By GokiSoft.com|
17:22 29/10/2021|
Java Advanced
[Examination] Kiểm tra 60 phút - Đề 3
Thiết kế CSDL đặt tên là : quanlysinhvien
Tạo 1 bảng students gồm các thuộc tính : rollno, fullname, gender, email, address
Nhập vào bảng trên ít nhất 3 sinh viên bất kỳ.
Thiết kế phần mềm có giao diện như sau
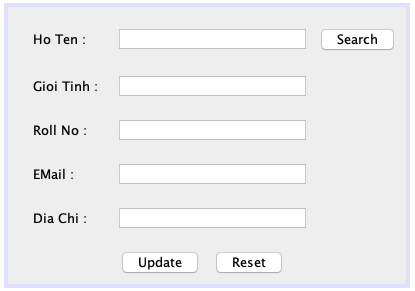
Khi người dùng nhấn vào button search thực hiện task vụ sau
- Nếu RollNo : để trống thì hiển thị message yêu cầu nhập rollno cần tìm kiếm
- Nếu RollNo : khác rỗng -> thực hiện tìm kiếm sinh viên trong csdl và hiển thị ra các trường trong form
Khi người dùng click reset thì xoá toàn bộ dữ liệu sv trong form
Khi người dùng click vào update thì cập nhật dữ liệu trong CSDL và xoá tất cả các dữ liệu trong form.
Tags:
Phản hồi từ học viên
5
(Dựa trên đánh giá ngày hôm nay)
![Trương Công Vinh [T1907A]](https://www.gravatar.com/avatar/223a7e3a46f4a747f81b921fe023fcc4.jpg?s=80&d=mm&r=g)
Trương Công Vinh
2020-04-08 10:12:29
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package L9;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.JOptionPane;
/**
*
* @author DELL
*/
public class studentFrame extends javax.swing.JFrame {
ArrayList<Student> list = new ArrayList();
studentModify stdModify ;
/**
* Creates new form studentFrame
*/
public studentFrame() {
initComponents();
}
public void reset(){
txthoten.setText("");
txtgioitinh.setText("");
txtrollno.setText("");
txtemail.setText("");
txtdiachi.setText("");
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
jLabel1 = new javax.swing.JLabel();
txthoten = new javax.swing.JTextField();
jLabel2 = new javax.swing.JLabel();
txtgioitinh = new javax.swing.JTextField();
jLabel3 = new javax.swing.JLabel();
txtrollno = new javax.swing.JTextField();
jLabel6 = new javax.swing.JLabel();
txtemail = new javax.swing.JTextField();
jLabel7 = new javax.swing.JLabel();
txtdiachi = new javax.swing.JTextField();
btnsearch = new javax.swing.JButton();
btnreset = new javax.swing.JButton();
btnupdate = new javax.swing.JButton();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jLabel1.setText("Ho Ten : ");
jLabel2.setText("Gioi Tinh :");
jLabel3.setText("Roll No :");
txtrollno.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
txtrollnoActionPerformed(evt);
}
});
jLabel6.setText("Email :");
jLabel7.setText("Dia Chi");
btnsearch.setText("Search");
btnsearch.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnsearchActionPerformed(evt);
}
});
btnreset.setText("Reset");
btnreset.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnresetActionPerformed(evt);
}
});
btnupdate.setText("Update");
btnupdate.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnupdateActionPerformed(evt);
}
});
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(46, 46, 46)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel6, javax.swing.GroupLayout.PREFERRED_SIZE, 69, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(32, 32, 32)
.addComponent(txtemail, javax.swing.GroupLayout.PREFERRED_SIZE, 330, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel3, javax.swing.GroupLayout.PREFERRED_SIZE, 69, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(32, 32, 32)
.addComponent(txtrollno, javax.swing.GroupLayout.PREFERRED_SIZE, 330, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel2, javax.swing.GroupLayout.PREFERRED_SIZE, 69, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(32, 32, 32)
.addComponent(txtgioitinh, javax.swing.GroupLayout.PREFERRED_SIZE, 330, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 69, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(32, 32, 32)
.addComponent(txthoten, javax.swing.GroupLayout.PREFERRED_SIZE, 330, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(50, 50, 50)
.addComponent(btnsearch))
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel7, javax.swing.GroupLayout.PREFERRED_SIZE, 69, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(32, 32, 32)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addGroup(layout.createSequentialGroup()
.addComponent(btnreset)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(btnupdate))
.addComponent(txtdiachi, javax.swing.GroupLayout.PREFERRED_SIZE, 330, javax.swing.GroupLayout.PREFERRED_SIZE))))
.addContainerGap(41, Short.MAX_VALUE))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(65, 65, 65)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 27, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(txthoten, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(btnsearch))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel2, javax.swing.GroupLayout.PREFERRED_SIZE, 27, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(txtgioitinh, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel3, javax.swing.GroupLayout.PREFERRED_SIZE, 27, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(txtrollno, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel6, javax.swing.GroupLayout.PREFERRED_SIZE, 27, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(txtemail, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel7, javax.swing.GroupLayout.PREFERRED_SIZE, 27, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(txtdiachi, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(btnreset)
.addComponent(btnupdate))
.addContainerGap(28, Short.MAX_VALUE))
);
pack();
}// </editor-fold>
private void txtrollnoActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
}
private void btnresetActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
reset();
}
private void btnupdateActionPerformed(java.awt.event.ActionEvent evt) {
Connection connection = null;
PreparedStatement statement = null;
list = stdModify.getList();
String rollno = txtrollno.getText();;
for (int i = 0; i < list.size(); i++) {
if (list.get(i).getRollno().contains(rollno)) {
txtrollno.setText("Roll no ton tai --> nhap lai !!!");
}else{
try {
// TODO add your handling code here:
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/studentL9?serverTimezone=UTC","root","");
String sql = "insert into student(rollno,hoten,gioitinh,email,diachi) values(?,?,?,?,?)";
statement = connection.prepareCall(sql);
statement.setString(1,rollno);
statement.setString(2, txthoten.getText());
statement.setString(3, txtgioitinh.getText());
statement.setString(4, txtemail.getText());
statement.setString(5, txtdiachi.getText());
statement.execute();
} catch (SQLException ex) {
Logger.getLogger(studentFrame.class.getName()).log(Level.SEVERE, null, ex);
}finally{
if (statement!=null) {
try {
statement.close();
} catch (SQLException ex) {
Logger.getLogger(studentFrame.class.getName()).log(Level.SEVERE, null, ex);
}
}
if (connection != null ) {
try {
connection.close();
} catch (SQLException ex) {
Logger.getLogger(studentFrame.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
reset();
}
}
}
private void btnsearchActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
String input = JOptionPane.showInputDialog(this, "Enter rollno to search : ");
list = stdModify.getList();
if (input != null && input.length() > 0) {
for (int i = 0; i < list.size(); i++) {
if (input.equals(list.get(i).getRollno())) {
txtdiachi.setText(list.get(i).getDiachi());
txtemail.setText(list.get(i).getEmail());
txtgioitinh.setText(list.get(i).getGioitinh());
txthoten.setText(list.get(i).getHoten());
txtrollno.setText(list.get(i).getRollno());
}else{
txtrollno.setText("khong ton tai rollno !!!");
}
}
}
}
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(studentFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(studentFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(studentFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(studentFrame.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new studentFrame().setVisible(true);
}
});
}
// Variables declaration - do not modify
private javax.swing.JButton btnreset;
private javax.swing.JButton btnsearch;
private javax.swing.JButton btnupdate;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel6;
private javax.swing.JLabel jLabel7;
private javax.swing.JTextField txtdiachi;
private javax.swing.JTextField txtemail;
private javax.swing.JTextField txtgioitinh;
private javax.swing.JTextField txthoten;
private javax.swing.JTextField txtrollno;
// End of variables declaration
}
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package L9;
/**
*
* @author DELL
*/
public class Student {
String rollno,hoten,gioitinh,email,diachi;
public Student() {
}
public Student(String rollno, String hoten, String gioitinh, String email, String diachi) {
this.rollno = rollno;
this.hoten = hoten;
this.gioitinh = gioitinh;
this.email = email;
this.diachi = diachi;
}
public String getRollno() {
return rollno;
}
public void setRollno(String rollno) {
this.rollno = rollno;
}
public String getHoten() {
return hoten;
}
public void setHoten(String hoten) {
this.hoten = hoten;
}
public String getGioitinh() {
return gioitinh;
}
public void setGioitinh(String gioitinh) {
this.gioitinh = gioitinh;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getDiachi() {
return diachi;
}
public void setDiachi(String diachi) {
this.diachi = diachi;
}
}
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package L9;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
*
* @author DELL
*/
public class studentModify {
public static ArrayList<Student> getList() {
ArrayList<Student> list = new ArrayList<>();
Connection connection = null;
Statement statement = null;
try {
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/studentL9?serverTimezone=UTC","root","");
statement = connection.createStatement();
String sql = "Select * from student ";
ResultSet resultset = statement.executeQuery(sql);
while (resultset.next()) {
Student std = new Student(resultset.getString("hoten"),
resultset.getString("gioitinh"),
resultset.getString("rollno"),
resultset.getString("email"),
resultset.getString("diachi"));
list.add(std);
}
} catch (SQLException ex) {
Logger.getLogger(studentModify.class.getName()).log(Level.SEVERE, null, ex);
}finally{
if (statement!=null) {
try {
statement.close();
} catch (SQLException ex) {
Logger.getLogger(studentFrame.class.getName()).log(Level.SEVERE, null, ex);
}
}
if (connection != null ) {
try {
connection.close();
} catch (SQLException ex) {
Logger.getLogger(studentFrame.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
return list;
}
/*public static Student search(String rollno){
Student std = new Student();
return std;
}*/
}
![NguyenHuuThanh [T1907A]](https://www.gravatar.com/avatar/035e4f4fed661b8e1c3e066e43cd5e41.jpg?s=80&d=mm&r=g)
NguyenHuuThanh
2020-04-08 10:02:14
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package javaswingjava05;
import java.beans.Statement;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
*
* @author abc
*/
public class Studentmodify {
public static List<Student> findAll() {
List<Student> studentlist = new ArrayList<>();
Connection connection = null;
// an instruction that explains what should happen
java.sql.Statement statement = null;
try {
// Ket noi toi database : jdbc : mysql://localhost:3306/ ten ; tai khoan + mat khau
//muc dich lay danh sach tat ca sinh vien :
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/student04?serverTimezone=UTC", "root", "");
//Query
String sql = "select * from student";
// Creates a Statement object for sending SQL statements to the database
statement = connection.createStatement();
// A table of data representing a database result set, which is usually generated by executing a statement that queries the database.
ResultSet resultSet = statement.executeQuery(sql);
// reselutSet.next() == moves the pointer of the current (ResultSet) object to the next row, from the current position
while (resultSet.next()) {
// Create a student getting from resultSet ( database )
Student std = new Student(
resultSet.getInt("id"),
resultSet.getString("fullname"),
resultSet.getString("gender"),
resultSet.getString("rollno"),
resultSet.getString("email"),
resultSet.getString("address"));
studentlist.add(std);
}
} catch (SQLException ex) {
Logger.getLogger(Studentmodify.class.getName()).log(Level.SEVERE, null, ex);
} finally {
if (statement != null) {
try {
statement.close();
} catch (SQLException ex) {
Logger.getLogger(Studentmodify.class.getName()).log(Level.SEVERE, null, ex);
}
}
// neu connection da chay , dong connection.
if (connection != null) {
try {
connection.close();
} catch (SQLException ex) {
Logger.getLogger(Studentmodify.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
return studentlist;
}
public static void update(Student std) {
Connection connection = null;
// for parametirized query
PreparedStatement statement = null;
try {
// Ket noi toi database : jdbc : mysql://localhost:3306/ ten ; tai khoan + mat khau
//muc dich lay danh sach tat ca sinh vien :
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/student04?serverTimezone=UTC", "root", "");
//Query
String sql = "update student set fullname = ?, gender = ? , rollno = ? , email = ? , address = ? where id = ?";
//
statement = connection.prepareCall(sql);
statement.setString(1, std.getFullname());
statement.setString(2, std.getGender());
statement.setString(3, std.getRollno());
statement.setString(4, std.getEmail());
statement.setString(5, std.getAddress());
statement.setInt(6, std.getId());
statement.execute();
} catch (SQLException ex) {
Logger.getLogger(Studentmodify.class.getName()).log(Level.SEVERE, null, ex);
} finally {
if (statement != null) {
try {
statement.close();
} catch (SQLException ex) {
Logger.getLogger(Studentmodify.class.getName()).log(Level.SEVERE, null, ex);
}
}
// neu connection da chay , dong connection.
if (connection != null) {
try {
connection.close();
} catch (SQLException ex) {
Logger.getLogger(Studentmodify.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
}
public static List<Student> findByRollno( String rollno ) {
List<Student> studentlist = new ArrayList<>();
Connection connection = null;
// an instruction that explains what should happen
PreparedStatement statement = null;
try {
// Ket noi toi database : jdbc : mysql://localhost:3306/ ten ; tai khoan + mat khau
//muc dich lay danh sach tat ca sinh vien :
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/student04?serverTimezone=UTC", "root", "");
//Query
String sql = "select * from student WHERE rollno = ?";
// Creates a Statement object for sending SQL statements to the database
statement = connection.prepareCall(sql);
statement.setString(1, rollno);
// A table of data representing a database result set, which is usually generated by executing a statement that queries the database.
ResultSet resultSet = statement.executeQuery(sql);
// reselutSet.next() == moves the pointer of the current (ResultSet) object to the next row, from the current position
while (resultSet.next()) {
// Create a student getting from resultSet ( database )
Student std = new Student(resultSet.getInt("id"),
resultSet.getString("fullname"),
resultSet.getString("gender"),
resultSet.getString("rollno"),
resultSet.getString("email"),
resultSet.getString("address"));
studentlist.add(std);
}
} catch (SQLException ex) {
Logger.getLogger(Studentmodify.class.getName()).log(Level.SEVERE, null, ex);
} finally {
if (statement != null) {
try {
statement.close();
} catch (SQLException ex) {
Logger.getLogger(Studentmodify.class.getName()).log(Level.SEVERE, null, ex);
}
}
// neu connection da chay , dong connection.
if (connection != null) {
try {
connection.close();
} catch (SQLException ex) {
Logger.getLogger(Studentmodify.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
return studentlist;
}
public static void insert(Student std) {
Connection connection = null;
// for parametirized query
PreparedStatement statement = null;
try {
// Ket noi toi database : jdbc : mysql://localhost:3306/ ten ; tai khoan + mat khau
//muc dich lay danh sach tat ca sinh vien :
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/student04?serverTimezone=UTC", "root", "");
//Query
String sql = "insert into student(fullname , gender , rollno , email , address) values(?,?,?,?,?)";
//
statement = connection.prepareCall(sql);
statement.setString(1, std.getFullname());
statement.setString(2, std.getGender());
statement.setString(3, std.getRollno());
statement.setString(4, std.getEmail());
statement.setString(5, std.getAddress());
statement.execute();
} catch (SQLException ex) {
Logger.getLogger(Studentmodify.class.getName()).log(Level.SEVERE, null, ex);
} finally {
if (statement != null) {
try {
statement.close();
} catch (SQLException ex) {
Logger.getLogger(Studentmodify.class.getName()).log(Level.SEVERE, null, ex);
}
}
// neu connection da chay , dong connection.
if (connection != null) {
try {
connection.close();
} catch (SQLException ex) {
Logger.getLogger(Studentmodify.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
}
}
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package javaswingjava05;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JOptionPane;
/**
*
* @author abc
*/
public class quanlysinhvien extends javax.swing.JFrame {
List<Student> studentlist = new ArrayList<>();
/**
* Creates new form quanlysinhvien
*/
public quanlysinhvien() {
initComponents();
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
jPanel1 = new javax.swing.JPanel();
txtFullname = new javax.swing.JLabel();
txtGender = new javax.swing.JLabel();
txtRollno = new javax.swing.JLabel();
txtEmail = new javax.swing.JLabel();
txtAddress = new javax.swing.JLabel();
txtFFullname = new javax.swing.JTextField();
txtFGender = new javax.swing.JTextField();
txtFRollno = new javax.swing.JTextField();
txtFEmail = new javax.swing.JTextField();
txtFAddress = new javax.swing.JTextField();
btnSearch = new javax.swing.JButton();
btnUpdate = new javax.swing.JButton();
btnReset = new javax.swing.JButton();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
txtFullname.setText("Name");
txtGender.setText("Gender");
txtRollno.setText("Roll no");
txtEmail.setText("Email");
txtAddress.setText("Address");
btnSearch.setText("Search");
btnSearch.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnSearchActionPerformed(evt);
}
});
btnUpdate.setText("Update");
btnUpdate.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnUpdateActionPerformed(evt);
}
});
btnReset.setText("Reset");
btnReset.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnResetActionPerformed(evt);
}
});
javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1);
jPanel1.setLayout(jPanel1Layout);
jPanel1Layout.setHorizontalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(txtFullname)
.addComponent(txtGender)
.addComponent(txtRollno)
.addComponent(txtEmail)
.addComponent(txtAddress))
.addGap(60, 60, 60)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING, false)
.addComponent(txtFEmail, javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(txtFRollno, javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(txtFGender, javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(txtFFullname, javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(txtFAddress, javax.swing.GroupLayout.DEFAULT_SIZE, 177, Short.MAX_VALUE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(btnSearch)
.addContainerGap())
.addGroup(jPanel1Layout.createSequentialGroup()
.addGap(52, 52, 52)
.addComponent(btnUpdate)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 130, Short.MAX_VALUE)
.addComponent(btnReset)
.addGap(90, 90, 90))
);
jPanel1Layout.setVerticalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addGap(22, 22, 22)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(txtFullname)
.addComponent(txtFFullname, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(btnSearch))
.addGap(35, 35, 35)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(txtGender)
.addComponent(txtFGender, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(44, 44, 44)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(txtRollno)
.addComponent(txtFRollno, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(38, 38, 38)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(txtEmail)
.addComponent(txtFEmail, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(36, 36, 36)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(txtAddress)
.addComponent(txtFAddress, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 45, Short.MAX_VALUE)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(btnUpdate)
.addComponent(btnReset))
.addGap(41, 41, 41))
);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jPanel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(jPanel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(0, 130, Short.MAX_VALUE))
);
pack();
}// </editor-fold>
private void btnResetActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
txtFFullname.setText("");
txtFGender.setText("");
txtFRollno.setText("");
txtFEmail.setText("");
txtFAddress.setText("");
}
private void btnSearchActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
String input = JOptionPane.showInputDialog(this, "Enter rollno");
if (input.length() > 0)
{
studentlist = Studentmodify.findByRollno(input);
for ( Student student : studentlist)
{
txtFFullname.setText(student.getFullname());
txtFGender.setText(student.getGender());
txtFRollno.setText(student.getRollno());
txtFEmail.setText(student.getEmail());
txtFAddress.setText(student.getAddress());
}
}
}
private void btnUpdateActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
String fullname = txtFFullname.getText();
String gender = txtFGender.getText();
String rollno = txtFRollno.getText();
String email = txtFEmail.getText();
String address = txtFAddress.getText();
Student std = new Student(fullname, gender, rollno , email , address );
Studentmodify.insert(std);
txtFFullname.setText("");
txtFGender.setText("");
txtFRollno.setText("");
txtFEmail.setText("");
txtFAddress.setText("");
}
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(quanlysinhvien.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(quanlysinhvien.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(quanlysinhvien.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(quanlysinhvien.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new quanlysinhvien().setVisible(true);
}
});
}
// Variables declaration - do not modify
private javax.swing.JButton btnReset;
private javax.swing.JButton btnSearch;
private javax.swing.JButton btnUpdate;
private javax.swing.JPanel jPanel1;
private javax.swing.JLabel txtAddress;
private javax.swing.JLabel txtEmail;
private javax.swing.JTextField txtFAddress;
private javax.swing.JTextField txtFEmail;
private javax.swing.JTextField txtFFullname;
private javax.swing.JTextField txtFGender;
private javax.swing.JTextField txtFRollno;
private javax.swing.JLabel txtFullname;
private javax.swing.JLabel txtGender;
private javax.swing.JLabel txtRollno;
// End of variables declaration
}
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package javaswingjava05;
/**
*
* @author abc
*/
public class Student {
int id ;
String fullname ,gender , rollno , email , address;
public Student()
{
}
public Student(int id, String fullname, String gender, String rollno, String email, String address) {
this.id = id;
this.fullname = fullname;
this.gender = gender;
this.rollno = rollno;
this.email = email;
this.address = address;
}
public Student(String fullname, String gender, String rollno, String email, String address) {
this.fullname = fullname;
this.gender = gender;
this.rollno = rollno;
this.email = email;
this.address = address;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getFullname() {
return fullname;
}
public void setFullname(String fullname) {
this.fullname = fullname;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
public String getRollno() {
return rollno;
}
public void setRollno(String rollno) {
this.rollno = rollno;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
}