By GokiSoft.com|
10:04 09/10/2021|
C Sharp
Bài tập ôn luyện OOP + Interface trong C# - Lập Trình C# - Lập Trình C Sharp
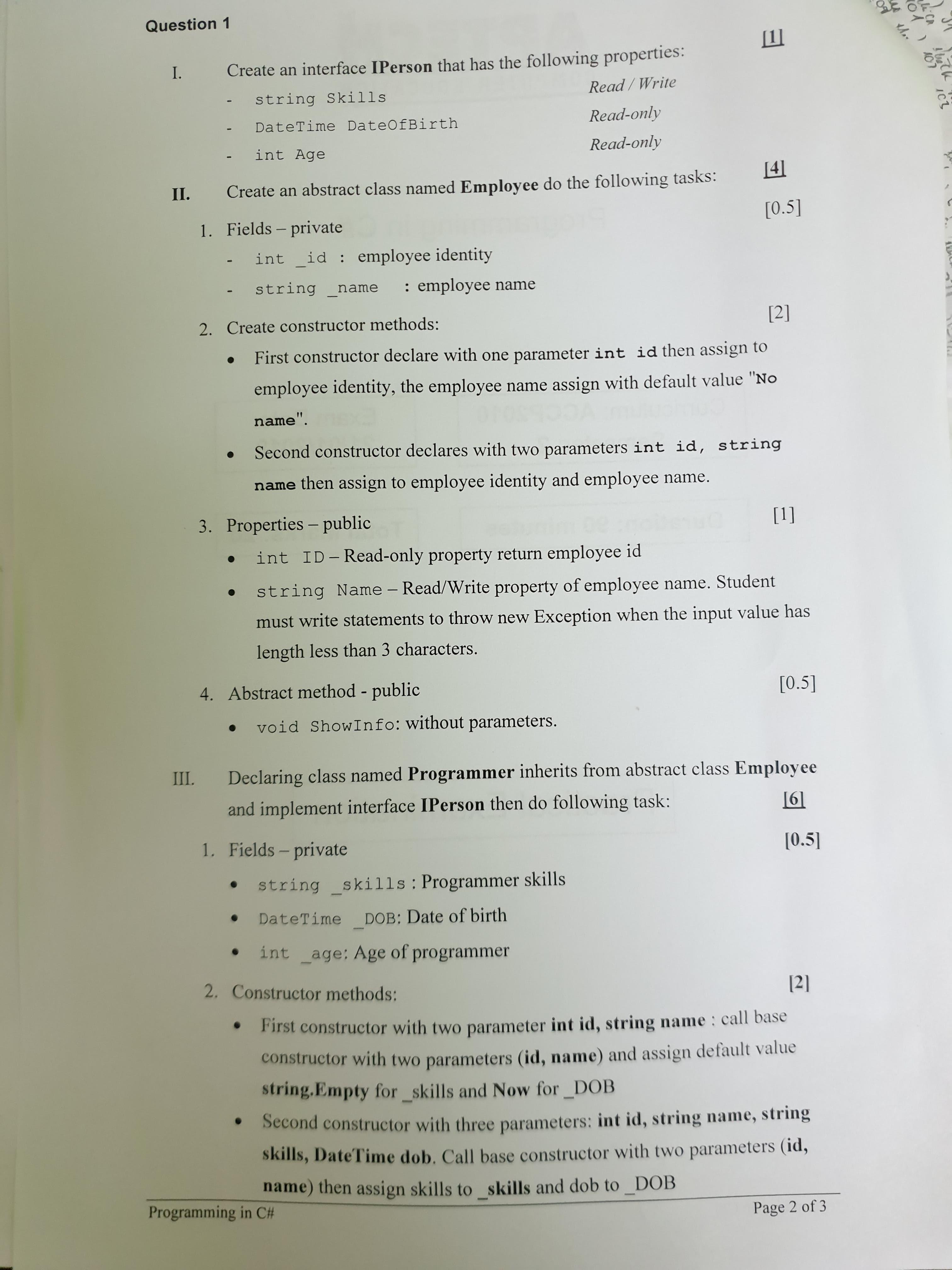
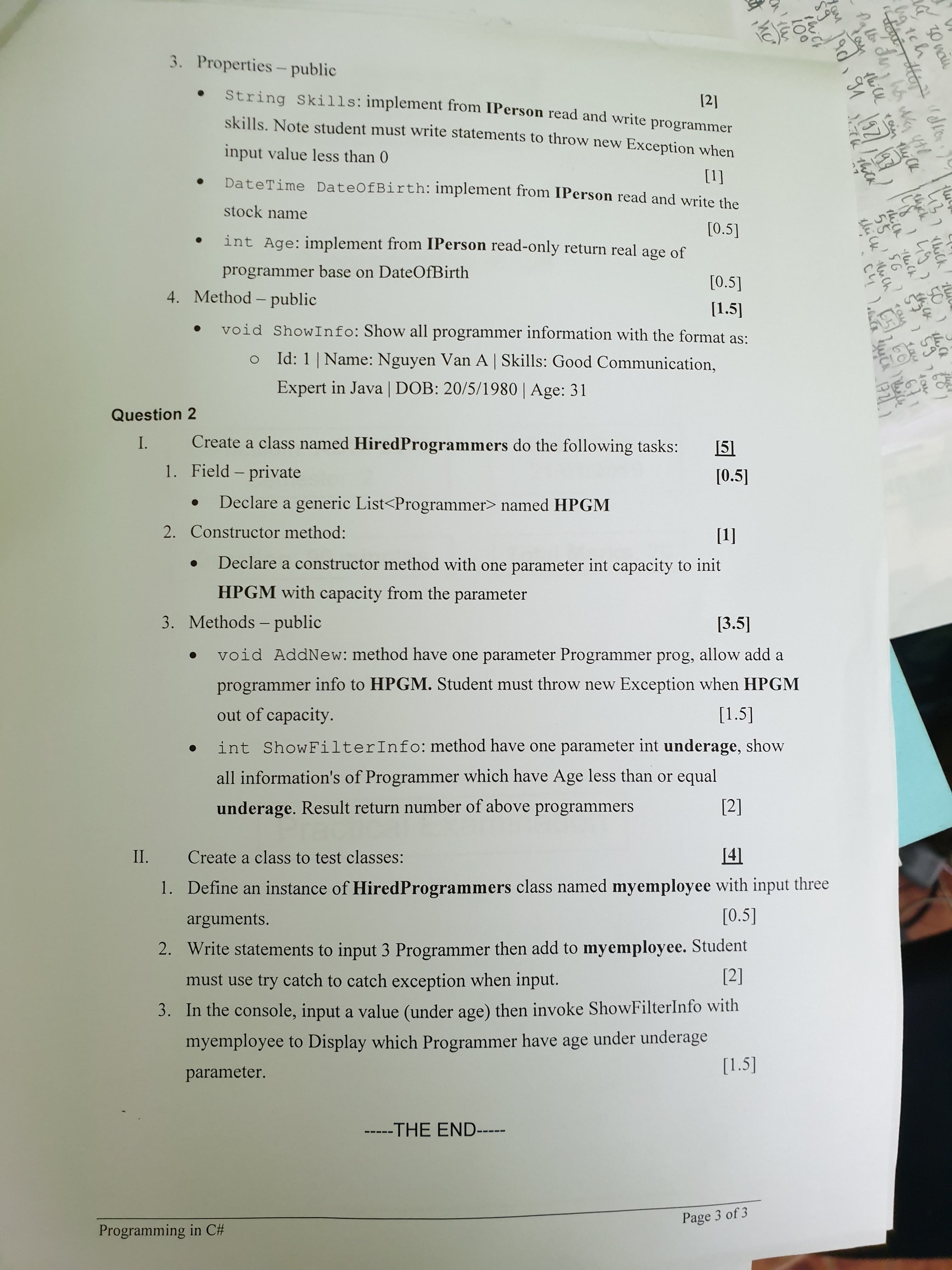
Tags:
Phản hồi từ học viên
5
(Dựa trên đánh giá ngày hôm nay)
![Nguyễn Hùng Anh [community,C2009G]](https://www.gravatar.com/avatar/32d1c530d2d2c1f3cd46282949f78ebf.jpg?s=80&d=mm&r=g)
Nguyễn Hùng Anh
2021-10-30 03:17:15
#Employee.cs
using System;
using System.Collections.Generic;
using System.Text;
namespace Bt1506
{
abstract class Employee
{
private int _id;
public int ID
{
get
{
return _id;
}
}
private string _name;
public string Name
{
get
{
return _name;
}
set
{
if(value.Length < 3)
{
throw new Exception();
}
_name = value;
}
}
protected Employee(int id)
{
_id = id;
Name = "No Name";
}
protected Employee(int id, string name) : this(id)
{
Name = name;
}
public abstract void ShowInfo();
}
}
#HiredProgrammer.cs
using System;
using System.Collections.Generic;
using System.Text;
namespace Bt1506
{
class HiredProgrammer
{
private List<Programmer> HPGM;
public HiredProgrammer(int capacity)
{
HPGM = new List<Programmer>(capacity);
}
public void AddNew(Programmer prog)
{
try
{
HPGM.Add(prog);
} catch (IndexOutOfRangeException)
{
throw new Exception("Out of capacity");
}
}
public int ShowFilterInfo(int underage)
{
int count = 0;
foreach(Programmer prog in HPGM)
{
if(prog.Age <= underage)
{
count++;
}
}
return count;
}
}
}
#IPerson.cs
using System;
using System.Collections.Generic;
using System.Text;
namespace Bt1506
{
interface IPerson
{
string Skills { get; set; }
DateTime DateOfBirth { get; }
int Age { get; }
}
}
#Program.cs
using System;
using System.Collections.Generic;
namespace Bt1506
{
class Program
{
static HiredProgrammer myemployee;
static void Main(string[] args)
{
myemployee = new HiredProgrammer(3);
for (int i = 0; i < 3; i++)
{
Console.WriteLine("Nhap ten:");
string name = Console.ReadLine();
Console.WriteLine("Nhap ky nang:");
string skills = Console.ReadLine();
Console.WriteLine("Nhap DOB(yyyy-MM-dd):");
string dobStr = Console.ReadLine();
DateTime dob = ConvertStringToDateTime(dobStr);
Programmer prob = new Programmer(skills, dob, i+1, name);
myemployee.AddNew(prob);
prob.ShowInfo();
}
Console.WriteLine("Nhap do tuoi can kiem tra:");
int checkAge = int.Parse(Console.ReadLine());
int checkCount = myemployee.ShowFilterInfo(checkAge);
Console.WriteLine("So nhan vien duoi {0} tuoi la {1}", checkAge, checkCount);
}
static DateTime ConvertStringToDateTime(string str)
{
DateTime myDate = DateTime.ParseExact(str, "yyyy-MM-dd",
System.Globalization.CultureInfo.InvariantCulture);
return myDate;
}
}
}
#Programmer.cs
using System;
using System.Collections.Generic;
using System.Text;
namespace Bt1506
{
class Programmer : Employee, IPerson
{
private string _skills;
public string Skills {
get
{
return _skills;
}
set
{
if(value.Length == 0)
{
throw new Exception();
}
_skills = value;
}
}
private DateTime _DOB;
public DateTime DateOfBirth {
get
{
return _DOB;
}
set
{
_DOB = value;
}
}
private int _age;
public int Age {
get
{
int year = int.Parse(DateOfBirth.ToString("yyyy"));
int currentYear = int.Parse(DateTime.Now.ToString("yyyy"));
_age = currentYear - year;
return _age;
}
}
public Programmer(int id, string name):base(id,name)
{
_skills = string.Empty;
DateOfBirth = DateTime.Now;
}
public Programmer(string skills, DateTime dob, int id, string name) : base(id, name)
{
Skills = skills;
DateOfBirth = dob;
}
public override void ShowInfo()
{
Console.WriteLine("Id: {0} | Name: {1} | Skills: {2} | DOB: {3} | Age: {4}",
ID, Name, Skills, DateOfBirth, Age);
}
}
}
![Hieu Ngo [community,C2009G]](https://www.gravatar.com/avatar/cee2973101b9cf9580ef561ff3eeecf0.jpg?s=80&d=mm&r=g)
Hieu Ngo
2021-10-30 03:09:26
#Utility.cs
using System;
using System.Collections.Generic;
using System.Globalization;
using System.Text;
namespace Programmer
{
class Utility
{
public static int ReadInt()
{
int value;
while(true)
{
try
{
value = int.Parse(Console.ReadLine());
return value;
}catch
{
Console.WriteLine("Error");
}
}
}
public static DateTime StringCovertToDate(string dateStr)
{
DateTime myDate = DateTime.ParseExact(dateStr, "dd/MM/yyyy", CultureInfo.InvariantCulture);
return myDate;
}
public static string DateTimeCovertToString(DateTime myDate)
{
return myDate.ToString("dd/MM/yyyy");
}
}
}
#Employee.cs
using System;
using System.Collections.Generic;
using System.Text;
namespace Programmer
{
abstract class Employee
{
private int _id;
public int ID
{
get
{
return _id;
}
}
public string Name
{
get
{
return _name;
}
set
{
if (value.Length < 3)
{
throw new Exception();
}
_name = value;
}
}
private string _name;
public Employee(int id)
{
_id = id;
Name = "No Name";
}
public Employee(int id, string name) : this(id)
{
Name = name;
}
public void Input()
{
Console.WriteLine("Id :");
_id = int.Parse(Console.ReadLine());
Console.WriteLine("Name :");
Name = Console.ReadLine();
}
public abstract void ShowInfo();
}
}
#HiredProgrammers.cs
using System;
using System.Collections.Generic;
using System.Text;
namespace Programmer
{
class HiredProgrammers
{
private List<Programmer> HPGM;
public HiredProgrammers(int capacity)
{
HPGM = new List<Programmer>(capacity);
}
public void AddNew(Programmer prog)
{
try
{
HPGM.Add(prog);
} catch(IndexOutOfRangeException)
{
throw new Exception("So phan tu lon hon suc chua cua mang");
}
}
public int ShowFillterInfo(int underage)
{
int num = 0;
foreach(Programmer program in HPGM)
{
if(program.Age <= underage)
{
num++;
}
}
return num;
}
public void Show(int underage)
{
int num = 0;
foreach (Programmer program in HPGM)
{
if (program.Age <= underage)
{
program.ShowInfo();
}
}
}
}
}
#IPerson.cs
using System;
using System.Collections.Generic;
using System.Text;
namespace Programmer
{
interface IPerson
{
public string Skills { get; set; }
public DateTime DateOfBirth { get; }
public int Age { get; }
}
}
#Program.cs
using System;
namespace Programmer
{
class Program
{
static HiredProgrammers myEmployee = new HiredProgrammers(3);
static Programmer programmer;
static void Main(string[] args)
{
for(int i = 0; i<3; i++)
{
Console.WriteLine("Name :");
String name = Console.ReadLine();
int id = i + 1;
programmer = new Programmer(id, name);
Console.WriteLine("Skills :");
programmer.Skills = Console.ReadLine();
Console.WriteLine("DOB :");
programmer.DateOfBirth = Utility.StringCovertToDate(Console.ReadLine());
myEmployee.AddNew(programmer);
}
Console.WriteLine();
Console.WriteLine("underage:");
int underage = Utility.ReadInt();
int t = myEmployee.ShowFillterInfo(underage);
Console.WriteLine("under age = " + t);
myEmployee.Show(underage);
}
}
}
#Programmer.cs
using System;
using System.Collections.Generic;
using System.Text;
namespace Programmer
{
class Programmer : Employee, IPerson
{
private string _skills;
public string Skills {
get
{
return _skills;
}
set
{
if(value.Length ==0)
{
throw new Exception();
}
_skills = value;
}
}
private DateTime _DOB;
public DateTime DateOfBirth {
get
{
return _DOB;
}
set
{
_DOB = value;
}
}
private int _age;
public int Age {
get
{
int year = int.Parse(DateOfBirth.ToString("yyyy"));
int currentYear = int.Parse(DateTime.Now.ToString("yyyy"));
_age = currentYear - year;
return _age;
}
}
public Programmer(int id, string name) : base (id, name)
{
_skills = string.Empty;
_DOB = DateTime.Now;
}
public Programmer(string skills, DateTime dob,int id, string name) : base(id, name)
{
Skills = skills;
DateOfBirth = dob;
}
public override void ShowInfo()
{
Console.WriteLine("Id: {0} | Name: {1} | Skills: {2} | DOB: {3} | Age: {4}",
ID, Name, Skills, DateOfBirth, Age);
}
}
}
![Nguyễn Việt Hoàng [community,AAHN-C2009G]](https://www.gravatar.com/avatar/bdbde8074d82148dcda6927ccb016466.jpg?s=80&d=mm&r=g)
Nguyễn Việt Hoàng
2021-10-14 15:37:35
#Program.cs
using Ss11.B1;
using System;
namespace Ss11
{
class Program
{
static void Main(string[] args)
{
HiredProgramers myemployee = new HiredProgramers();
myemployee.AddNew();
myemployee.AddNew();
myemployee.AddNew();
myemployee.ShowFilterInfo(8);
}
}
}
![Nguyễn Việt Hoàng [community,AAHN-C2009G]](https://www.gravatar.com/avatar/bdbde8074d82148dcda6927ccb016466.jpg?s=80&d=mm&r=g)
Nguyễn Việt Hoàng
2021-10-14 15:27:09
#Employee.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Ss11.B1
{
abstract class Employee
{
private int _id;
public int Id
{
get
{
return this._id;
}
set
{
this._id = value;
}
}
private string _name;
public string Name
{
get
{
return this._name;
}
set
{
while(value.Length <= 3)
{
Console.WriteLine("Lenght Name must be > 3 characters !");
Console.WriteLine("Enter Name");
value = Console.ReadLine();
}
this._name = value;
}
}
public Employee()
{
this.Id = 0;
this.Name = "No Name";
}
public Employee(int id, string name)
{
this.Id = id;
this.Name = name;
}
public void Input()
{
Console.WriteLine("Id :");
Id = int.Parse(Console.ReadLine());
Console.WriteLine("Name :");
Name = Console.ReadLine();
}
public abstract void ShowInfo();
}
}
#HiredProgramers.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Ss11.B1
{
class HiredProgramers
{
public List<Programer> HPGM { get; set; }
public HiredProgramers()
{
HPGM = new List<Programer>();
}
public HiredProgramers(List<Programer> hpgm)
{
this.HPGM = hpgm;
}
public void AddNew()
{
Programer p = new Programer();
p.Input();
HPGM.Add(p);
}
public int ShowFilterInfo(int underage)
{
int count = 0;
foreach(Programer p in HPGM)
{
if(p.Ages <= underage)
{
p.ShowInfo();
count++;
}
}
if(count == 0)
{
Console.WriteLine("No Find Age");
}
return count;
}
}
}
#IPerson.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Ss11.B1
{
interface IPerson
{
public string Skills();
public DateTime DateOfBirth();
public int Age();
}
}
#Programer.cs
using Ss11.Ultitis;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Ss11.B1
{
class Programer : Employee , IPerson
{
private string _skill;
public string Skill
{
get
{
return this._skill;
}
set
{
/*while(value.Length <= 0)
{
Console.WriteLine("Skill must be lenght > 0 !");
Console.WriteLine("Enter Skill :");
value = Console.ReadLine();
}*/
this._skill = value;
}
}
private DateTime _dob;
public DateTime DOB
{
get
{
return this._dob;
}
set
{
this._dob = value;
}
}
private int _age;
public int Ages
{
get
{
return this._age;
}
set
{
this._age = value;
}
}
public Programer()
{
base.Id = 0;
base.Name = "No Name";
this.Skill = "";
this.DOB = DateTime.Now;
}
public Programer(int id, string name , string skill, DateTime dob, int age) : base (id, name)
{
this.Skill = skill;
this.DOB = dob;
this.Ages = age;
}
public override void ShowInfo()
{
Console.WriteLine("Id :{0}, Name :{1}, Skill :{2}, DOB :{3}, Age :{4}",base.Id, base.Name,Skills(), DateOfBirth(), Age());
}
public string Skills()
{
Console.WriteLine("Enter Skill :");
string skills = Console.ReadLine();
if(skills.Length <= 0)
{
Console.WriteLine("Skill lenght must be > 0 !");
Console.WriteLine("Enter Skill Again :");
skills = Console.ReadLine();
}
return skills;
}
public DateTime DateOfBirth()
{
Console.WriteLine("Enter Date Of Birth :");
DateTime dob = Ultility.ConvertStringToDateTime(Console.ReadLine());
return dob;
}
public int Age()
{
Console.WriteLine("Age :");
int age = DateTime.Now.Year - DateOfBirth().Year;
return age;
}
}
}
![hainguyen [T2008A]](https://www.gravatar.com/avatar/32855ce6db55d60134d830aee06b41e5.jpg?s=80&d=mm&r=g)
hainguyen
2021-05-26 08:28:37
#Employee.cs
using System;
using System.Collections.Generic;
using System.Text;
namespace Lesson05
{
abstract class Employee
{
private int _id;
private string _name;
public int id
{
get
{
return _id;
}
}
public string name
{
get
{
return _name;
}
set
{
checkName(value);
}
}
public Employee() { }
public Employee(int id)
{
this._id = id;
this._name = "NO name";
}
public Employee(int id, string name)
{
this._id = id;
this._name = name;
}
private void checkName(string name)
{
bool isCheck = false;
while (!isCheck)
{
if(name.Length < 3)
{
Console.WriteLine("Name.Length > 3");
} else
{
_name = name;
isCheck = true;
}
}
}
public abstract void showInfor();
}
}
#IPerson.cs
using System;
using System.Collections.Generic;
using System.Text;
namespace Lesson05
{
class IPerson
{
public string Skills { get; set; }
public DateTime DateOfBirth { get; set; }
public int Age { get; set; }
public IPerson() { }
public IPerson(string Skills, DateTime DateOfBirth, int Age)
{
this.Skills = Skills;
this.DateOfBirth = DateOfBirth;
this.Age = Age;
}
}
}
#Programmer.cs
using System;
using System.Collections.Generic;
using System.Text;
namespace Lesson05
{
class Programmer : Employee, IPerson
{
private string _Skill { get; set; }
private DateTime _DOB { get; set; }
private int Age { get; set; }
public Programmer(int id, string name) : base (id, name)
{
this._Skill = string.Empty;
this._DOB = DateTime.Now;
}
public Programmer(int id, string name, string skill, DateTime dob) : base (id, name)
{
this._Skill = skill;
this._DOB = dob;
}
public string Skill
{
get
{
return _Skill;
}
set
{
if(value.Length < 0)
{
Console.WriteLine("lon hon 0");
}
_Skill = value;
}
}
public DateTime DateOfBirth
{
get
{
return _DOB;
}
set
{
_DOB = value;
}
}
public int age
{
get
{
Age = DateTime.Now.Year - _DOB.Year;
return Age;
}
}
public override void showInfor()
{
Console.WriteLine("id: " + id + "| Skill: " + _Skill + "| DOB: " + _DOB + "| Age: " + age);
}
}
}
![Do Trung Duc [T2008A]](https://www.gravatar.com/avatar/2973ac07124f066b4605c535e8d39a99.jpg?s=80&d=mm&r=g)
Do Trung Duc
2021-05-25 04:04:13
using System;
using System.Collections.Generic;
using System.Text;
namespace Lesson5.OntapInterface
{
public interface IPerson
{
public string Skills { get; set; }
public DateTime DateOfBirth { get; }
public int Age { get; }
};
}
![Do Trung Duc [T2008A]](https://www.gravatar.com/avatar/2973ac07124f066b4605c535e8d39a99.jpg?s=80&d=mm&r=g)
Do Trung Duc
2021-05-25 04:04:00
using System;
using System.Collections.Generic;
using System.Text;
namespace Lesson5.OntapInterface
{
abstract class Employee
{
private int _id;
private string _name;
public int id
{
get
{
return _id;
}
}
public string name
{
get
{
return _name;
}
set
{
if (value.Length < 3)
{
throw new Exception("Khong Nhap chuoi it hon3 ky tu");
}
else
{
_name = value;
}
}
}
public Employee(int id)
{
this._id = id;
this._name = "No Name";
}
public Employee(int id, string name)
{
this._id = id;
this._name = name;
}
public abstract void ShowInfo();
}
}
![Do Trung Duc [T2008A]](https://www.gravatar.com/avatar/2973ac07124f066b4605c535e8d39a99.jpg?s=80&d=mm&r=g)
Do Trung Duc
2021-05-25 04:03:47
using System;
using System.Collections.Generic;
using System.Text;
namespace Lesson5.OntapInterface
{
class Programmer : Employee, IPerson
{
private string _skills;
private DateTime _DOB;
private int _age;
public Programmer(int id, string name) : base(id,name)
{
this._skills = string.Empty;
this._DOB = DateTime.Now;
}
public Programmer(int id, string name, string skills, DateTime dob) : base(id, name)
{
this._skills = skills;
this._DOB = dob;
}
public string Skills
{
get
{
return _skills;
}
set
{
if(value.Length < 0)
{
throw new Exception("SO ky tu nhap vao khong nho hon 0");
}
else
{
_skills = value;
}
}
}
public DateTime DateOfBirth
{
get
{
return _DOB;
}
set
{
_DOB = value;
}
}
public int Age
{
get
{
_age = DateTime.Now.Year - DateOfBirth.Year;
return _age;
}
}
public override void ShowInfo()
{
Console.WriteLine("ID: {0}, Name: {1}, Skill: {2}, DOB: {3}, Age: {4}", id, name, Skills, DateOfBirth, Age);
}
}
}
![Do Trung Duc [T2008A]](https://www.gravatar.com/avatar/2973ac07124f066b4605c535e8d39a99.jpg?s=80&d=mm&r=g)
Do Trung Duc
2021-05-25 04:03:33
using System;
using System.Collections.Generic;
using System.Text;
namespace Lesson5.OntapInterface
{
class HiredProgammers
{
List<Programmer> HPGM;
public HiredProgammers(int capacity)
{
HPGM = new List<Programmer>(capacity);
}
public void AddNew(Programmer prog)
{
try {
HPGM.Add(prog);
} catch (IndexOutOfRangeException)
{
throw new Exception("So phan tu lon hon suc chua cua mang");
}
}
public int ShowFillterInfo(int underage)
{
int num = 0;
foreach(Programmer prog in HPGM)
{
if (prog.Age < underage)
{
num++;
}
}
return num;
}
}
}
![Do Trung Duc [T2008A]](https://www.gravatar.com/avatar/2973ac07124f066b4605c535e8d39a99.jpg?s=80&d=mm&r=g)
Do Trung Duc
2021-05-25 04:03:16
using System;
namespace Lesson5.OntapInterface
{
class test
{
static HiredProgammers myemployee = new HiredProgammers(3);
static void Main(string[] args)
{
for(int i = 0; i < 3; i++)
{
Console.WriteLine("nhap ten nhan vien thu {0}: ", i+1);
string name = Console.ReadLine();
Console.WriteLine("Nhap skill nhan vien:");
string skills = Console.ReadLine();
Console.WriteLine("Nhap ngay thang nam sinh nhan vien:");
DateTime dob = DateTime.Parse(Console.ReadLine());
Programmer programmer = new Programmer(i+1, name, skills, dob);
Console.WriteLine("Tuoi: " + programmer.Age );
myemployee.AddNew(programmer);
}
Console.WriteLine("Nhap vao do tuoi can kiem tra:");
int checkage = Int32.Parse(Console.ReadLine());
int checknumber = myemployee.ShowFillterInfo(checkage);
Console.WriteLine("So nhan vien duoi {0} tuoi la {1}", checkage, checknumber);
}
}
}