By GokiSoft.com|
15:44 24/07/2023|
Java Advanced
Chương trình quản lý sản phẩm - quản lý tin tức - quản lý bán hàng - Lập trình Java
THiết kế cách chức năng giao diện của chương trình sau
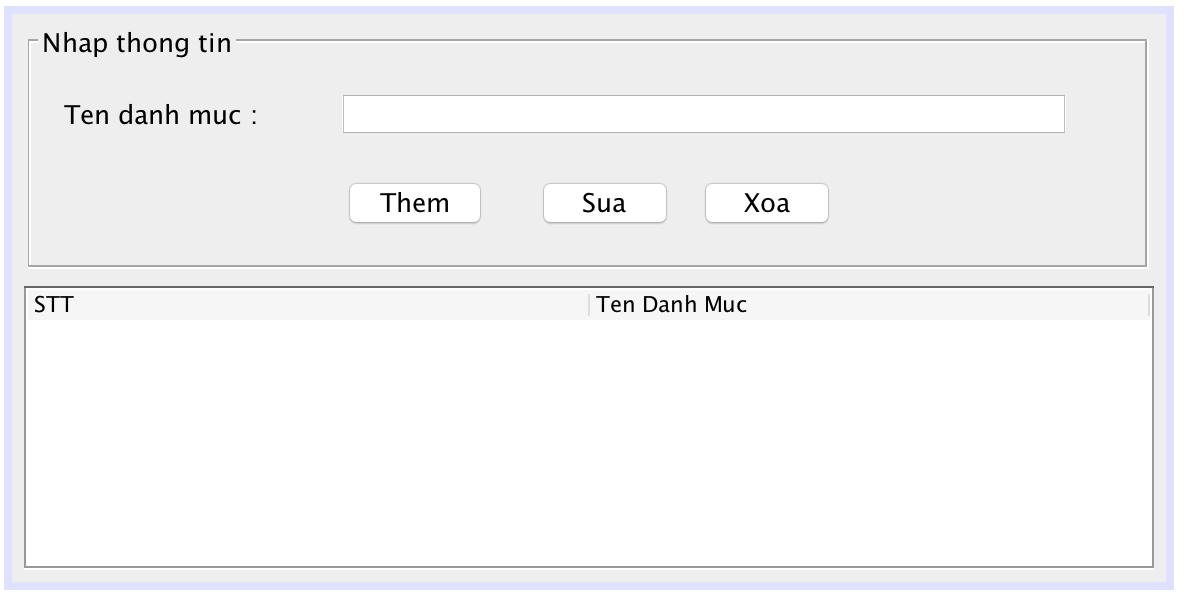
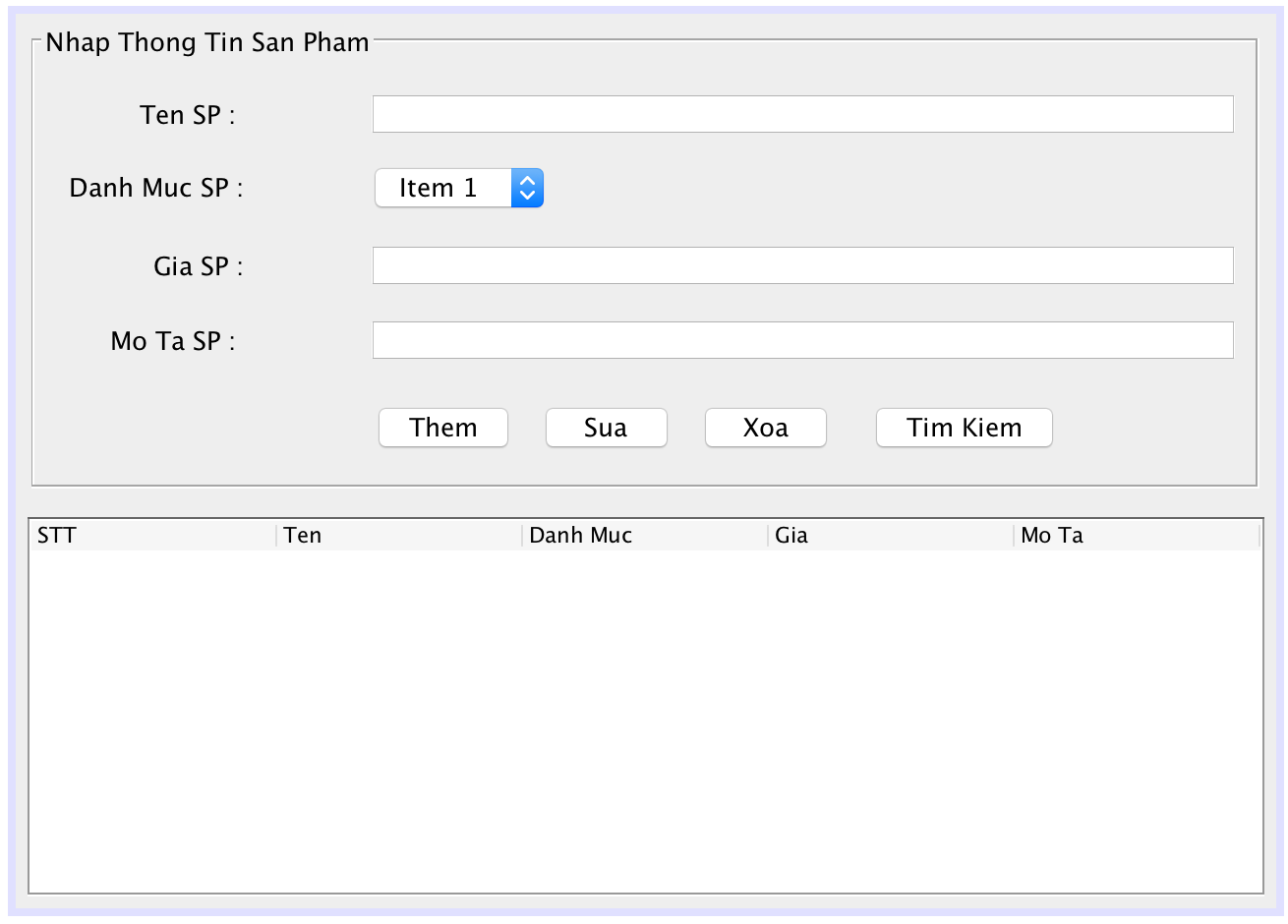
Yêu cầu:
- Tạo file category.xml -> lưu thông tin danh mục sản phẩm
- Tạo file product.json -> lưu thông tin sản phẩm.
Viết chức năng chương trình trên.
Tags:
Phản hồi từ học viên
5
(Dựa trên đánh giá ngày hôm nay)
![TRẦN VĂN ĐIỆP [Teacher]](https://www.gravatar.com/avatar/fc6ba9324e017d540af3613b3a77dd21.jpg?s=80&d=mm&r=g)
TRẦN VĂN ĐIỆP
2021-04-14 10:25:06
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package aptech.bt2249;
import aptech.xml.lesson01.LibraryParser;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.util.ArrayList;
import java.util.List;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.JOptionPane;
import javax.swing.table.DefaultTableModel;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
import org.xml.sax.SAXException;
/**
*
* @author Diep.Tran
*/
public class CategoryFrame extends javax.swing.JFrame {
List<String> dataList = new ArrayList<>();
int currentIndex = -1;
DefaultTableModel tableModel;
/**
* Creates new form CategoryFrame
*/
public CategoryFrame() {
initComponents();
tableModel = (DefaultTableModel) tblCategory.getModel();
tblCategory.addMouseListener(new MouseListener() {
@Override
public void mouseClicked(MouseEvent e) {
currentIndex = tblCategory.getSelectedRow();
txtCategoryName.setText(dataList.get(currentIndex));
}
@Override
public void mousePressed(MouseEvent e) {
}
@Override
public void mouseReleased(MouseEvent e) {
}
@Override
public void mouseEntered(MouseEvent e) {
}
@Override
public void mouseExited(MouseEvent e) {
}
});
//Doc noi dung xml
try {
File file = new File("category.xml");
SAXParserFactory factory = SAXParserFactory.newInstance();
SAXParser parser;
parser = factory.newSAXParser();
CategoryHadler hadler = new CategoryHadler();
parser.parse(file, hadler);
dataList = hadler.dataList;
showData();
} catch (ParserConfigurationException ex) {
Logger.getLogger(CategoryFrame.class.getName()).log(Level.SEVERE, null, ex);
} catch (SAXException ex) {
Logger.getLogger(CategoryFrame.class.getName()).log(Level.SEVERE, null, ex);
} catch (IOException ex) {
Logger.getLogger(CategoryFrame.class.getName()).log(Level.SEVERE, null, ex);
}
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
jPanel1 = new javax.swing.JPanel();
jLabel1 = new javax.swing.JLabel();
txtCategoryName = new javax.swing.JTextField();
btnAdd = new javax.swing.JButton();
btnEdit = new javax.swing.JButton();
btnDelete = new javax.swing.JButton();
jScrollPane1 = new javax.swing.JScrollPane();
tblCategory = new javax.swing.JTable();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
setTitle("Category Form");
jPanel1.setBorder(javax.swing.BorderFactory.createTitledBorder("Category Input"));
jLabel1.setText("Category Name:");
btnAdd.setText("Add");
btnAdd.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnAddActionPerformed(evt);
}
});
btnEdit.setText("Edit");
btnEdit.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnEditActionPerformed(evt);
}
});
btnDelete.setText("Delete");
btnDelete.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnDeleteActionPerformed(evt);
}
});
javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1);
jPanel1.setLayout(jPanel1Layout);
jPanel1Layout.setHorizontalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addGap(19, 19, 19)
.addComponent(jLabel1)
.addGap(39, 39, 39)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(btnAdd)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(btnEdit)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(btnDelete))
.addComponent(txtCategoryName, javax.swing.GroupLayout.PREFERRED_SIZE, 323, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(30, Short.MAX_VALUE))
);
jPanel1Layout.setVerticalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addGap(16, 16, 16)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel1)
.addComponent(txtCategoryName, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(btnAdd)
.addComponent(btnEdit)
.addComponent(btnDelete))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
tblCategory.setModel(new javax.swing.table.DefaultTableModel(
new Object [][] {
},
new String [] {
"ID", "Category Name"
}
) {
boolean[] canEdit = new boolean [] {
false, false
};
public boolean isCellEditable(int rowIndex, int columnIndex) {
return canEdit [columnIndex];
}
});
jScrollPane1.setViewportView(tblCategory);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jPanel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jScrollPane1))
.addContainerGap())
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(jPanel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 129, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
pack();
}// </editor-fold>
private void btnAddActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
if(currentIndex >= 0) {
JOptionPane.showMessageDialog(rootPane, "Not add");
return;
}
String categoryName = txtCategoryName.getText();
dataList.add(categoryName);
tableModel.addRow(new Object[] {tableModel.getRowCount() + 1, categoryName});
saveFile();
}
void saveFile() {
FileOutputStream fos = null;
try {
fos = new FileOutputStream("category.xml");
String xml = "";
for (String value : dataList) {
xml += "<category>"+value+"</category>\n";
}
xml = "<?xml version=\"1.0\" encoding=\"UTF-8\"?>\n" +
"\n" +
"<categories>\n" + xml +
"</categories>";
byte[] data = xml.getBytes("utf8");
fos.write(data);
} catch (FileNotFoundException ex) {
Logger.getLogger(CategoryFrame.class.getName()).log(Level.SEVERE, null, ex);
} catch (UnsupportedEncodingException ex) {
Logger.getLogger(CategoryFrame.class.getName()).log(Level.SEVERE, null, ex);
} catch (IOException ex) {
Logger.getLogger(CategoryFrame.class.getName()).log(Level.SEVERE, null, ex);
} finally {
try {
fos.close();
} catch (IOException ex) {
Logger.getLogger(CategoryFrame.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
void showData() {
tableModel.setRowCount(0);
for (String value : dataList) {
tableModel.addRow(new Object[] {tableModel.getRowCount() + 1, value});
}
}
private void btnEditActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
String value = txtCategoryName.getText();
if(currentIndex >= 0) {
dataList.set(currentIndex, value);
currentIndex = -1;
saveFile();
showData();
} else {
JOptionPane.showMessageDialog(rootPane, "Not edit");
}
}
private void btnDeleteActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
String value = txtCategoryName.getText();
if(currentIndex >= 0) {
dataList.remove(currentIndex);
currentIndex = -1;
saveFile();
showData();
} else {
JOptionPane.showMessageDialog(rootPane, "Not delete");
}
}
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(CategoryFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(CategoryFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(CategoryFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(CategoryFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new CategoryFrame().setVisible(true);
}
});
}
// Variables declaration - do not modify
private javax.swing.JButton btnAdd;
private javax.swing.JButton btnDelete;
private javax.swing.JButton btnEdit;
private javax.swing.JLabel jLabel1;
private javax.swing.JPanel jPanel1;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JTable tblCategory;
private javax.swing.JTextField txtCategoryName;
// End of variables declaration
}
![TRẦN VĂN ĐIỆP [Teacher]](https://www.gravatar.com/avatar/fc6ba9324e017d540af3613b3a77dd21.jpg?s=80&d=mm&r=g)
TRẦN VĂN ĐIỆP
2021-04-14 10:05:49
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package aptech.bt2249;
import java.util.ArrayList;
import java.util.List;
import org.xml.sax.Attributes;
import org.xml.sax.SAXException;
import org.xml.sax.helpers.DefaultHandler;
/**
*
* @author Diep.Tran
*/
public class CategoryHadler extends DefaultHandler{
List<String> dataList = new ArrayList<>();
boolean isCategory = false;
@Override
public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException {
if(qName.equalsIgnoreCase("category")) {
isCategory = true;
}
}
@Override
public void endElement(String uri, String localName, String qName) throws SAXException {
if(qName.equalsIgnoreCase("category")) {
isCategory = false;
}
}
@Override
public void characters(char[] ch, int start, int length) throws SAXException {
if(isCategory) {
String value = new String(ch, start, length);
dataList.add(value);
}
}
}
![Nguyễn Tiến Đạt [T2008A]](https://www.gravatar.com/avatar/b5819cd0adc95c727c7ad0c2bcf6098b.jpg?s=80&d=mm&r=g)
Nguyễn Tiến Đạt
2021-04-14 09:20:23
#Category.java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package Java2.lesson10;
/**
*
* @author MyPC
*/
public class Category {
String categoryName;
public Category() {
}
public Category(String categoryName) {
this.categoryName = categoryName;
}
public String getCategoryName() {
return categoryName;
}
public void setCategoryName(String categoryName) {
this.categoryName = categoryName;
}
public String toXML(){
return" " + "<category>"+categoryName+"</category>\n";
}
}
#Product.java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package Java2.lesson10;
/**
*
* @author MyPC
*/
public class Product {
String name, categoryName, description;
float price;
public Product() {
}
public Product(String name, String categoryName, String description, float price) {
this.name = name;
this.categoryName = categoryName;
this.description = description;
this.price = price;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getCategoryName() {
return categoryName;
}
public void setCategoryName(String categoryName) {
this.categoryName = categoryName;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public float getPrice() {
return price;
}
public void setPrice(float price) {
this.price = price;
}
@Override
public String toString() {
return "Product{" + "name=" + name + ", categoryName=" + categoryName + ", description=" + description + ", price=" + price + '}';
}
}
#ProductFrame.form
<?xml version="1.0" encoding="UTF-8" ?>
<Form version="1.3" maxVersion="1.9" type="org.netbeans.modules.form.forminfo.JFrameFormInfo">
<Properties>
<Property name="defaultCloseOperation" type="int" value="3"/>
</Properties>
<SyntheticProperties>
<SyntheticProperty name="formSizePolicy" type="int" value="1"/>
<SyntheticProperty name="generateCenter" type="boolean" value="false"/>
</SyntheticProperties>
<AuxValues>
<AuxValue name="FormSettings_autoResourcing" type="java.lang.Integer" value="0"/>
<AuxValue name="FormSettings_autoSetComponentName" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_generateFQN" type="java.lang.Boolean" value="true"/>
<AuxValue name="FormSettings_generateMnemonicsCode" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_i18nAutoMode" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_layoutCodeTarget" type="java.lang.Integer" value="1"/>
<AuxValue name="FormSettings_listenerGenerationStyle" type="java.lang.Integer" value="0"/>
<AuxValue name="FormSettings_variablesLocal" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_variablesModifier" type="java.lang.Integer" value="2"/>
</AuxValues>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="jPanel1" max="32767" attributes="0"/>
<Component id="jPanel2" max="32767" attributes="0"/>
</Group>
<EmptySpace max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Component id="jPanel1" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jPanel2" min="-2" max="-2" attributes="0"/>
<EmptySpace max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Container class="javax.swing.JPanel" name="jPanel1">
<Properties>
<Property name="border" type="javax.swing.border.Border" editor="org.netbeans.modules.form.editors2.BorderEditor">
<Border info="org.netbeans.modules.form.compat2.border.TitledBorderInfo">
<TitledBorder title="Nhap thong tin"/>
</Border>
</Property>
</Properties>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="216" max="-2" attributes="0"/>
<Component id="btnInsertCategory" min="-2" pref="59" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="51" max="-2" attributes="0"/>
<Component id="btnEditCategory" min="-2" pref="69" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="57" max="-2" attributes="0"/>
<Component id="btnDeleteCategory" min="-2" pref="68" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="21" max="-2" attributes="0"/>
<Component id="jLabel1" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="34" max="-2" attributes="0"/>
<Component id="txtCategory" min="-2" pref="498" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Component id="jScrollPane1" min="-2" pref="738" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace pref="36" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel1" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="txtCategory" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="19" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="btnEditCategory" pref="33" max="32767" attributes="0"/>
<Component id="btnInsertCategory" alignment="0" max="32767" attributes="0"/>
<Component id="btnDeleteCategory" alignment="0" max="32767" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Component id="jScrollPane1" min="-2" pref="124" max="-2" attributes="0"/>
<EmptySpace max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JLabel" name="jLabel1">
<Properties>
<Property name="text" type="java.lang.String" value="Ten danh muc:"/>
</Properties>
</Component>
<Component class="javax.swing.JTextField" name="txtCategory">
</Component>
<Component class="java.awt.Button" name="btnInsertCategory">
<Properties>
<Property name="label" type="java.lang.String" value="Them"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="btnInsertCategoryActionPerformed"/>
</Events>
</Component>
<Component class="java.awt.Button" name="btnEditCategory">
<Properties>
<Property name="label" type="java.lang.String" value="Sua"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="btnEditCategoryActionPerformed"/>
</Events>
</Component>
<Component class="java.awt.Button" name="btnDeleteCategory">
<Properties>
<Property name="label" type="java.lang.String" value="Xoa"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="btnDeleteCategoryActionPerformed"/>
</Events>
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane1">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTable" name="tblCategory">
<Properties>
<Property name="model" type="javax.swing.table.TableModel" editor="org.netbeans.modules.form.editors2.TableModelEditor">
<Table columnCount="2" rowCount="0">
<Column editable="true" title="STT" type="java.lang.Object"/>
<Column editable="false" title="Ten danh muc" type="java.lang.Object"/>
</Table>
</Property>
<Property name="columnModel" type="javax.swing.table.TableColumnModel" editor="org.netbeans.modules.form.editors2.TableColumnModelEditor">
<TableColumnModel selectionModel="0">
<Column maxWidth="-1" minWidth="-1" prefWidth="-1" resizable="true">
<Title/>
<Editor/>
<Renderer/>
</Column>
<Column maxWidth="-1" minWidth="-1" prefWidth="-1" resizable="true">
<Title/>
<Editor/>
<Renderer/>
</Column>
</TableColumnModel>
</Property>
<Property name="tableHeader" type="javax.swing.table.JTableHeader" editor="org.netbeans.modules.form.editors2.JTableHeaderEditor">
<TableHeader reorderingAllowed="true" resizingAllowed="true"/>
</Property>
</Properties>
</Component>
</SubComponents>
</Container>
</SubComponents>
</Container>
<Container class="javax.swing.JPanel" name="jPanel2">
<Properties>
<Property name="border" type="javax.swing.border.Border" editor="org.netbeans.modules.form.editors2.BorderEditor">
<Border info="org.netbeans.modules.form.compat2.border.TitledBorderInfo">
<TitledBorder title="Nhap thong tin san pham"/>
</Border>
</Property>
</Properties>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<EmptySpace min="-2" pref="26" max="-2" attributes="0"/>
<Group type="103" groupAlignment="1" attributes="0">
<Component id="jLabel5" min="-2" max="-2" attributes="0"/>
<Component id="jLabel4" min="-2" max="-2" attributes="0"/>
<Component id="jLabel3" min="-2" max="-2" attributes="0"/>
<Component id="jLabel2" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="43" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="txtName" max="32767" attributes="0"/>
<Component id="boxCategory" min="-2" max="-2" attributes="0"/>
<Component id="txtPrice" alignment="0" max="32767" attributes="0"/>
<Component id="txtDescription" pref="495" max="32767" attributes="0"/>
</Group>
</Group>
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="217" max="-2" attributes="0"/>
<Component id="btnInsertProduct" min="-2" pref="62" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="33" max="-2" attributes="0"/>
<Component id="btnEditProduct" min="-2" pref="62" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="39" max="-2" attributes="0"/>
<Component id="btnDeleteProduct" min="-2" pref="62" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="40" max="-2" attributes="0"/>
<Component id="btnProductSearch" min="-2" pref="83" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace min="0" pref="129" max="32767" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Component id="jScrollPane2" max="32767" attributes="0"/>
</Group>
</Group>
<EmptySpace max="-2" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel2" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="txtName" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel3" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="boxCategory" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel4" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="txtPrice" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel5" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="txtDescription" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="21" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="btnInsertProduct" min="-2" pref="36" max="-2" attributes="0"/>
<Component id="btnEditProduct" min="-2" pref="36" max="-2" attributes="0"/>
<Component id="btnDeleteProduct" min="-2" pref="36" max="-2" attributes="0"/>
<Component id="btnProductSearch" min="-2" pref="36" max="-2" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Component id="jScrollPane2" min="-2" pref="150" max="-2" attributes="0"/>
<EmptySpace max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JLabel" name="jLabel2">
<Properties>
<Property name="text" type="java.lang.String" value="Ten SP:"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel3">
<Properties>
<Property name="text" type="java.lang.String" value="Danh muc SP:"/>
</Properties>
</Component>
<Component class="javax.swing.JTextField" name="txtName">
</Component>
<Component class="javax.swing.JComboBox" name="boxCategory">
<Properties>
<Property name="model" type="javax.swing.ComboBoxModel" editor="org.netbeans.modules.form.editors2.ComboBoxModelEditor">
<StringArray count="0"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_TypeParameters" type="java.lang.String" value="<String>"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel4">
<Properties>
<Property name="text" type="java.lang.String" value="Gia SP:"/>
</Properties>
</Component>
<Component class="javax.swing.JTextField" name="txtPrice">
</Component>
<Component class="javax.swing.JLabel" name="jLabel5">
<Properties>
<Property name="text" type="java.lang.String" value="Mo ta SP:"/>
</Properties>
</Component>
<Component class="javax.swing.JTextField" name="txtDescription">
</Component>
<Component class="java.awt.Button" name="btnInsertProduct">
<Properties>
<Property name="label" type="java.lang.String" value="Them"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="btnInsertProductActionPerformed"/>
</Events>
</Component>
<Component class="java.awt.Button" name="btnEditProduct">
<Properties>
<Property name="label" type="java.lang.String" value="Sua"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="btnEditProductActionPerformed"/>
</Events>
</Component>
<Component class="java.awt.Button" name="btnDeleteProduct">
<Properties>
<Property name="label" type="java.lang.String" value="Xoa"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="btnDeleteProductActionPerformed"/>
</Events>
</Component>
<Component class="java.awt.Button" name="btnProductSearch">
<Properties>
<Property name="label" type="java.lang.String" value="Tim kiem"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="btnProductSearchActionPerformed"/>
</Events>
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane2">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTable" name="tblProduct">
<Properties>
<Property name="model" type="javax.swing.table.TableModel" editor="org.netbeans.modules.form.editors2.TableModelEditor">
<Table columnCount="5" rowCount="0">
<Column editable="false" title="STT" type="java.lang.Object"/>
<Column editable="false" title="Ten" type="java.lang.Object"/>
<Column editable="false" title="Danh muc" type="java.lang.Object"/>
<Column editable="true" title="Gia" type="java.lang.Object"/>
<Column editable="false" title="Mo ta" type="java.lang.Object"/>
</Table>
</Property>
<Property name="columnModel" type="javax.swing.table.TableColumnModel" editor="org.netbeans.modules.form.editors2.TableColumnModelEditor">
<TableColumnModel selectionModel="0">
<Column maxWidth="-1" minWidth="-1" prefWidth="-1" resizable="true">
<Title/>
<Editor/>
<Renderer/>
</Column>
<Column maxWidth="-1" minWidth="-1" prefWidth="-1" resizable="true">
<Title/>
<Editor/>
<Renderer/>
</Column>
<Column maxWidth="-1" minWidth="-1" prefWidth="-1" resizable="true">
<Title/>
<Editor/>
<Renderer/>
</Column>
<Column maxWidth="-1" minWidth="-1" prefWidth="-1" resizable="true">
<Title/>
<Editor/>
<Renderer/>
</Column>
<Column maxWidth="-1" minWidth="-1" prefWidth="-1" resizable="true">
<Title/>
<Editor/>
<Renderer/>
</Column>
</TableColumnModel>
</Property>
<Property name="tableHeader" type="javax.swing.table.JTableHeader" editor="org.netbeans.modules.form.editors2.JTableHeaderEditor">
<TableHeader reorderingAllowed="true" resizingAllowed="true"/>
</Property>
</Properties>
</Component>
</SubComponents>
</Container>
</SubComponents>
</Container>
</SubComponents>
</Form>
#ProductFrame.java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package Java2.lesson10;
import com.google.gson.Gson;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.JOptionPane;
import javax.swing.table.DefaultTableModel;
/**
*
* @author MyPC
*/
public class ProductFrame extends javax.swing.JFrame {
DefaultTableModel tblModel;
DefaultTableModel tblModel1;
List<Category> categoryList = new ArrayList<>();
List<Product> productList = new ArrayList<>();
int index = -1;
int index1 = -1;
/**
* Creates new form ProductFrame
*/
public ProductFrame() {
initComponents();
tblModel = (DefaultTableModel) tblCategory.getModel();
tblModel1 = (DefaultTableModel) tblProduct.getModel();
tblCategory.addMouseListener(new MouseListener() {
@Override
public void mouseClicked(MouseEvent e) {
index = tblCategory.getSelectedRow();
txtCategory.setText(categoryList.get(index).getCategoryName());
}
@Override
public void mousePressed(MouseEvent e) {
}
@Override
public void mouseReleased(MouseEvent e) {
}
@Override
public void mouseEntered(MouseEvent e) {
}
@Override
public void mouseExited(MouseEvent e) {
}
});
tblProduct.addMouseListener(new MouseListener() {
@Override
public void mouseClicked(MouseEvent e) {
index1 = tblProduct.getSelectedRow();
txtName.setText(productList.get(index1).getName());
boxCategory.setSelectedItem(productList.get(index1).getCategoryName());
txtPrice.setText(String.valueOf(productList.get(index1).getPrice()));
txtDescription.setText(productList.get(index1).getDescription());
}
@Override
public void mousePressed(MouseEvent e) {
}
@Override
public void mouseReleased(MouseEvent e) {
}
@Override
public void mouseEntered(MouseEvent e) {
}
@Override
public void mouseExited(MouseEvent e) {
}
});
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
jPanel1 = new javax.swing.JPanel();
jLabel1 = new javax.swing.JLabel();
txtCategory = new javax.swing.JTextField();
btnInsertCategory = new java.awt.Button();
btnEditCategory = new java.awt.Button();
btnDeleteCategory = new java.awt.Button();
jScrollPane1 = new javax.swing.JScrollPane();
tblCategory = new javax.swing.JTable();
jPanel2 = new javax.swing.JPanel();
jLabel2 = new javax.swing.JLabel();
jLabel3 = new javax.swing.JLabel();
txtName = new javax.swing.JTextField();
boxCategory = new javax.swing.JComboBox<>();
jLabel4 = new javax.swing.JLabel();
txtPrice = new javax.swing.JTextField();
jLabel5 = new javax.swing.JLabel();
txtDescription = new javax.swing.JTextField();
btnInsertProduct = new java.awt.Button();
btnEditProduct = new java.awt.Button();
btnDeleteProduct = new java.awt.Button();
btnProductSearch = new java.awt.Button();
jScrollPane2 = new javax.swing.JScrollPane();
tblProduct = new javax.swing.JTable();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jPanel1.setBorder(javax.swing.BorderFactory.createTitledBorder("Nhap thong tin"));
jLabel1.setText("Ten danh muc:");
btnInsertCategory.setLabel("Them");
btnInsertCategory.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnInsertCategoryActionPerformed(evt);
}
});
btnEditCategory.setLabel("Sua");
btnEditCategory.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnEditCategoryActionPerformed(evt);
}
});
btnDeleteCategory.setLabel("Xoa");
btnDeleteCategory.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnDeleteCategoryActionPerformed(evt);
}
});
tblCategory.setModel(new javax.swing.table.DefaultTableModel(
new Object [][] {
},
new String [] {
"STT", "Ten danh muc"
}
) {
boolean[] canEdit = new boolean [] {
true, false
};
public boolean isCellEditable(int rowIndex, int columnIndex) {
return canEdit [columnIndex];
}
});
jScrollPane1.setViewportView(tblCategory);
javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1);
jPanel1.setLayout(jPanel1Layout);
jPanel1Layout.setHorizontalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addGap(216, 216, 216)
.addComponent(btnInsertCategory, javax.swing.GroupLayout.PREFERRED_SIZE, 59, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(51, 51, 51)
.addComponent(btnEditCategory, javax.swing.GroupLayout.PREFERRED_SIZE, 69, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(57, 57, 57)
.addComponent(btnDeleteCategory, javax.swing.GroupLayout.PREFERRED_SIZE, 68, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(jPanel1Layout.createSequentialGroup()
.addGap(21, 21, 21)
.addComponent(jLabel1)
.addGap(34, 34, 34)
.addComponent(txtCategory, javax.swing.GroupLayout.PREFERRED_SIZE, 498, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(jPanel1Layout.createSequentialGroup()
.addContainerGap()
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 738, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addContainerGap(36, Short.MAX_VALUE))
);
jPanel1Layout.setVerticalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel1)
.addComponent(txtCategory, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(19, 19, 19)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(btnEditCategory, javax.swing.GroupLayout.DEFAULT_SIZE, 33, Short.MAX_VALUE)
.addComponent(btnInsertCategory, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(btnDeleteCategory, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 124, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
jPanel2.setBorder(javax.swing.BorderFactory.createTitledBorder("Nhap thong tin san pham"));
jLabel2.setText("Ten SP:");
jLabel3.setText("Danh muc SP:");
jLabel4.setText("Gia SP:");
jLabel5.setText("Mo ta SP:");
btnInsertProduct.setLabel("Them");
btnInsertProduct.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnInsertProductActionPerformed(evt);
}
});
btnEditProduct.setLabel("Sua");
btnEditProduct.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnEditProductActionPerformed(evt);
}
});
btnDeleteProduct.setLabel("Xoa");
btnDeleteProduct.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnDeleteProductActionPerformed(evt);
}
});
btnProductSearch.setLabel("Tim kiem");
btnProductSearch.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnProductSearchActionPerformed(evt);
}
});
tblProduct.setModel(new javax.swing.table.DefaultTableModel(
new Object [][] {
},
new String [] {
"STT", "Ten", "Danh muc", "Gia", "Mo ta"
}
) {
boolean[] canEdit = new boolean [] {
false, false, false, true, false
};
public boolean isCellEditable(int rowIndex, int columnIndex) {
return canEdit [columnIndex];
}
});
jScrollPane2.setViewportView(tblProduct);
javax.swing.GroupLayout jPanel2Layout = new javax.swing.GroupLayout(jPanel2);
jPanel2.setLayout(jPanel2Layout);
jPanel2Layout.setHorizontalGroup(
jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel2Layout.createSequentialGroup()
.addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel2Layout.createSequentialGroup()
.addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel2Layout.createSequentialGroup()
.addGap(26, 26, 26)
.addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(jLabel5)
.addComponent(jLabel4)
.addComponent(jLabel3)
.addComponent(jLabel2))
.addGap(43, 43, 43)
.addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(txtName)
.addComponent(boxCategory, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(txtPrice)
.addComponent(txtDescription, javax.swing.GroupLayout.DEFAULT_SIZE, 495, Short.MAX_VALUE)))
.addGroup(jPanel2Layout.createSequentialGroup()
.addGap(217, 217, 217)
.addComponent(btnInsertProduct, javax.swing.GroupLayout.PREFERRED_SIZE, 62, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(33, 33, 33)
.addComponent(btnEditProduct, javax.swing.GroupLayout.PREFERRED_SIZE, 62, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(39, 39, 39)
.addComponent(btnDeleteProduct, javax.swing.GroupLayout.PREFERRED_SIZE, 62, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(40, 40, 40)
.addComponent(btnProductSearch, javax.swing.GroupLayout.PREFERRED_SIZE, 83, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addGap(0, 129, Short.MAX_VALUE))
.addGroup(jPanel2Layout.createSequentialGroup()
.addContainerGap()
.addComponent(jScrollPane2)))
.addContainerGap())
);
jPanel2Layout.setVerticalGroup(
jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel2Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel2)
.addComponent(txtName, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel3)
.addComponent(boxCategory, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel4)
.addComponent(txtPrice, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel5)
.addComponent(txtDescription, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(21, 21, 21)
.addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(btnInsertProduct, javax.swing.GroupLayout.PREFERRED_SIZE, 36, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(btnEditProduct, javax.swing.GroupLayout.PREFERRED_SIZE, 36, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(btnDeleteProduct, javax.swing.GroupLayout.PREFERRED_SIZE, 36, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(btnProductSearch, javax.swing.GroupLayout.PREFERRED_SIZE, 36, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jScrollPane2, javax.swing.GroupLayout.PREFERRED_SIZE, 150, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jPanel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jPanel2, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(jPanel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(jPanel2, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
pack();
}// </editor-fold>//GEN-END:initComponents
private void btnInsertCategoryActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnInsertCategoryActionPerformed
// TODO add your handling code here:
Category category = new Category(txtCategory.getText());
categoryList.add(category);
boxCategory.addItem(category.getCategoryName());
txtCategory.setText("");
tblModel.setRowCount(0);
for (Category category1 : categoryList) {
tblModel.addRow(new Object[]{tblModel.getRowCount() + 1, category1.getCategoryName()});
}
saveXML();
}//GEN-LAST:event_btnInsertCategoryActionPerformed
private void saveXML() {
FileOutputStream fos = null;
try {
fos = new FileOutputStream("category.xml");
String categoryList1 = "";
for (Category category : categoryList) {
categoryList1 += category.toXML();
}
String content = toXML(categoryList1);
byte[] b = content.getBytes();
fos.write(b);
} catch (FileNotFoundException ex) {
Logger.getLogger(ProductFrame.class.getName()).log(Level.SEVERE, null, ex);
} catch (IOException ex) {
Logger.getLogger(ProductFrame.class.getName()).log(Level.SEVERE, null, ex);
}finally{
if(fos != null){
try {
fos.close();
} catch (IOException ex) {
Logger.getLogger(ProductFrame.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
}
private String toXML(String categoryList) {
return "<?xml version=\"1.0\" encoding=\"UTF-8\"?>\n"
+ "\n"
+ "<category-list>\n"
+ categoryList
+ "</category-list>";
}
private void btnDeleteCategoryActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnDeleteCategoryActionPerformed
// TODO add your handling code here:
if (index < 0) {
JOptionPane.showMessageDialog(rootPane, "Vui long lua chon danh muc muon xoa!!");
} else {
categoryList.remove(index);
tblModel.setRowCount(0);
for (Category category1 : categoryList) {
tblModel.addRow(new Object[]{tblModel.getRowCount() + 1, category1.getCategoryName()});
}
txtCategory.setText("");
index = -1;
}
saveXML();
}//GEN-LAST:event_btnDeleteCategoryActionPerformed
private void btnEditCategoryActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnEditCategoryActionPerformed
// TODO add your handling code here:
if (index < 0) {
JOptionPane.showMessageDialog(rootPane, "Vui long lua chon danh muc muon sua!!");
} else {
categoryList.get(index).setCategoryName(txtCategory.getText());
tblModel.setRowCount(0);
for (Category category1 : categoryList) {
tblModel.addRow(new Object[]{tblModel.getRowCount() + 1, category1.getCategoryName()});
}
txtCategory.setText("");
index = -1;
}
saveXML();
}//GEN-LAST:event_btnEditCategoryActionPerformed
private void btnInsertProductActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnInsertProductActionPerformed
// TODO add your handling code here:
Product product = new Product(txtName.getText(), String.valueOf(boxCategory.getSelectedItem()),
txtDescription.getText(), Float.parseFloat(txtPrice.getText()));
productList.add(product);
txtName.setText("");
boxCategory.setSelectedIndex(0);
txtPrice.setText("");
txtDescription.setText("");
tblModel1.setRowCount(0);
for (Product product1 : productList) {
tblModel1.addRow(new Object[]{tblModel1.getRowCount() + 1, product1.getName(),
product1.getCategoryName(), product1.getPrice(), product1.getDescription()});
}
saveJSON();
}//GEN-LAST:event_btnInsertProductActionPerformed
private void saveJSON(){
Gson gson = new Gson();
String json = gson.toJson(productList);
FileOutputStream fos = null;
try {
fos = new FileOutputStream("products.json");
byte[] b = json.getBytes();
fos.write(b);
} catch (FileNotFoundException ex) {
Logger.getLogger(ProductFrame.class.getName()).log(Level.SEVERE, null, ex);
} catch (IOException ex) {
Logger.getLogger(ProductFrame.class.getName()).log(Level.SEVERE, null, ex);
}finally{
if(fos != null){
try {
fos.close();
} catch (IOException ex) {
Logger.getLogger(ProductFrame.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
}
private void btnEditProductActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnEditProductActionPerformed
// TODO add your handling code here:
if (index1 < 0) {
JOptionPane.showMessageDialog(rootPane, "Vui long lua chon san pham muon sua!!");
} else {
productList.get(index1).setName(txtName.getText());
productList.get(index1).setCategoryName(String.valueOf(boxCategory.getSelectedItem()));
productList.get(index1).setPrice(Float.parseFloat(txtPrice.getText()));
productList.get(index1).setDescription(txtDescription.getText());
tblModel1.setRowCount(0);
for (Product product1 : productList) {
tblModel1.addRow(new Object[]{tblModel1.getRowCount() + 1, product1.getName(),
product1.getCategoryName(), product1.getPrice(), product1.getDescription()});
}
txtName.setText("");
boxCategory.setSelectedIndex(0);
txtPrice.setText("");
txtDescription.setText("");
index1 = -1;
}
saveJSON();
}//GEN-LAST:event_btnEditProductActionPerformed
private void btnDeleteProductActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnDeleteProductActionPerformed
// TODO add your handling code here:
if (index1 < 0) {
JOptionPane.showMessageDialog(rootPane, "Vui long lua chon san pham muon xoa!!");
} else {
productList.remove(index1);
tblModel1.setRowCount(0);
for (Product product1 : productList) {
tblModel1.addRow(new Object[]{tblModel1.getRowCount() + 1, product1.getName(),
product1.getCategoryName(), product1.getPrice(), product1.getDescription()});
}
txtName.setText("");
boxCategory.setSelectedIndex(0);
txtPrice.setText("");
txtDescription.setText("");
index1 = -1;
}
saveJSON();
}//GEN-LAST:event_btnDeleteProductActionPerformed
private void btnProductSearchActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnProductSearchActionPerformed
// TODO add your handling code here:
String nameSearch = JOptionPane.showInputDialog("Ten san pham muon tim kiem:");
try {
if (nameSearch.isEmpty()) {
tblModel1.setRowCount(0);
for (Product product1 : productList) {
tblModel1.addRow(new Object[]{tblModel1.getRowCount() + 1, product1.getName(),
product1.getCategoryName(), product1.getPrice(), product1.getDescription()});
}
}
} catch (Exception e) {
return;
}
tblModel1.setRowCount(0);
int check = 0;
for (Product product1 : productList) {
if (product1.getName().equalsIgnoreCase(nameSearch)) {
tblModel1.addRow(new Object[]{tblModel1.getRowCount() + 1, product1.getName(),
product1.getCategoryName(), product1.getPrice(), product1.getDescription()});
check++;
}
}
if (check == 0) {
JOptionPane.showMessageDialog(rootPane, "Khong tim thay san pham can tim kiem!!");
tblModel1.setRowCount(0);
for (Product product1 : productList) {
tblModel1.addRow(new Object[]{tblModel1.getRowCount() + 1, product1.getName(),
product1.getCategoryName(), product1.getPrice(), product1.getDescription()});
}
}
}//GEN-LAST:event_btnProductSearchActionPerformed
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(ProductFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(ProductFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(ProductFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(ProductFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new ProductFrame().setVisible(true);
}
});
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JComboBox<String> boxCategory;
private java.awt.Button btnDeleteCategory;
private java.awt.Button btnDeleteProduct;
private java.awt.Button btnEditCategory;
private java.awt.Button btnEditProduct;
private java.awt.Button btnInsertCategory;
private java.awt.Button btnInsertProduct;
private java.awt.Button btnProductSearch;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel4;
private javax.swing.JLabel jLabel5;
private javax.swing.JPanel jPanel1;
private javax.swing.JPanel jPanel2;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JScrollPane jScrollPane2;
private javax.swing.JTable tblCategory;
private javax.swing.JTable tblProduct;
private javax.swing.JTextField txtCategory;
private javax.swing.JTextField txtDescription;
private javax.swing.JTextField txtName;
private javax.swing.JTextField txtPrice;
// End of variables declaration//GEN-END:variables
}