, Short.MAX_VALUE)
.addGap(18, 18, 18)
.addComponent(btFind, javax.swing.GroupLayout.PREFERRED_SIZE, 67, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(17, 17, 17))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(35, 35, 35)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(tfullname, javax.swing.GroupLayout.PREFERRED_SIZE, 27, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(btFind))
.addContainerGap(127, Short.MAX_VALUE))
);
pack();
}// </editor-fold>
private String line() {
return tfullname.getText();
}
private void btFindActionPerformed(java.awt.event.ActionEvent evt) {
System.out.println("Chuyen du lieu");
dataShare = line();
System.out.println("data: "+dataShare);
tfullname.setText("");
this.setVisible(false);
}
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(FindStudent.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(FindStudent.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(FindStudent.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(FindStudent.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
//</editor-fold>
/* Create and display the dialog */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
FindStudent dialog = new FindStudent(new javax.swing.JFrame(), true);
dialog.addWindowListener(new java.awt.event.WindowAdapter() {
@Override
public void windowClosing(java.awt.event.WindowEvent e) {
System.exit(0);
}
});
dialog.setVisible(true);
}
});
}
// Variables declaration - do not modify
private javax.swing.JButton btFind;
private javax.swing.JLabel jLabel1;
private javax.swing.JTextField tfullname;
// End of variables declaration
}