By GokiSoft.com|
19:51 17/06/2024|
Java Advanced
Phần mềm quản lý sinh viên MySQL + Java - Chương trình quản lý sinh viên MySQL + Java - Lập Trình Java
Thiết kế CSDL đặt tên là : quanlysinhvien
Tạo 1 bảng students gồm các thuộc tính : rollno, fullname, gender, email, address
Nhập vào bảng trên ít nhất 3 sinh viên bất kỳ.
Thiết kế phần mềm có giao diện như sau
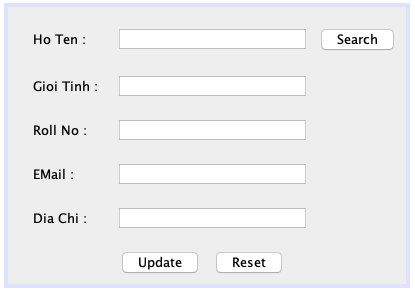
- Nếu RollNo : để trống thì hiển thị message yêu cầu nhập rollno cần tìm kiếm
- Nếu RollNo : khác rỗng -> thực hiện tìm kiếm sinh viên trong csdl và hiển thị ra các trường trong form
Khi người dùng click reset thì xoá toàn bộ dữ liệu sv trong form
Khi người dùng click vào update thì cập nhật dữ liệu trong CSDL và xoá tất cả các dữ liệu trong form.
Tags: