By GokiSoft.com|
20:39 25/10/2023|
Học JS
Bài tập - Quản lý sản phẩm bằng javascript - lập trình javascript
Xây dựng một website như sau
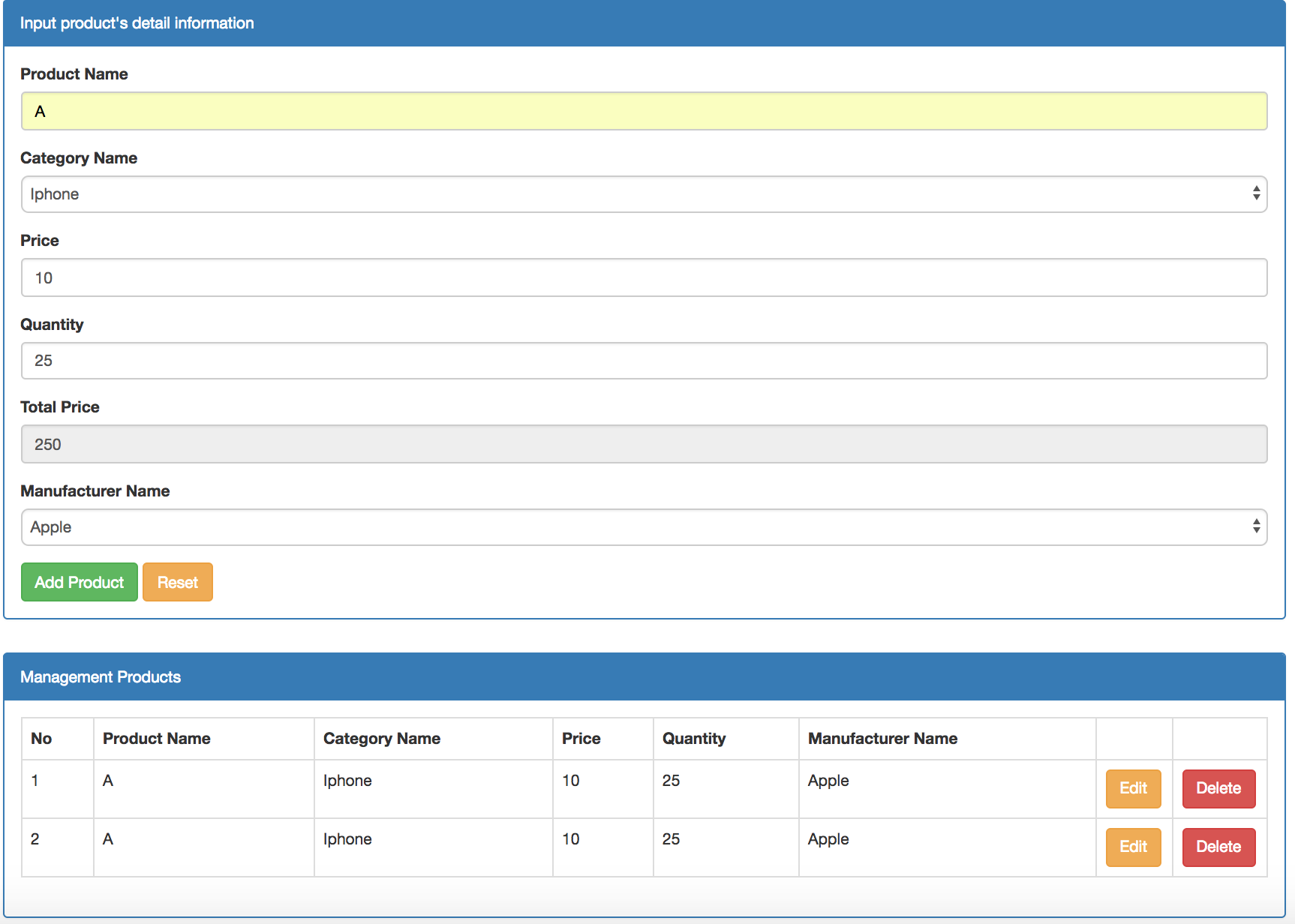
Yêu cầu :
Hoàn thành các chức năng : Thêm, sửa, xoá như hình trên.
Mục manafacturer name -> Chứa danh sách các nhà sản xuất (Apple, SamSung, LG, Sony, Google)
Khi lựa chọn từng danh mục trên thì sẽ xuất hiện trong mục : Category Name tương ứng các loại sản phâm của nhà sản xuất đó
Ví dụ : chọn apple thì category name sẽ xuất hiện IPhone, Ipad, IPod,...
Khi người dùng điện price và quantity thì sẽ tự tính giá trị cho TotalPrice = price * quantity
Tags:
Phản hồi từ học viên
5
(Dựa trên đánh giá ngày hôm nay)
![TRẦN VĂN ĐIỆP [Teacher]](https://www.gravatar.com/avatar/fc6ba9324e017d540af3613b3a77dd21.jpg?s=80&d=mm&r=g)
TRẦN VĂN ĐIỆP
2021-02-27 13:03:08
#bt2019.js
//Phan 1: Xu ly Form du lieu
//Item 1: Xu ly du lieu trong Manufacturer Name & Category Name
var dataList = {
"Apple": ["iPhone", "iPad", "Macbook"],
"Sam Sung": ["Samsung galaxy 5", "Samsung galaxy 5s"],
"LG": ["LG01", "LG02"]
}
// Fill du lieu tu dataList -> select (manufacturer name)
var manufacturerTag = document.getElementById('manufacturer_name')
for(m_name in dataList) {
console.log(m_name)
manufacturerTag.innerHTML += `<option value="${m_name}">${m_name}</option>`
// manufacturerTag.innerHTML += '<option value="'+m_name+'">'+m_name+'</option>'
}
var categoryTag = document.getElementById('category_name')
function changeManufacturerName() {
// Lay ra duoc du lieu vua chon
var value = manufacturerTag.value
categoryTag.innerHTML = '<option value="">-- Select --</option>'
if(value != '') {
var categoryList = dataList[value]
for (var i = 0; i < categoryList.length; i++) {
categoryTag.innerHTML += `<option value="${categoryList[i]}">${categoryList[i]}</option>`
}
}
}
// Item 2: Xu ly input
var priceTag = document.getElementById('price')
var quantityTag = document.getElementById('quantity')
var totalPriceTag = document.getElementById('total_price')
function updateTotalPrice() {
price = priceTag.value
quantity = quantityTag.value
totalPriceTag.value = price * quantity
}
// Phan 2: Xu ly them/sua/xoa san pham
var productList = [] //Chua tat ca cac san pham => thuc hien add hien thi len table
var productNameTag = document.getElementById('product_name')
var resultTag = document.getElementById('result')
var currentIndex = -1
function addProduct() {
productName = productNameTag.value
price = priceTag.value
quantity = quantityTag.value
totalPrice = totalPriceTag.value
manufacturerName = manufacturerTag.value
categoryName = categoryTag.value
var product = {
'productName': productName,
'manufacturerName': manufacturerName,
'categoryName': categoryName,
'price': price,
'quantity': quantity,
'totalPrice': totalPrice,
}
if(currentIndex >= 0) {
productList[currentIndex] = product
currentIndex = -1
saveBtn.innerHTML = 'Add Product'
} else {
productList.push(product)
}
console.log(product)
// resultTag.innerHTML += `<tr>
// <td>${productList.length}</td>
// <td>${product.productName}</td>
// <td>${product.manufacturerName}</td>
// <td>${product.categoryName}</td>
// <td>${product.price}</td>
// <td>${product.quantity}</td>
// <td><button onclick="editItem(${productList.length-1})">Edit</button></td>
// <td><button onclick="deleteItem(${productList.length-1})">Delete</button></td>
// </tr>`
showData()
// Xoa du lieu di
// document.getElementById('reset_btn').click()
resetData()
}
function resetData() {
productNameTag.value = ''
priceTag.value = ''
quantityTag.value = ''
totalPriceTag.value = ''
manufacturerTag.value = ''
categoryTag.value = ''
}
function deleteItem(index) {
console.log(index)
productList.splice(index, 1)
showData()
}
function showData() {
//Hien thi lai danh sach san pham trong table
resultTag.innerHTML = ''
for (var i = 0; i < productList.length; i++) {
product = productList[i]
resultTag.innerHTML += `<tr>
<td>${i + 1}</td>
<td>${product.productName}</td>
<td>${product.manufacturerName}</td>
<td>${product.categoryName}</td>
<td>${product.price}</td>
<td>${product.quantity}</td>
<td><button onclick="editItem(${i})">Edit</button></td>
<td><button onclick="deleteItem(${i})">Delete</button></td>
</tr>`
}
}
var saveBtn = document.getElementById('save_btn')
function editItem(index) {
currentIndex = index
productNameTag.value = productList[index].productName
priceTag.value = productList[index].price
quantityTag.value = productList[index].quantity
totalPriceTag.value = productList[index].totalPrice
manufacturerTag.value = productList[index].manufacturerName
changeManufacturerName()
categoryTag.value = productList[index].categoryName
saveBtn.innerHTML = 'Update Product'
}
#style.css
.container {
width: 640px;
margin: 0px auto;
}
.panel {
width: 100%;
border: solid #21adf0 2px;
margin-bottom: 20px;
}
.panel-heading {
background-color: #21adf0;
color: white;
font-weight: bold;
padding: 6px;
}
.panel-body {
display: block;
}
.panel-body label, .panel-body input, .panel-body select, .panel-body .form-group {
display: block;
width: 96%;
font-size: 16px;
margin: 0px auto;
margin-bottom: 6px;
}
.panel-body label {
font-weight: bold;
}
.panel-body table {
width: 96%;
margin: 0px auto;
margin-top: 10px;
margin-bottom: 10px;
}
table, th, td {
border: solid #c0bfbf 1px;
}
th, td {
padding: 5px;
}
#vidu.html
<!DOCTYPE html>
<html>
<head>
<title>Quan ly san pham - page</title>
<meta charset="utf-8">
<!-- code css -->
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div class="container">
<div class="panel">
<div class="panel-heading">
Input Product's Detail Information
</div>
<div class="panel-body">
<form method="post" id="MyForm">
<label>Product Name: </label>
<input type="text" id="product_name">
<label>Manufacturer Name: </label>
<select id="manufacturer_name" onchange="changeManufacturerName()">
<option value="">-- Select --</option>
</select>
<label>Category Name: </label>
<select id="category_name">
<option value="">-- Select --</option>
</select>
<label>Price: </label>
<input type="number" id="price" onkeyup="updateTotalPrice()">
<label>Quantity: </label>
<input type="number" id="quantity" onkeyup="updateTotalPrice()">
<label>Total Price: </label>
<input type="number" id="total_price" disabled="true">
<div class="form-group">
<button type="button" onclick="addProduct()" style="margin-left: 0px !important;" id="save_btn">Add Product</button>
<button type="reset" id="reset_btn">Reset</button>
</div>
</form>
</div>
</div>
<div class="panel">
<div class="panel-heading">
Product List Management
</div>
<div class="panel-body">
<table border="1" cellspacing="0">
<thead>
<tr>
<th>No</th>
<th>Product Name</th>
<th>Manufacturer Name</th>
<th>Category Name</th>
<th>Price</th>
<th>Quantity</th>
<th></th>
<th></th>
</tr>
</thead>
<tbody id="result">
</tbody>
</table>
</div>
</div>
</div>
<!-- code js -->
<script type="text/javascript" src="bt2019.js"></script>
</body>
</html>
![Do Trung Duc [T2008A]](https://www.gravatar.com/avatar/2973ac07124f066b4605c535e8d39a99.jpg?s=80&d=mm&r=g)
Do Trung Duc
2020-10-26 02:35:48
<!DOCTYPE html>
<html>
<head>
<title>Quản lý sản phẩm bằng javascript </title>
<meta charset="utf-8">
<style type="text/css">
.panel {
width: 60%;
margin: 0 auto;
/*border: solid #4267b2 1px;*/
}
.panel-heading {
background-color: #4267b2;
padding: 10px;
color: white;
}
.panel-body {
padding: 10px;
}
.panel-body label {
font-weight: bold;
}
.form-group {
/*display: block;*/
margin-bottom: 20px;
}
.form-control {
/*display: block;*/
width: 98%;
font-size: 16px;
margin-top: 10px;
}
.table {
width: 100%;
}
.table tr {
border-bottom: solid blue 1px;
}
</style>
</head>
<body>
<div class="panel">
<div class="panel-heading">
Input product detail information
</div>
<div class="panel-body">
<form method="post">
<div class="form-group">
<label>Product Name:</label>
<input type="number" name="index" id="index" value="">
<input class="form-control" type="text" name="product_name" id="product_name" placeholder="Enter product name">
</div>
<div class="form-group">
<label>Manufacturer Name:</label>
<select class="form-control" id="manuafaturer_name" onchange="changeManufaturer()">
<option value="">-- Choose --</option>
</select>
</div>
<div class="form-group">
<label>Category Name:</label>
<select class="form-control" id="category_name">
</select>
</div>
<div class="form-group">
<label>Price:</label>
<input class="form-control" type="number" name="price" id="price" placeholder="Enter price" onkeyup="updateTotalPrice()">
</div>
<div class="form-group">
<label>Quantity:</label>
<input class="form-control" type="text" name="quantity" id="quantity" placeholder="Enter quantity" onkeyup="updateTotalPrice()">
</div>
<div class="form-group">
<label>Total Price:</label>
<input class="form-control" type="text" name="total_price" id="total_price" value="0">
</div>
<div class="form-group">
<button class="btn btn-success" type="button" onclick="addProduct()">Add Produce</button>
<button class="btn btn-danger" type="Reset">Reset</button>
</div>
</form>
</div>
</div>
<div class="panel" style="margin-top: 20px;">
<div class="panel-heading">
Product List
</div>
<div class="panel-body">
<table class="table" id="result">
<thead>
<tr>
<th>No</th>
<th>Product Name</th>
<th>Manufacture Name</th>
<th>Category Name</th>
<th>Price</th>
<th>Quantity</th>
<th>Total Price</th>
<th></th>
<th></th>
</tr>
</thead>
<tbody>
</tbody>
</table>
</div>
</div>
<script type="text/javascript">
var manufactureList = {
"Apple": ["Iphone 5", "Iphone 7", "Iphone 12"],
"Samsung": ["Galaxy 5", "Galaxy 10"],
"LG": ["Xperia Z1", "Xperia Z2", "Xperia Z3"],
'ABC':null
}
var productList = []
var manuafaturerTag = document.getElementById('manuafaturer_name')
for (var key in manufactureList) {
manuafaturerTag.innerHTML += `<option value='${key}'>${key}</option>`
}
function changeManufaturer(){
key = document.getElementById('manuafaturer_name').value
categoryList = manufactureList[key]
var categoryTag = document.getElementById('category_name')
categoryTag.innerHTML = ''
if (categoryList != null) {
for (var i =0; i < categoryList.length; i++) {
categoryTag.innerHTML += `<option value='${categoryList[i]}'>${categoryList[i]}</option>`
}
}
}
function updateTotalPrice(){
var price = document.getElementById('price').value
var quantity = document.getElementById('quantity').value
var totalPrice = price*quantity
document.getElementById('total_price').value = totalPrice
}
count = 0
function addProduct() {
var index = document.getElementById('index').value
var productName = document.getElementById('product_name').value
var manufactureName = document.getElementById('manuafaturer_name').value
var categoryName = document.getElementById('category_name').value
var price = document.getElementById('price').value
var quantity = document.getElementById('quantity').value
var totalPrice = document.getElementById('total_price').value
var product= {
'productName': productName,
'manufactureName': manufactureName,
'categoryName': categoryName,
'price': price,
'quantity': quantity
}
if(index != ''){
productList[index] = product
showProduct()
return;
}
productList.push(product)
document.getElementById('result').innerHTML += `<tr>
<td>${++count}</td>
<td>${productName}</td>
<td>${manufactureName}</td>
<td>${categoryName}</td>
<td>${price}</td>
<td>${quantity}</td>
<td>${totalPrice}</td>
<td><button onclick="editProduct(${count-1})">Edit</button></td>
<td><button onclick="deleteProduct(${count-1})">Delete</button></td>
</tr>`
}
function deleteProduct(index) {
productList.splice(index,1)
showProduct()
}
function showProduct(){
document.getElementById('result').innerHTML = ''
for (var i =0; i < productList.length; i++) {
document.getElementById('result').innerHTML += `<tr>
<td>${i+1}</td>
<td>${productList[i].productName}</td>
<td>${productList[i].manufactureName}</td>
<td>${productList[i].categoryName}</td>
<td>${productList[i].price}</td>
<td>${productList[i].quantity}</td>
<td>${productList[i].totalPrice}</td>
<td><button onclick = "editProduct(${i})">Edit</button></td>
<td><button onclick="deleteProduct(${i})">Delete</button></td>
</tr>`
}
}
function editProduct(index){
var product = productList[index]
document.getElementById('product_name').value = product.productName
document.getElementById('manuafaturer_name').value = product.manufactureName
changeManufaturer()
document.getElementById('category_name').value =product.productName
document.getElementById('price').value=product.price
document.getElementById('quantity').value=product.quantity
document.getElementById('total_price').value=product.totalPrice
document.getElementById('index').value=index
}
</script>
</body>
</html>
![Nguyễn Anh Vũ [T2008A]](https://www.gravatar.com/avatar/8863d24ed74b396082becbc4db8331fd.jpg?s=80&d=mm&r=g)
Nguyễn Anh Vũ
2020-10-24 06:10:07
#qlsp.html
<!DOCTYPE html>
<html>
<head>
<title>Quản lý sản phẩm bằng javascript </title>
<meta charset="utf-8">
<style type="text/css">
.panel {
width: 60%;
margin: 0 auto;
border: solid #4267b2 1px;
}
.panel-heading {
background-color: #4267b2;
padding: 10px;
color: white;
}
.panel-body {
padding: 10px;
}
.panel-body label {
font-weight: bold;
}
.form-group {
display: block;
margin-bottom: 20px;
}
.form-control {
display: block;
width: 98%;
font-size: 16px;
margin-top: 10px;
}
.table {
width: 100%;
}
.table tr {
border-bottom: solid blue 1px;
}
</style>
</head>
<body>
<div class="panel">
<div class="panel-heading">
Input product detail information
</div>
<div class="panel-body">
<form method="post">
<div class="form-group">
<label>Product Name:</label>
<input type="number" name="index" id="index" value="" hidden="true">
<input class="form-control" type="text" name="product_name" id="product_name" placeholder="Enter product name">
</div>
<div class="form-group">
<label>Manufacturer Name:</label>
<select class="form-control" id="manuafaturer_name" onchange="changeManufaturer()">
<option value="">-- Choose --</option>
</select>
</div>
<div class="form-group">
<label>Category Name:</label>
<select class="form-control" id="category_name">
</select>
</div>
<div class="form-group">
<label>Price:</label>
<input class="form-control" type="number" name="price" id="price" placeholder="Enter price" value="0" onkeyup="updateTotalPrice()">
</div>
<div class="form-group">
<label>Quantity:</label>
<input class="form-control" type="text" name="quantity" id="quantity" placeholder="Enter quantity" value="0" onkeyup="updateTotalPrice()">
</div>
<div class="form-group">
<label>Total Price:</label>
<input class="form-control" type="text" name="total_price" id="total_price" value="0" disabled="true">
</div>
<div class="form-group">
<button class="btn btn-success" type="button" onclick="addProduct()">Add Produce</button>
<button class="btn btn-danger" type="reset">Reset</button>
</div>
</form>
</div>
</div>
<div class="panel" style="margin-top: 20px;">
<div class="panel-heading">
Product List
</div>
<div class="panel-body">
<table class="table">
<thead>
<tr>
<th>No</th>
<th>Product Name</th>
<th>Manufacture Name</th>
<th>Category Name</th>
<th>Price</th>
<th>Quantity</th>
<th>Total Price</th>
<th></th>
<th></th>
</tr>
</thead>
<tbody id="result">
</tbody>
</table>
</div>
</div>
<script type="text/javascript">
var manufactureList = {
"Apple": [
"IPhone 5", "IPhone 5s", "IPhone 12"
],
"Sam Sung": [
"Galaxy S5", "Galaxy 10"
],
"LG": [
"1", "2", "3"
]
}
var productList = []
var manuafaturerTag = document.getElementById('manuafaturer_name')
for(var key in manufactureList) {
manuafaturerTag.innerHTML += `<option value='${key}'>${key}</option>`
}
function changeManufaturer() {
key = manuafaturerTag.value
categoryList = manufactureList[key]
console.log(categoryList)
var categoruTag = document.getElementById('category_name')
categoruTag.innerHTML = ''
if(categoryList != null) {
for (var i = 0; i < categoryList.length; i++) {
categoruTag.innerHTML += `<option value='${categoryList[i]}'>${categoryList[i]}</option>`
}
}
}
function updateTotalPrice() {
var price = document.getElementById('price').value
var quantity = document.getElementById('quantity').value
totalPrice = price * quantity
document.getElementById('total_price').value = totalPrice
}
var count = 0
function addProduct() {
var index = document.getElementById('index').value
var productName = document.getElementById('product_name').value
var manufactureName = document.getElementById('manuafaturer_name').value
var categoryName = document.getElementById('category_name').value
var price = document.getElementById('price').value
var quantity = document.getElementById('quantity').value
var totalPrice = document.getElementById('total_price').value
var product = {
'productName': productName,
'manufactureName': manufactureName,
'categoryName': categoryName,
'price': price,
'quantity': quantity,
'totalPrice': totalPrice
}
if(index != '') {
productList[index] = product
showProduct()
return;
}
productList.push(product)
document.getElementById('result').innerHTML += `<tr>
<td>${++count}</td>
<td>${productName}</td>
<td>${manufactureName}</td>
<td>${categoryName}</td>
<td>${price}</td>
<td>${quantity}</td>
<td>${totalPrice}</td>
<td><button class="btn btn-warning" onclick="editProduct(${count - 1})">Edit</button></td>
<td><button class="btn btn-danger" onclick="deleteProduct(${count - 1})">Delete</button></td>
</tr>`
}
function deleteProduct(index) {
console.log(index)
productList.splice(index, 1)
showProduct();
}
function showProduct() {
document.getElementById('result').innerHTML = ''
for (var i = 0; i < productList.length; i++) {
document.getElementById('result').innerHTML += `<tr>
<td>${i+1}</td>
<td>${productList[i].productName}</td>
<td>${productList[i].manufactureName}</td>
<td>${productList[i].categoryName}</td>
<td>${productList[i].price}</td>
<td>${productList[i].quantity}</td>
<td>${productList[i].totalPrice}</td>
<td><button class="btn btn-warning" onclick="editProduct(${i})">Edit</button></td>
<td><button class="btn btn-danger" onclick="deleteProduct(${i})">Delete</button></td>
</tr>`
}
}
function editProduct(index) {
var product = productList[index]
console.log(product)
document.getElementById('index').value = index
document.getElementById('product_name').value = product.productName
document.getElementById('manuafaturer_name').value = product.manufactureName
changeManufaturer()
document.getElementById('category_name').value = product.categoryName
document.getElementById('price').value = product.price
document.getElementById('quantity').value = product.quantity
document.getElementById('total_price').value = product.totalPrice
}
</script>
</body>
</html>
![Nguyễn Xuân Mai [T2008A]](https://www.gravatar.com/avatar/d3d863d6f47708501814fb41e9c38f31.jpg?s=80&d=mm&r=g)
Nguyễn Xuân Mai
2020-10-24 04:37:48
<!DOCTYPE html>
<html>
<head>
<title>Quan ly san pham</title>
</head>
<body>
<table border="1" cellspacing="0" cellpadding="5" style="border-color: blue">
<tr style="background-color: blue; color: white; text-align: left;"><th>Input product's detail information</th></tr>
<tr ><td>
<label>Product Name></label>
<br>
<input type="number" name="index" id="index" value="" hidden="true">
<input type="text" name="product" id="product" style="background-color: #f5dec1; width: 700px">
<br>
<label>Manufacturer Name</label>
<br>
<select id="mname" onchange="changemanuf()">
<option>-- Manufacturer --</option>
</select>
<br>
<label>Category Name</label>
<br>
<select id="category"></select>
<br>
<label>Price</label>
<br>
<input type="text" name="price" id="price" style=" width: 700px" onkeyup="updateTotalPrice()">
<br>
<label>Quantity</label>
<br>
<input type="text" name="quantity" style=" width: 700px" id="quantity" onkeyup="updateTotalPrice()">
<br>
<label>Total Price</label>
<br>
<input type="text" name="product" id="totalprice" style="background-color: #ccc8be; width: 700px">
<br>
<button class="btn btn-success" type="button" onclick="addPro()">Add Product</button>
<button class="btn btn-danger" type="reset" onclick="reset()">Reset</button>
</td></tr>
</table>
<br>
<table border="1" cellspacing="0" cellpadding="3">
<tr style="background-color: blue; color: white; text-align: left;"><th>Management Products</th></tr>
<tr><td>
<table border="1" cellspacing="0">
<thead>
<tr>
<th>No</th>
<th>Product name</th>
<th>Manufacturer name</th>
<th>Category name</th>
<th>Price</th>
<th>Quantity</th>
</tr>
</thead>
<tbody id="result">
</tbody>
</table>
</td></tr>
</table>
<script type="text/javascript">
var manufactureList = {
"Apple": [
"IPhone", "IPad", "IPod"
],
"Samsung": [
"Galaxy S5", "Galaxy 10"
],
"LG": [
"Television", "Laptop", "Fridge"
],
"Sony": [
"Television", "Playstation", "Camera"
],
"Google": [
"Pixel", "Stadia", "Nest"
]
}
var productList = []
var manuafaturerTag = document.getElementById('mname')
for(var key in manufactureList) {
manuafaturerTag.innerHTML += `<option value='${key}'>${key}</option>`
}
function changemanuf() {
key = manuafaturerTag.value
categoryList = manufactureList[key]
console.log(categoryList)
var categoryTag = document.getElementById('category')
categoryTag.innerHTML = ''
if(categoryList != null) {
for (var i = 0; i < categoryList.length; i++) {
categoryTag.innerHTML += `<option value='${categoryList[i]}'>${categoryList[i]}</option>`
}
}
}
function updateTotalPrice() {
var price = document.getElementById('price').value
var quantity = document.getElementById('quantity').value
totalprice = price * quantity
document.getElementById('totalprice').value = totalprice
}
var count = 0;
function addPro() {
var index = document.getElementById('index').value
var productName = document.getElementById('product').value
var manufactureName = document.getElementById('mname').value
var categoryName = document.getElementById('category').value
var price = document.getElementById('price').value
var quantity = document.getElementById('quantity').value
var totalPrice = document.getElementById('totalprice').value
var product = {
'productName': productName,
'manufactureName': manufactureName,
'categoryName': categoryName,
'price': price,
'quantity': quantity,
'totalPrice': totalPrice
}
if(index != '') {
productList[index] = product
showProduct()
return;
}
productList.push(product)
document.getElementById('result').innerHTML += `<tr>
<td>${++count}</td>
<td>${productName}</td>
<td>${manufactureName}</td>
<td>${categoryName}</td>
<td>${price}</td>
<td>${quantity}</td>
<td>${totalPrice}</td>
<td><button class="btn btn-warning" onclick="editProduct(${count - 1})">Edit</button></td>
<td><button class="btn btn-danger" onclick="deleteProduct(${count - 1})">Delete</button></td>
</tr>`
}
function deleteProduct(index) {
console.log(index)
productList.splice(index, 1)
showProduct();
}
function showProduct() {
document.getElementById('result').innerHTML = ''
for (var i = 0; i < productList.length; i++) {
document.getElementById('result').innerHTML += `<tr>
<td>${i+1}</td>
<td>${productList[i].productName}</td>
<td>${productList[i].manufactureName}</td>
<td>${productList[i].categoryName}</td>
<td>${productList[i].price}</td>
<td>${productList[i].quantity}</td>
<td>${productList[i].totalPrice}</td>
<td><button class="btn btn-warning" onclick="editProduct(${i})">Edit</button></td>
<td><button class="btn btn-danger" onclick="deleteProduct(${i})">Delete</button></td>
</tr>`
}
}
function editProduct(index) {
var product = productList[index]
console.log(product)
document.getElementById('index').value = index
document.getElementById('product').value = product.productName
document.getElementById('mname').value = product.manufactureName
changemanuf()
document.getElementById('category').value = product.categoryName
document.getElementById('price').value = product.price
document.getElementById('quantity').value = product.quantity
document.getElementById('totalprice').value = product.totalPrice
}
function reset(){
document.getElementById('product').value=''
document.getElementById('mname').value='--Manufacturer--'
document.getElementById('category').value=''
document.getElementById('price').value=''
document.getElementById('quantity').value=''
document.getElementById('totalprice').value=''
}
</script>
</body>
</html>
![hainguyen [T2008A]](https://www.gravatar.com/avatar/32855ce6db55d60134d830aee06b41e5.jpg?s=80&d=mm&r=g)
hainguyen
2020-10-21 10:17:30
#BT.html
<!DOCTYPE html>
<html>
<head>
<title></title>
<meta charset="utf-8">
<style type="text/css">
.poin {
width: 70%;
margin: 0 auto;
border: solid 1px;
margin-top: 20px;
}
.poinding {
background-color: #4267b2;
color: white;
padding: 10px;
}
.pointer {
padding: 15px;
}
.pointer label{
font-weight: bold;
}
.first {
display: block;
margin-top: 15px;
}
.secon {
display: block;
width: 98%;
font-size: 16px;
margin-top: 10px;
}
.table {
width: 100%;
}
.table tr {
border-bottom: solid 1px;
}
</style>
</head>
<body>
<div class="poin">
<div class="poinding">
Input product detall information
</div>
<div class="pointer">
<form method="pas">
<div class="first">
<label>Product Name:</label>
<input type="number" name="index" id="index" value="" hidden="true">
<input class="secon" type="text" name="product_name" id="product_name" placeholder="Enter product name">
</div>
<div class="first">
<label>Manufacturer Name:</label>
<select class="secon" id="manufacturer_name" onchange="changeManufacturer()">
<option value="">-- Choose --</option>
</select>
</div>
<div class="first">
<label>Category Name:</label>
<select class="secon" id="category_name"></select>
</div>
<div class="first">
<label>Price:</label>
<input class="secon" type="number" name="price" id="price" placeholder="Enter price" value="0" onkeyup="updateTotalPrice()">
</div>
<div class="first">
<label>Quantity:</label>
<input class="secon" type="text" name="quantity" id="quantity" placeholder="Enter quantity" value="" onkeyup="updateTotalPrice()">
</div>
<div class="first">
<label>Tatal Price:</label>
<input class="secon" type="text" name="total_price" id="total_price" value="0" disabled="true">
</div>
<div class="first">
<button class="btn-one" type="button" onclick="addProduct()">Add Produce</button>
<button class="btn-two" type="reset">Reset</button>
</div>
</form>
</div>
</div>
<div class="poin">
<div class="poinding">
Product List
</div>
<div class="pointer">
<table class="table">
<thead>
<tr>
<th>No</th>
<th>Product Name</th>
<th>Manufacture Name</th>
<th>Category Name</th>
<th>Price</th>
<th>Quantity</th>
<th>Total Price</th>
<th></th>
<th></th>
</tr>
</thead>
<tbody id="result"></tbody>
</table>
</div>
</div>
<script type="text/javascript">
var manufactureLish = {
"Apple": ["Iphone 5", "Iphone 5s", "Iphone 11max"],
"Samsung": ["Galaxy s5", "Galaxy A7"],
"LG": ["1", "2", "3"]
}
var productLish = []
var manufactureTag = document.getElementById('manufacturer_name')
for(var key in manufactureLish) {
manufactureTag.innerHTML += `<option value = '${key}'>${key}</option>`
}
function changeManufacturer() {
key = manufactureTag.value
categoryLish = manufactureLish[key]
console.log(categoryLish)
var categoryTag = document.getElementById('category_name')
categoryTag.innerHTML = ''
if(categoryLish != null) {
for(var i = 0; i < categoryLish.length; i++) {
categoryTag.innerHTML += `<option value = '${categoryLish[i]}'>${categoryLish[i]}</option>`
}
}
}
function updateTotalPrice() {
var price = document.getElementById('price').value
var quantity = document.getElementById('quantity').value
totalPrice = price * quantity
document.getIlementById('total_price').value = totalPrice
}
var count = 0
function addProduct() {
var index = document.getElementById('index').value
var productName = document.getElementById('product_name').value
var manufactureName = document.getElementById('manufacturer_name').value
var categoryName = document.getElementById('category_name').value
var price = document.getElementById('price').value
var quantity = document.getElementById('quantity').value
var totalPrice = document.getElementById('total_price').value
var product = {
'productName': productName,
'manufactureName': manufactureName,
'categoryName': categoryName,
'price': price,
'quantity': quantity,
'totalPrice': totalPrice
}
if(index != '') {
productLish[index] = product
showProduct()
return;
}
productLish.push(product)
document.getElementById('result').innerHTML += `<tr>
<td>${++count}</td>
<td>${productName}</td>
<td>${manufactureName}</td>
<td>${categoryName}</td>
<td>${price}</td>
<td>${quantity}</td>
<td>${totalPrice}</td>
<td><button class="btn-one" onclick="editProduct(${count-1})">Edit</button></td>
<td><button class="btn-two" onclick="deleteProduct(${count-1})">Delete</button></td>
</tr>`
}
function deleteProduct(index) {
console.log(index)
productLish.splice(index, 1)
showProduct();
}
function showProduct() {
document.getElementById('result').innerHTML = ''
for (var i = 0; i < productLish.length; i++) {
document.getElementById('result').innerHTML += `<tr>
<td>${i+1}</td>
<td>${productList[i].productName}</td>
<td>${productList[i].manufactureName}</td>
<td>${productList[i].categoryName}</td>
<td>${productList[i].price}</td>
<td>${productList[i].quantity}</td>
<td>${productList[i].totalPrice}</td>
<td><button class="btn btn-warning" onclick="editProduct(${i})">Edit</button></td>
<td><button class="btn btn-danger" onclick="deleteProduct(${i})">Delete</button></td>
</tr>`
}
}
function editProduct(index) {
var product = productLish[index]
console.log(product)
document.getElementById('index').value = index
document.getElementById('product_name').value = product.productName
document.getElementById('manufacturer_name').value = product.manufactureName
changeManufacturer()
document.getElementById('category_name').value = product.categoryName
document.getElementById('price').value = product.price
document.getElementById('quantity').value = product.quantity
document.getElementById('total_price').value = product.totalPrice
}
</script>
</body>
</html>
![Nguyễn Tiến Đạt [T2008A]](https://www.gravatar.com/avatar/b5819cd0adc95c727c7ad0c2bcf6098b.jpg?s=80&d=mm&r=g)
Nguyễn Tiến Đạt
2020-10-20 17:25:12
#quanlisanpham.html
nhọc vl<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Quản lí sản phẩm</title>
<style>
.main{
border: 1px solid #4970a3;
border-radius: 5px;
width: 800px;
height: 472px;
display: block;
}
.top{
background-color: #4970a3;
width: 99.4%;
height: 30px;
padding-left: 5px;
padding-top: 5px;
color: white;
}
ul{
list-style-type: none;
}
li{
margin-bottom: 16px;
}
</style>
<script>
function changeManufacturer() {
var v=document.getElementById('select1').value;
switch(v){
case '--Select--':
document.getElementById('nsx').value='';
document.getElementById('nsx').disabled= true;
break;
case 'Apple':
var manu1=document.getElementById('nsx');
manu1.innerHTML=`
<option>--Select--</option>
<option>Iphone X</option>
<option>Ipad</option>
<option>Ipad Pro</option>
`
manu1.disabled = false
break;
case 'Vsmart':
var manu2=document.getElementById('nsx');
manu2.innerHTML=`
<option>--Select--</option>
<option>Vsmart Active 1 plus</option>
<option>Vsmart Live</option>
<option>Vsmart Joy</option>
`
manu2.disabled = false
break;
case 'Samsung':
var manu3=document.getElementById('nsx');
manu3.innerHTML=`
<option>--Select--</option>
<option>Samsung Tab</option>
<option>Samsung Galaxy</option>
<option>Samsung Note</option>
`
manu3.disabled = false
break;
}
}
function tinhtoan() {
var x=document.getElementById('fm_price').value
var y=document.getElementById('fm_quantity').value
if(isNaN(x)||isNaN(y)){
alert('Price or Quantity must be a number!!')
return
}
if(x!='' && y!=''){
document.getElementById('fm_sum').value=x*y
}
}
var bigList=[];
function addproduct() {
var getname=document.getElementById('name').value;
var getcategoryname=document.getElementById('nsx').value;
var getprice=document.getElementById('fm_price').value;
var getquantity=document.getElementById('fm_quantity').value;
var getmanu=document.getElementById('select1').value;
if(getname==''|| getcategoryname==''|| getprice==''|| getquantity==''|| getmanu==''){
alert('Please fill all forms!!');
return
}
if(isNaN(getprice)||isNaN(getquantity)){
alert('Price or Quantity must be a number!!')
return
}
if(getcategoryname=='--Select--'){
alert('Please choose your category name!!')
return
}
var smallList={
listname: getname,
listcategoryname: getcategoryname,
listprice: getprice,
listquantity: getquantity,
listmanu: getmanu
}
if(position==-1){
add(smallList)
}
else{
bigList[position]=smallList;
position=-1;
document.getElementById('btn_register').innerHTML='Add products'
display()
}
}
function display() {
var m=document.getElementById('bodytable')
m.innerHTML=''
for(i=0;i<bigList.length;i++){
var smallList=bigList[i]
m.innerHTML+=`
<tr>
<td>${i+1}</td>
<td>${smallList.listname}</td>
<td>${smallList.listcategoryname}</td>
<td>${smallList.listprice}</td>
<td>${smallList.listquantity}</td>
<td>${smallList.listmanu}</td>
<td><div onclick="Edit(${i})" style="border-radius: 3px;border: 1px solid rgb(235, 174, 84);background-color: rgb(235, 174, 84);color: white;padding: 5px;cursor: pointer;">Edit</div></td>
<td><div onclick="Delete(${i})" style="border-radius: 3px;border: 1px solid rgb(224, 85, 85);background-color: rgb(224, 85, 85);color: white;padding: 5px;cursor: pointer;">Delete</div></td>
</tr>
`
}
}
function add(smallList) {
bigList.push(smallList)
var m=document.getElementById('bodytable')
m.innerHTML+=`
<tr>
<td>${bigList.length}</td>
<td>${smallList.listname}</td>
<td>${smallList.listcategoryname}</td>
<td>${smallList.listprice}</td>
<td>${smallList.listquantity}</td>
<td>${smallList.listmanu}</td>
<td><div onclick="Edit(${bigList.length -1})" style="border-radius: 3px;border: 1px solid rgb(235, 174, 84);background-color: rgb(235, 174, 84);color: white;padding: 5px;cursor: pointer;">Edit</div></td>
<td><div onclick="Delete(${bigList.length -1})" style="border-radius: 3px;border: 1px solid rgb(224, 85, 85);background-color: rgb(224, 85, 85);color: white;padding: 5px;cursor: pointer;">Delete</div></td>
</tr>
`
}
function resetall() {
document.getElementsByTagName('form')[0].reset()
document.getElementById('nsx').value='';
document.getElementById('nsx').disabled=true;
}
var position=-1;
function Edit(index) {
position=index;
var smallList= bigList[index];
console.log(smallList)
document.getElementById('name').value=smallList.listname;
document.getElementById('fm_price').value=smallList.listprice;
document.getElementById('fm_quantity').value=smallList.listquantity;
document.getElementById('select1').value=smallList.listmanu;
changeManufacturer()
document.getElementById('btn_register').innerHTML='Update'
}
function Delete(index) {
var m=document.getElementById('bodytable')
m.innerHTML=''
bigList.splice(index,1)
for(i=0;i<bigList.length;i++){
var smallList=bigList[i]
m.innerHTML+=`
<tr>
<td>${i+1}</td>
<td>${smallList.listname}</td>
<td>${smallList.listcategoryname}</td>
<td>${smallList.listprice}</td>
<td>${smallList.listquantity}</td>
<td>${smallList.listmanu}</td>
<td><div onclick="Edit(${i})" style="border-radius: 3px;border: 1px solid rgb(235, 174, 84);background-color: rgb(235, 174, 84);color: white;padding: 5px;cursor: pointer;">Edit</div></td>
<td><div onclick="Delete(${i})" style="border-radius: 3px;border: 1px solid rgb(224, 85, 85);background-color: rgb(224, 85, 85);color: white;padding: 5px;cursor: pointer;">Delete</div></td>
</tr>
`
}
}
</script>
</head>
<body>
<div class="main">
<div class="top">
Input product's detail information
</div>
<form>
<ul>
<li>
<div style="font-weight: bold; font-family: Arial, Helvetica, sans-serif;">Product Name</div>
<input type="text" style="width: 706px;height: 22px;background-color:#f7f69e;border: 1px solid grey;" id="name">
</li>
<li>
<div style="font-weight: bold; font-family: Arial, Helvetica, sans-serif;">Category Name</div>
<select type="text" style="width: 712px;height: 29px;border: 1px solid grey;" id="nsx" disabled>
</select>
</li>
<li>
<div style="font-weight: bold; font-family: Arial, Helvetica, sans-serif;">Price</div>
<input type="text" style="width: 706px;height: 22px;border: 1px solid grey;" id="fm_price" onchange="tinhtoan()">
</li>
<li>
<div style="font-weight: bold; font-family: Arial, Helvetica, sans-serif;">Quantity</div>
<input type="text" style="width: 706px;height: 22px;border: 1px solid grey;" id="fm_quantity" onchange="tinhtoan()">
</li>
<li>
<div style="font-weight: bold; font-family: Arial, Helvetica, sans-serif;">Total Price</div>
<input type="text" style="width: 706px;height: 22px;border: 1px solid grey;background-color: rgb(241, 232, 232);" id="fm_sum" onchange="tinhtoan()" disabled>
</li>
<li>
<div style="font-weight: bold; font-family: Arial, Helvetica, sans-serif;">Manufacturer Name</div>
<select type="text" onchange="changeManufacturer()" style="width: 712px;height: 29px;border: 1px solid grey;" id="select1">
<option>--Select--</option>
<option value="Apple">Apple</option>
<option value="Vsmart">Vsmart</option>
<option value="Samsung">Samsung</option>
</select>
</li>
<div style="display: flex;">
<div id="btn_register" onclick="addproduct()" style="border: 1px solid rgb(91, 202, 118);border-radius: 3px;color: white;padding: 5px;background-color: rgb(91, 202, 118);margin-right: 8px;cursor: pointer;">Add products</div>
<div onclick="resetall()" style="border: 1px solid rgb(235, 174, 84);border-radius: 3px;color: white;padding: 5px;background-color: rgb(235, 174, 84);cursor: pointer;">Reset</div>
</div>
</ul>
</form>
</div>
<br><br><br>
<table border="1" cellspacing='0' cellpadding="5">
<caption style="background-color: #4970a3;color: white;height: 30px;padding-top: 8px;">Management Products</caption>
<thead id="headtable">
<th>No</th>
<th>Product Name</th>
<th>Category name</th>
<th>Price</th>
<th>Quantity</th>
<th>Manufacturer Name</th>
<th></th>
<th></th>
</thead>
<tbody id="bodytable">
</tbody>
</table>
</body>
</html>